How to Format Datetime in C#
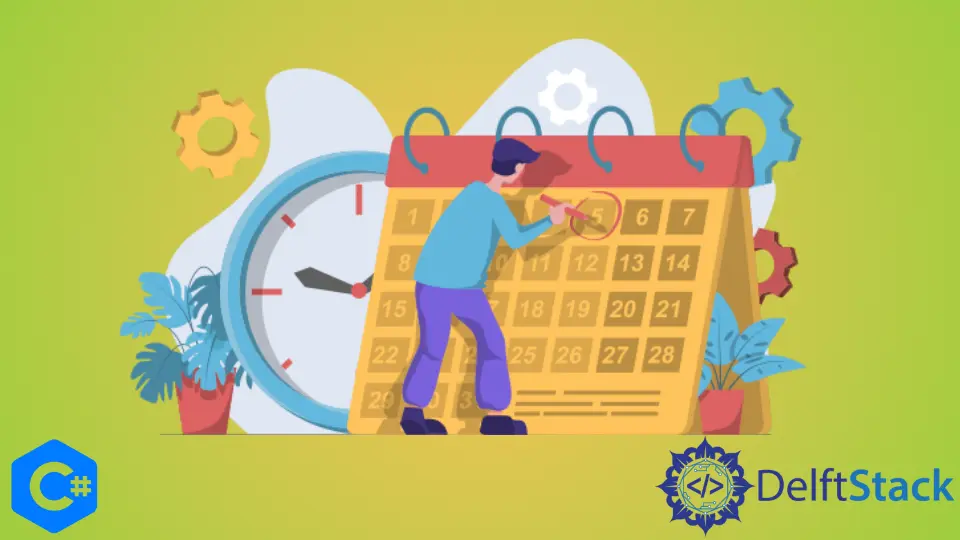
This tutorial will demonstrate how to create a formatted string from a DateTime
variable using the ToString()
or String.Format
function.
Date Format Specifiers are the string representation of the different components in a DateTime
variable. You can use them in both the ToString()
and String.Format
functions to specify your own custom DateTime
format.
Here are some commonly used date format specifiers.
Sample Date: 08/09/2021 3:25:10 PM
Custom DateTime Format Specifiers:
Format Specifier | Output | DateTime Part |
---|---|---|
d |
8 |
Day |
dd |
08 |
Day |
M |
9 |
Month |
MM |
09 |
Month |
MMM |
Sep |
Month |
MMMM |
September |
Month |
yy |
21 |
Year |
yyyy |
2021 |
Year |
hh |
03 |
Hour |
HH |
15 |
Hour |
mm |
25 |
Minute |
ss |
10 |
Second |
tt |
PM |
Tim |
Standard DateTime Format Specifiers:
Specifier | DateTimeFormatInfo Property | Pattern Value |
---|---|---|
t |
ShortTimePattern |
h:mm tt |
d |
ShortDatePattern |
M/d/yyyy |
F |
FullDateTimePattern |
dddd, MMMM dd, yyyy h:mm:ss tt |
M |
MonthDayPattern |
MMMM dd |
For a more detailed list and more examples for DateTime
Format specifiers, you can check out the official documentation from Microsoft below:
Formatting DateTime in C# Using ToString()
Formatting a DateTime
to string is as simple as applying the ToString()
to the DateTime
and supplying the format you would like to use.
DateTime.Now.ToString("ddMMyyyy") DateTime.Now.ToString("d")
Example:
using System;
namespace ConsoleApp1 {
class Program {
static void Main(string[] args) {
// Initialize the datetime value
DateTime date = new DateTime(2021, 12, 18, 16, 5, 7, 123);
// Format the datetime value to a standard format (ShortDatePattern)
string stanadard_fmt_date = date.ToString("d");
// Format the datetime value to a custom format
string custom_fmt_date = date.ToString("ddMMyyyy");
// Print the datetime formatted in the different ways
Console.WriteLine("Plain DateTime: " + date.ToString());
Console.WriteLine("Standard DateTime Format: " + stanadard_fmt_date);
Console.WriteLine("Custom DateTime Format: " + custom_fmt_date);
}
}
}
Output:
Plain DateTime: 18/12/2021 4:05:07 pm
Standard DateTime Format: 18/12/2021
Custom DateTime Format: 18122021
Formatting DateTime in C# Using String.Format()
Formatting a DateTime
to string using the String.Format
method is done by providing both the format and the DateTime
value in the parameters. The format must be enclosed in brackets and prefixed with "0:"
when printing the format.
String.Format("{0:ddMMyyyy}", DateTime.Now) String.Format("{0:d}", DateTime.Now)
Example:
using System;
namespace ConsoleApp1 {
class Program {
static void Main(string[] args) {
// Initialize the datetime value
DateTime date = new DateTime(2021, 12, 18, 16, 5, 7, 123);
// Format the datetime value to a standard format (ShortDatePattern)
string stanadard_fmt_date = String.Format("{0:d}", date);
// Format the datetime value to a custom format
string custom_fmt_date = String.Format("{0:ddMMyyyy}", date);
// Print the datetime formatted in the different ways
Console.WriteLine("Plain DateTime: " + date.ToString());
Console.WriteLine("Standard DateTime Format: " + stanadard_fmt_date);
Console.WriteLine("Custom DateTime Format: " + custom_fmt_date);
}
}
}
Output:
Plain DateTime: 18/12/2021 4:05:07 pm
Standard DateTime Format: 18/12/2021
Custom DateTime Format: 18122021