C#에서 날짜/시간 형식 지정
Fil Zjazel Romaeus Villegas
2024년2월16일
Csharp
Csharp Datetime
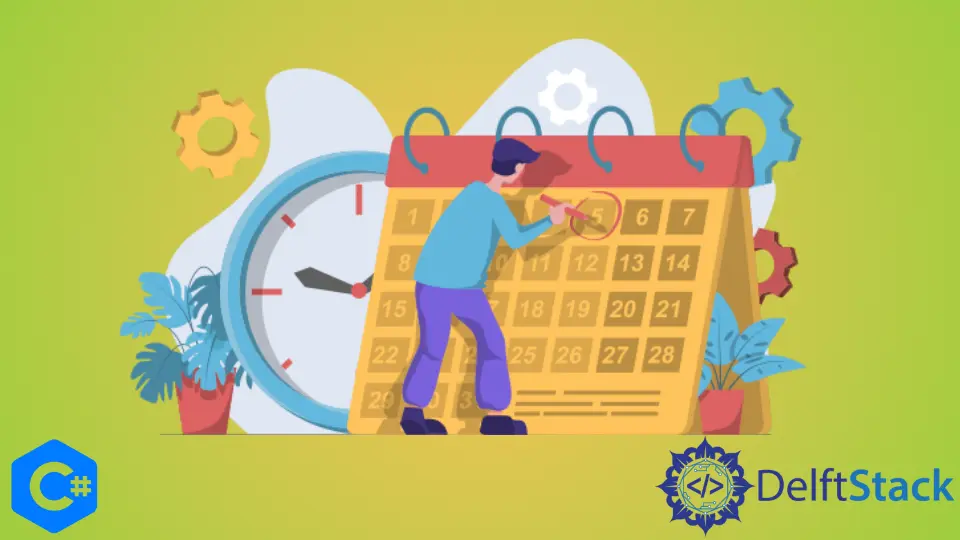
이 튜토리얼은 ToString()
또는 String.Format
함수를 사용하여 DateTime
변수에서 형식이 지정된 문자열을 만드는 방법을 보여줍니다.
날짜 형식 지정자는 DateTime
변수에 있는 다양한 구성 요소의 문자열 표현입니다. ToString()
및 String.Format
함수에서 모두 사용하여 사용자 정의 DateTime
형식을 지정할 수 있습니다.
다음은 일반적으로 사용되는 날짜 형식 지정자입니다.
샘플 날짜: 08/09/2021 3:25:10 PM
사용자 지정 날짜/시간 형식 지정자:
형식 지정자 | 산출 | 날짜/시간 부분 |
---|---|---|
d |
8 |
낮 |
dd |
08 |
낮 |
M |
9 |
월 |
MM |
09 |
월 |
MMM |
Sep |
월 |
MMMM |
September |
월 |
yy |
21 |
년도 |
yyyy |
2021 |
년도 |
hh |
03 |
시간 |
HH |
15 |
시간 |
mm |
25 |
분 |
ss |
10 |
두번째 |
tt |
PM |
팀 |
표준 날짜/시간 형식 지정자:
지정자 | DateTimeFormatInfo 속성 | 패턴 값 |
---|---|---|
t |
ShortTimePattern |
h:mm tt |
d |
ShortDatePattern |
M/d/yyyy |
F |
FullDateTimePattern |
dddd, MMMM dd, yyyy h:mm:ss tt |
M |
MonthDayPattern |
MMMM dd |
DateTime
형식 지정자에 대한 자세한 목록과 더 많은 예를 보려면 아래 Microsoft의 공식 문서를 확인할 수 있습니다.
ToString()
을 사용하여 C#에서 DateTime 형식 지정
DateTime
을 문자열로 포맷하는 것은 ToString()
을 DateTime
에 적용하고 사용하려는 포맷을 제공하는 것만큼 간단합니다.
DateTime.Now.ToString("ddMMyyyy") DateTime.Now.ToString("d")
예시:
using System;
namespace ConsoleApp1 {
class Program {
static void Main(string[] args) {
// Initialize the datetime value
DateTime date = new DateTime(2021, 12, 18, 16, 5, 7, 123);
// Format the datetime value to a standard format (ShortDatePattern)
string stanadard_fmt_date = date.ToString("d");
// Format the datetime value to a custom format
string custom_fmt_date = date.ToString("ddMMyyyy");
// Print the datetime formatted in the different ways
Console.WriteLine("Plain DateTime: " + date.ToString());
Console.WriteLine("Standard DateTime Format: " + stanadard_fmt_date);
Console.WriteLine("Custom DateTime Format: " + custom_fmt_date);
}
}
}
출력:
Plain DateTime: 18/12/2021 4:05:07 pm
Standard DateTime Format: 18/12/2021
Custom DateTime Format: 18122021
String.Format()
을 사용하여 C#에서 DateTime 형식 지정
String.Format
메서드를 사용하여 DateTime
을 문자열로 형식화하려면 매개변수에 형식과 DateTime
값을 모두 제공하면 됩니다. 형식을 인쇄할 때 형식은 대괄호로 묶어야 하며 접두어 "0:"
이 있어야 합니다.
String.Format("{0:ddMMyyyy}", DateTime.Now) String.Format("{0:d}", DateTime.Now)
예시:
using System;
namespace ConsoleApp1 {
class Program {
static void Main(string[] args) {
// Initialize the datetime value
DateTime date = new DateTime(2021, 12, 18, 16, 5, 7, 123);
// Format the datetime value to a standard format (ShortDatePattern)
string stanadard_fmt_date = String.Format("{0:d}", date);
// Format the datetime value to a custom format
string custom_fmt_date = String.Format("{0:ddMMyyyy}", date);
// Print the datetime formatted in the different ways
Console.WriteLine("Plain DateTime: " + date.ToString());
Console.WriteLine("Standard DateTime Format: " + stanadard_fmt_date);
Console.WriteLine("Custom DateTime Format: " + custom_fmt_date);
}
}
}
출력:
Plain DateTime: 18/12/2021 4:05:07 pm
Standard DateTime Format: 18/12/2021
Custom DateTime Format: 18122021
튜토리얼이 마음에 드시나요? DelftStack을 구독하세요 YouTube에서 저희가 더 많은 고품질 비디오 가이드를 제작할 수 있도록 지원해주세요. 구독하다