C# 中的 CharAt
Fil Zjazel Romaeus Villegas
2023年10月12日
Csharp
Csharp String
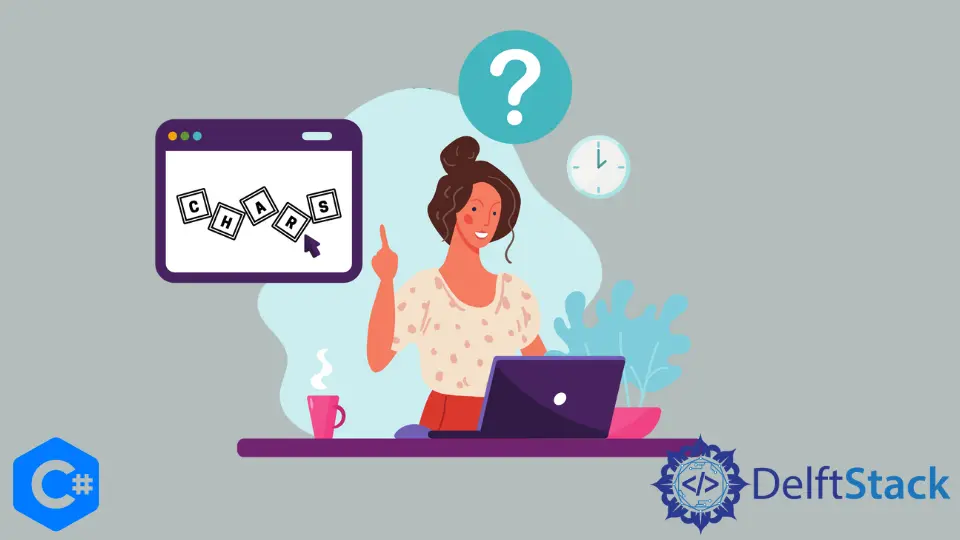
本教程将演示如何使用 Java 方法 charAt()
的等价函数。charAt()
是一种按位置从字符串中提取单个字符的方法。在 C# 中有几种方法可以做到这一点,我们将在下面讨论。
虽然字符串在 C# 中是一个类而不是数组,但在这种情况下,可以将字符串与数组类似地对待。就像你如何将索引器应用于数组以获取特定位置的值一样,你可以从字符串中提取一个字符。这是从字符串中提取单个字符的最简单、最有效的方法。
string sample_str = "Test";
char sample_chr = sample_str[0];
所有索引都是从零开始的,因此第一个字符可以被索引 0 拉出,第二个字符可以被 1 拉出,等等。
例子:
using System;
namespace StringArray_Example {
class Program {
static void Main(string[] args) {
// Initialize the test string
string test_str = "Test String";
// Get the first character of the string at index 0
char charAt = test_str[0];
// Print the character pulled
Console.WriteLine("The Character at Index 0 is: " + charAt);
// Get the last character of the string
// The index is the length of the test string minus 1
charAt = test_str[test_str.Length - 1];
// Print the character pulled
Console.WriteLine("The last character of the string is: " + charAt);
Console.ReadLine();
}
}
}
在上面的示例中,我们声明了一个字符串来提取字符。将字符串视为数组并按索引拉出字符,我们拉出第一个字符(索引为 0)和最后一个字符(字符串长度的索引减 1)。
请务必注意,如果你尝试提供大于或等于字符串大小的索引,你将收到 Index was outside the bounds of the array
错误。
输出:
The Character at Index 0 is: T
The last character of the string is: g
C#
中的 Substring
方法
你可以使用的另一种方法是使用 Substring()
方法,该方法根据提供的位置和要检索的字符串的长度从字符串中提取字符串。此方法速度较慢,但可以轻松修改为拉出多个字符。与 indexer 方法不同,它返回一个字符串而不是一个字符。
string sample_str = "Test";
string sample_chr = sample_str.Substring(index, length);
默认情况下,如果将长度留空,它将返回从索引位置到字符串末尾的所有字符。
例子:
using System;
namespace Substring_Example {
class Program {
static void Main(string[] args) {
// Initialize the test string
string test_str = "Test String";
// Get the first character of the string at index 0
string charAt = test_str.Substring(0, 1);
// Print the character pulled
Console.WriteLine("The Character at Index 0 is: " + charAt);
// Get the last character of the string
// The index is the length of the test string minus 1
charAt = test_str.Substring(test_str.Length - 1, 1);
// Print the character pulled
Console.WriteLine("The last character of the string is: " + charAt);
Console.ReadLine();
}
}
}
在上面的示例中,我们声明了一个字符串来提取字符。使用 Substring()
方法,我们从索引 0 和长度为 1 的子字符串中提取子字符串。在将结果打印到控制台后,我们还使用相同的方法检索字符串的最后一个字符。字符串索引器和子字符串方法的输出是相同的。
输出:
The Character at Index 0 is: T
The last character of the string is: g
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe