C# 中的 CharAt
Fil Zjazel Romaeus Villegas
2023年10月12日
Csharp
Csharp String
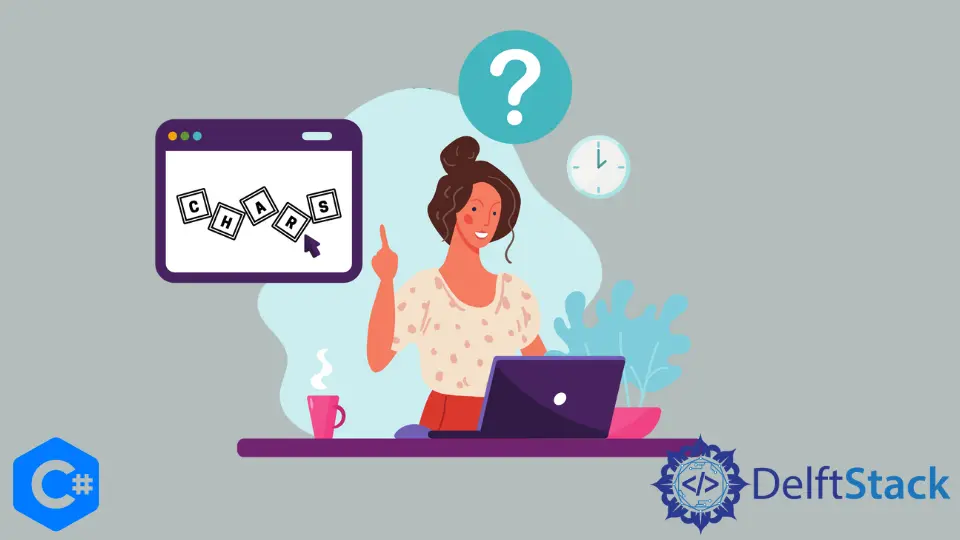
本教程將演示如何使用 Java 方法 charAt()
的等價函式。charAt()
是一種按位置從字串中提取單個字元的方法。在 C# 中有幾種方法可以做到這一點,我們將在下面討論。
雖然字串在 C# 中是一個類而不是陣列,但在這種情況下,可以將字串與陣列類似地對待。就像你如何將索引器應用於陣列以獲取特定位置的值一樣,你可以從字串中提取一個字元。這是從字串中提取單個字元的最簡單、最有效的方法。
string sample_str = "Test";
char sample_chr = sample_str[0];
所有索引都是從零開始的,因此第一個字元可以被索引 0 拉出,第二個字元可以被 1 拉出,等等。
例子:
using System;
namespace StringArray_Example {
class Program {
static void Main(string[] args) {
// Initialize the test string
string test_str = "Test String";
// Get the first character of the string at index 0
char charAt = test_str[0];
// Print the character pulled
Console.WriteLine("The Character at Index 0 is: " + charAt);
// Get the last character of the string
// The index is the length of the test string minus 1
charAt = test_str[test_str.Length - 1];
// Print the character pulled
Console.WriteLine("The last character of the string is: " + charAt);
Console.ReadLine();
}
}
}
在上面的示例中,我們宣告瞭一個字串來提取字元。將字串視為陣列並按索引拉出字元,我們拉出第一個字元(索引為 0)和最後一個字元(字串長度的索引減 1)。
請務必注意,如果你嘗試提供大於或等於字串大小的索引,你將收到 Index was outside the bounds of the array
錯誤。
輸出:
The Character at Index 0 is: T
The last character of the string is: g
C#
中的 Substring
方法
你可以使用的另一種方法是使用 Substring()
方法,該方法根據提供的位置和要檢索的字串的長度從字串中提取字串。此方法速度較慢,但可以輕鬆修改為拉出多個字元。與 indexer 方法不同,它返回一個字串而不是一個字元。
string sample_str = "Test";
string sample_chr = sample_str.Substring(index, length);
預設情況下,如果將長度留空,它將返回從索引位置到字串末尾的所有字元。
例子:
using System;
namespace Substring_Example {
class Program {
static void Main(string[] args) {
// Initialize the test string
string test_str = "Test String";
// Get the first character of the string at index 0
string charAt = test_str.Substring(0, 1);
// Print the character pulled
Console.WriteLine("The Character at Index 0 is: " + charAt);
// Get the last character of the string
// The index is the length of the test string minus 1
charAt = test_str.Substring(test_str.Length - 1, 1);
// Print the character pulled
Console.WriteLine("The last character of the string is: " + charAt);
Console.ReadLine();
}
}
}
在上面的示例中,我們宣告瞭一個字串來提取字元。使用 Substring()
方法,我們從索引 0 和長度為 1 的子字串中提取子字串。在將結果列印到控制檯後,我們還使用相同的方法檢索字串的最後一個字元。字串索引器和子字串方法的輸出是相同的。
輸出:
The Character at Index 0 is: T
The last character of the string is: g
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe