C# の CharAt
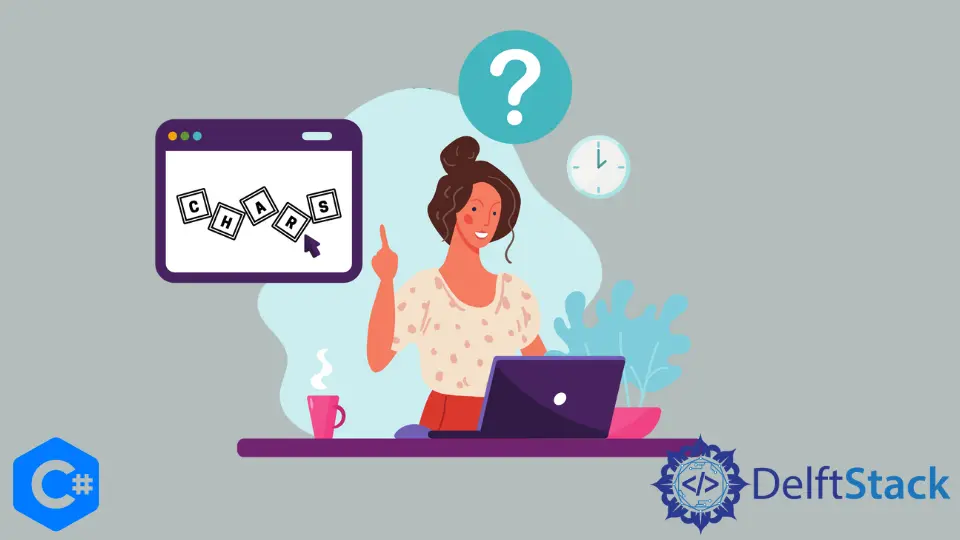
このチュートリアルでは、Java のメソッド charAt()
に相当するものを使用する方法を示します。charAt()
は、文字列から個々の文字をその位置でプルするメソッドです。C# でこれを行うには、いくつかの方法があります。これについては、以下で説明します。
C# では文字列はクラスであり、配列ではありませんが、このシナリオでは、文字列を配列と同様に扱うことができます。インデクサーを配列に適用して特定の位置の値を取得するのと同じように、文字列から文字を引き出すことができます。これは、文字列から 1 文字を引き出す最も簡単で効率的な方法です。
string sample_str = "Test";
char sample_chr = sample_str[0];
すべてのインデックスはゼロベースであるため、最初の文字はインデックス 0 でプルされ、2 番目の文字は 1 でプルされます。
例:
using System;
namespace StringArray_Example {
class Program {
static void Main(string[] args) {
// Initialize the test string
string test_str = "Test String";
// Get the first character of the string at index 0
char charAt = test_str[0];
// Print the character pulled
Console.WriteLine("The Character at Index 0 is: " + charAt);
// Get the last character of the string
// The index is the length of the test string minus 1
charAt = test_str[test_str.Length - 1];
// Print the character pulled
Console.WriteLine("The last character of the string is: " + charAt);
Console.ReadLine();
}
}
}
上記の例では、文字をプルするための文字列を宣言しました。文字列を配列のように扱い、インデックスで文字をプルすることで、最初の文字(インデックスが 0)と最後の文字(文字列の長さから 1 を引いたインデックス)の両方をプルしました。
文字列のサイズ以上のインデックスを指定しようとすると、インデックスが配列の境界外にありました
というエラーが発生することに注意してください。
出力:
The Character at Index 0 is: T
The last character of the string is: g
C# の Substring
メソッド
使用できるもう 1つのメソッドは、Substring()
メソッドを使用することです。このメソッドは、指定された場所と取得する文字列の長さに基づいて、文字列内から文字列をプルします。この方法は低速ですが、1 文字以上をプルするように簡単に変更できます。インデクサーメソッドとは異なり、これは文字ではなく文字列を返します。
string sample_str = "Test";
string sample_chr = sample_str.Substring(index, length);
デフォルトでは、長さを空白のままにすると、インデックス位置から文字列の最後までのすべての文字が返されます。
例:
using System;
namespace Substring_Example {
class Program {
static void Main(string[] args) {
// Initialize the test string
string test_str = "Test String";
// Get the first character of the string at index 0
string charAt = test_str.Substring(0, 1);
// Print the character pulled
Console.WriteLine("The Character at Index 0 is: " + charAt);
// Get the last character of the string
// The index is the length of the test string minus 1
charAt = test_str.Substring(test_str.Length - 1, 1);
// Print the character pulled
Console.WriteLine("The last character of the string is: " + charAt);
Console.ReadLine();
}
}
}
上記の例では、文字をプルするための文字列を宣言しました。Substring()
メソッドを使用して、インデックス 0 から長さ 1 のサブストリングをプルしました。結果をコンソールに出力した後、同じメソッドを使用してストリングの最後の文字も取得しました。文字列インデクサーとサブ文字列メソッドの両方の出力は同じです。
出力:
The Character at Index 0 is: T
The last character of the string is: g