CharAt in C#
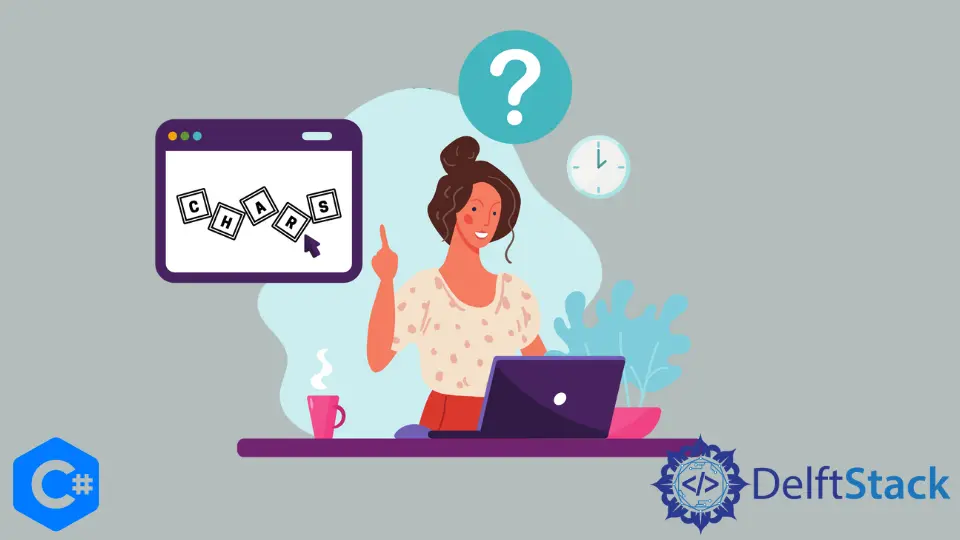
This tutorial will demonstrate how to use the equivalents of Java’s method charAt()
. charAt()
is a method that pulls an individual character from a string by its position. There are a few ways to do this in C#, which we will discuss below.
While strings are a class in C# and not an array, the string can be treated similarly to an array in this scenario. Just like how you would apply an indexer to an array to get the value at a specific position, you can pull a character from the string. This is the simplest and most efficient way to pull a single character from a string.
string sample_str = "Test";
char sample_chr = sample_str[0];
All indexes are zero-based so that the first character can be pulled by the index 0, the second by 1, etc.
Example:
using System;
namespace StringArray_Example {
class Program {
static void Main(string[] args) {
// Initialize the test string
string test_str = "Test String";
// Get the first character of the string at index 0
char charAt = test_str[0];
// Print the character pulled
Console.WriteLine("The Character at Index 0 is: " + charAt);
// Get the last character of the string
// The index is the length of the test string minus 1
charAt = test_str[test_str.Length - 1];
// Print the character pulled
Console.WriteLine("The last character of the string is: " + charAt);
Console.ReadLine();
}
}
}
In the example above, we declared a string to pull characters. Treating the string like an array and pulling the character by index, we pulled both the first character (with an index of 0) and the last character (which has the index of the length of the string minus 1).
It is important to note if you try to provide an index greater than or equal to the size of the string, you will get an Index was outside the bounds of the array
error.
Output:
The Character at Index 0 is: T
The last character of the string is: g
The Substring
Method in C#
Another method you can use is by using the Substring()
method, which pulls a string from within a string based on the location provided and the length of the string to be retrieved. This method is slower but can be easily modified to pull more than just a single character. Unlike the indexer method, this returns a string and not a char.
string sample_str = "Test";
string sample_chr = sample_str.Substring(index, length);
By default, if you leave the length blank, it will return all of the characters from the index position to the end of the string.
Example:
using System;
namespace Substring_Example {
class Program {
static void Main(string[] args) {
// Initialize the test string
string test_str = "Test String";
// Get the first character of the string at index 0
string charAt = test_str.Substring(0, 1);
// Print the character pulled
Console.WriteLine("The Character at Index 0 is: " + charAt);
// Get the last character of the string
// The index is the length of the test string minus 1
charAt = test_str.Substring(test_str.Length - 1, 1);
// Print the character pulled
Console.WriteLine("The last character of the string is: " + charAt);
Console.ReadLine();
}
}
}
In the example above, we declared a string to pull characters. Using the Substring()
method, we pulled the substring from the index of 0 and with a length of 1. After printing the result to the console, we also retrieved the last character of the string using the same method. The output for both the string indexer and substring methods is the same.
Output:
The Character at Index 0 is: T
The last character of the string is: g