在 C# 中格式化日期時間
Fil Zjazel Romaeus Villegas
2024年2月16日
Csharp
Csharp Datetime
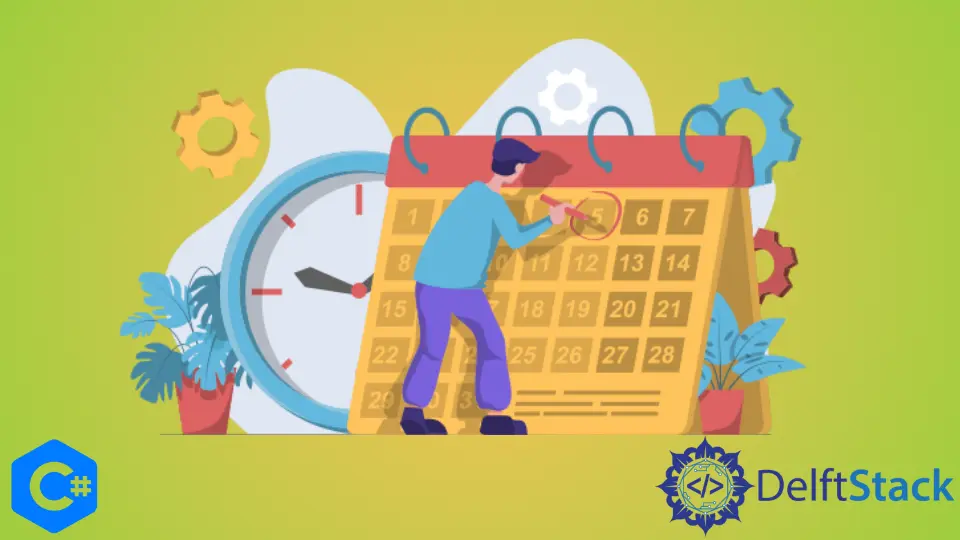
本教程將演示如何使用 ToString()
或 String.Format
函式從 DateTime
變數建立格式化字串。
日期格式說明符是 DateTime
變數中不同元件的字串表示形式。你可以在 ToString()
和 String.Format
函式中使用它們來指定你自己的自定義 DateTime
格式。
以下是一些常用的日期格式說明符。
樣品日期:08/09/2021 下午 3:25:10
自定義日期時間格式說明符:
格式說明符 | 輸出 | 日期時間部分 |
---|---|---|
d |
8 |
天 |
dd |
08 |
天 |
M |
9 |
月 |
MM |
09 |
月 |
MMM |
Sep |
月 |
MMMM |
September |
月 |
yy |
21 |
年 |
yyyy |
2021 |
年 |
hh |
03 |
小時 |
HH |
15 |
小時 |
mm |
25 |
分鐘 |
ss |
10 |
秒 |
tt |
PM |
時間 |
標準日期時間格式說明符:
說明符 | DateTimeFormatInfo 屬性 | 模式值 |
---|---|---|
t |
ShortTimePattern |
h:mm tt |
d |
ShortDatePattern |
M/d/yyyy |
F |
FullDateTimePattern |
dddd, MMMM dd, yyyy h:mm:ss tt |
M |
MonthDayPattern |
MMMM dd |
有關 DateTime
格式說明符的更詳細列表和更多示例,你可以檢視以下 Microsoft 的官方文件:
在 C# 中使用 ToString()
格式化日期時間
將 DateTime
格式化為字串就像將 ToString()
應用於 DateTime
並提供你想要使用的格式一樣簡單。
DateTime.Now.ToString("ddMMyyyy") DateTime.Now.ToString("d")
例子:
using System;
namespace ConsoleApp1 {
class Program {
static void Main(string[] args) {
// Initialize the datetime value
DateTime date = new DateTime(2021, 12, 18, 16, 5, 7, 123);
// Format the datetime value to a standard format (ShortDatePattern)
string stanadard_fmt_date = date.ToString("d");
// Format the datetime value to a custom format
string custom_fmt_date = date.ToString("ddMMyyyy");
// Print the datetime formatted in the different ways
Console.WriteLine("Plain DateTime: " + date.ToString());
Console.WriteLine("Standard DateTime Format: " + stanadard_fmt_date);
Console.WriteLine("Custom DateTime Format: " + custom_fmt_date);
}
}
}
輸出:
Plain DateTime: 18/12/2021 4:05:07 pm
Standard DateTime Format: 18/12/2021
Custom DateTime Format: 18122021
在 C# 中使用 String.Format()
格式化 DateTime
使用 String.Format
方法將 DateTime
格式化為字串是通過在引數中提供格式和 DateTime
值來完成的。列印格式時,格式必須用括號括起來並以"0:"
為字首。
String.Format("{0:ddMMyyyy}", DateTime.Now) String.Format("{0:d}", DateTime.Now)
例子:
using System;
namespace ConsoleApp1 {
class Program {
static void Main(string[] args) {
// Initialize the datetime value
DateTime date = new DateTime(2021, 12, 18, 16, 5, 7, 123);
// Format the datetime value to a standard format (ShortDatePattern)
string stanadard_fmt_date = String.Format("{0:d}", date);
// Format the datetime value to a custom format
string custom_fmt_date = String.Format("{0:ddMMyyyy}", date);
// Print the datetime formatted in the different ways
Console.WriteLine("Plain DateTime: " + date.ToString());
Console.WriteLine("Standard DateTime Format: " + stanadard_fmt_date);
Console.WriteLine("Custom DateTime Format: " + custom_fmt_date);
}
}
}
輸出:
Plain DateTime: 18/12/2021 4:05:07 pm
Standard DateTime Format: 18/12/2021
Custom DateTime Format: 18122021
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe