C#의 CharAt
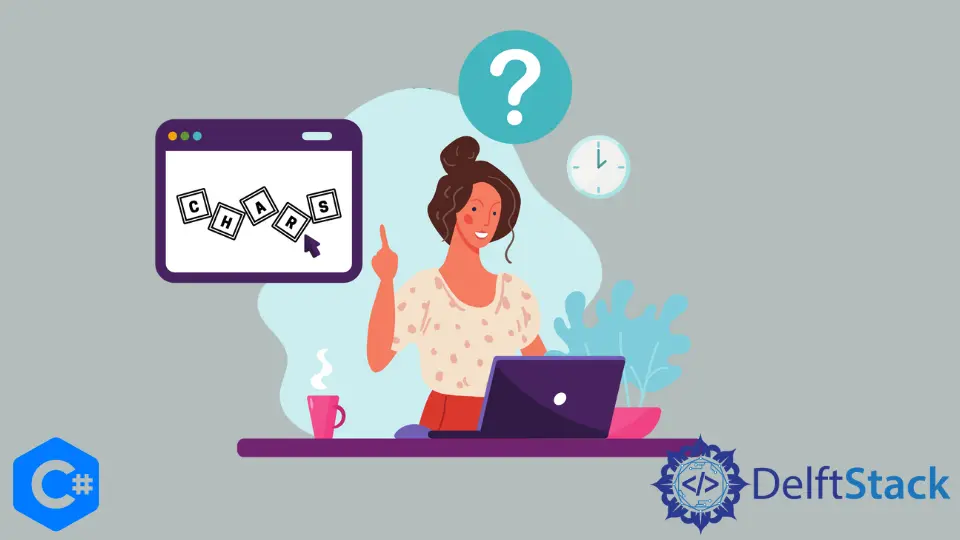
이 튜토리얼은 Java의 charAt()
메소드와 동등한 것을 사용하는 방법을 보여줄 것입니다. charAt()
는 위치별로 문자열에서 개별 문자를 가져오는 메서드입니다. 아래에서 논의할 C#에서 이를 수행하는 몇 가지 방법이 있습니다.
문자열은 배열이 아니라 C#의 클래스이지만 이 시나리오에서는 문자열을 배열과 유사하게 처리할 수 있습니다. 특정 위치에서 값을 가져오기 위해 배열에 인덱서를 적용하는 것과 마찬가지로 문자열에서 문자를 가져올 수 있습니다. 이것은 문자열에서 단일 문자를 가져오는 가장 간단하고 효율적인 방법입니다.
string sample_str = "Test";
char sample_chr = sample_str[0];
모든 인덱스는 0부터 시작하므로 첫 번째 문자는 인덱스 0으로, 두 번째 문자는 1로 끌어올 수 있습니다.
예시:
using System;
namespace StringArray_Example {
class Program {
static void Main(string[] args) {
// Initialize the test string
string test_str = "Test String";
// Get the first character of the string at index 0
char charAt = test_str[0];
// Print the character pulled
Console.WriteLine("The Character at Index 0 is: " + charAt);
// Get the last character of the string
// The index is the length of the test string minus 1
charAt = test_str[test_str.Length - 1];
// Print the character pulled
Console.WriteLine("The last character of the string is: " + charAt);
Console.ReadLine();
}
}
}
위의 예에서 우리는 문자를 가져오기 위해 문자열을 선언했습니다. 문자열을 배열처럼 취급하고 인덱스별로 문자를 가져오기 위해 첫 번째 문자(인덱스 0)와 마지막 문자(문자열 길이에서 1을 뺀 값)를 모두 가져왔습니다.
문자열 크기보다 크거나 같은 인덱스를 제공하려고 하면 인덱스가 배열 범위를 벗어났습니다.
오류가 발생한다는 점에 유의하는 것이 중요합니다.
출력:
The Character at Index 0 is: T
The last character of the string is: g
C#
의 Substring
메서드
사용할 수 있는 또 다른 방법은 제공된 위치와 검색할 문자열의 길이를 기반으로 문자열 내에서 문자열을 가져오는 Substring()
메서드를 사용하는 것입니다. 이 방법은 느리지만 단일 문자 이상을 가져오도록 쉽게 수정할 수 있습니다. 인덱서 메서드와 달리 이것은 char가 아닌 문자열을 반환합니다.
string sample_str = "Test";
string sample_chr = sample_str.Substring(index, length);
기본적으로 길이를 비워두면 인덱스 위치부터 문자열 끝까지의 모든 문자가 반환됩니다.
예시:
using System;
namespace Substring_Example {
class Program {
static void Main(string[] args) {
// Initialize the test string
string test_str = "Test String";
// Get the first character of the string at index 0
string charAt = test_str.Substring(0, 1);
// Print the character pulled
Console.WriteLine("The Character at Index 0 is: " + charAt);
// Get the last character of the string
// The index is the length of the test string minus 1
charAt = test_str.Substring(test_str.Length - 1, 1);
// Print the character pulled
Console.WriteLine("The last character of the string is: " + charAt);
Console.ReadLine();
}
}
}
위의 예에서 우리는 문자를 가져오기 위해 문자열을 선언했습니다. Substring()
메서드를 사용하여 인덱스가 0이고 길이가 1인 부분 문자열을 가져왔습니다. 결과를 콘솔에 인쇄한 후 동일한 메서드를 사용하여 문자열의 마지막 문자도 검색했습니다. 문자열 인덱서 및 하위 문자열 메서드의 출력은 동일합니다.
출력:
The Character at Index 0 is: T
The last character of the string is: g