How to Pass 2D Array to a Function in C++
- Method 1: Using Pointers
- Method 2: Using References
- Method 3: Using std::vector
- Method 4: Using Template Functions
- Conclusion
- FAQ
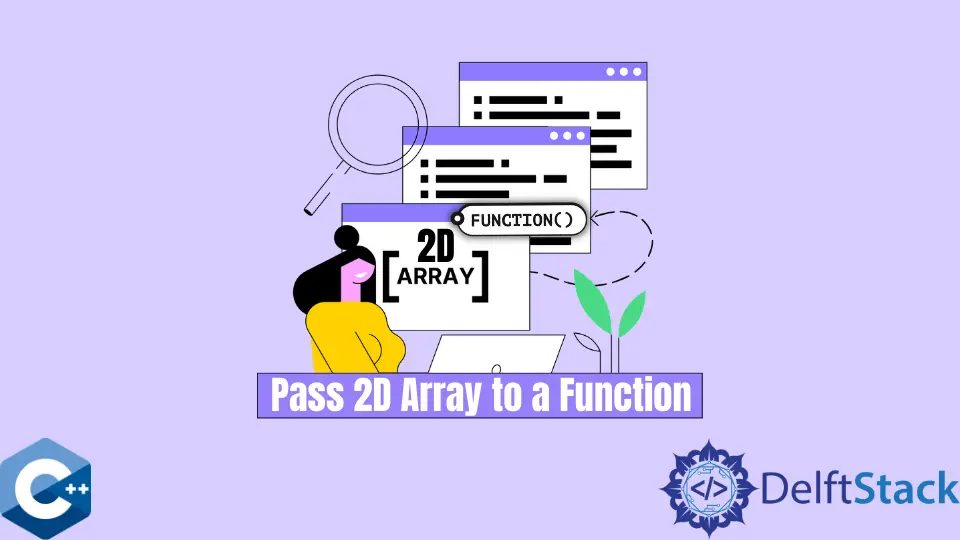
When working with C++, passing 2D arrays to functions can be a bit tricky. However, understanding the different methods available can simplify this process significantly.
In this article, we’ll explore multiple ways to pass a 2D array as a function parameter in C++. Whether you are a beginner or an experienced programmer, these techniques will enhance your coding skills and improve your understanding of array manipulation in C++. From using pointers to specifying dimensions, we’ll cover it all. By the end of this article, you’ll be equipped with the knowledge to efficiently pass 2D arrays to functions in your C++ programs. Let’s dive in!
Method 1: Using Pointers
One of the most common methods to pass a 2D array to a function in C++ is through pointers. By passing a pointer to the first element of the array, you can access the entire array within the function. Here’s how it works:
#include <iostream>
using namespace std;
void printArray(int (*arr)[3], int rows) {
for (int i = 0; i < rows; i++) {
for (int j = 0; j < 3; j++) {
cout << arr[i][j] << " ";
}
cout << endl;
}
}
int main() {
int arr[2][3] = {{1, 2, 3}, {4, 5, 6}};
printArray(arr, 2);
return 0;
}
Output:
1 2 3
4 5 6
In this example, the function printArray
takes a pointer to an array of 3 integers as its parameter. When calling the function, we pass the 2D array arr
and the number of rows. Inside the function, we can access each element using the typical array indexing method. This approach is efficient and straightforward, making it a popular choice among C++ developers.
Method 2: Using References
Another effective method to pass a 2D array to a function is by using references. This approach avoids the overhead of pointer dereferencing while still providing access to the entire array. Here’s how to implement it:
#include <iostream>
using namespace std;
void printArray(int (&arr)[2][3]) {
for (int i = 0; i < 2; i++) {
for (int j = 0; j < 3; j++) {
cout << arr[i][j] << " ";
}
cout << endl;
}
}
int main() {
int arr[2][3] = {{1, 2, 3}, {4, 5, 6}};
printArray(arr);
return 0;
}
Output:
1 2 3
4 5 6
In this case, the function printArray
takes a reference to a 2D array of fixed dimensions. This means that the dimensions must be specified at compile time, which can be beneficial for performance. The array can be accessed in the same way as in the previous example. Using references can lead to cleaner code and eliminates the need for pointer syntax, making it easier to read and maintain.
Method 3: Using std::vector
For a more flexible approach, especially when dimensions are not known at compile time, you can use std::vector
. This method allows for dynamic sizing of arrays and is often preferred in modern C++ programming. Here’s how to do it:
#include <iostream>
#include <vector>
using namespace std;
void printArray(const vector<vector<int>>& arr) {
for (const auto& row : arr) {
for (int val : row) {
cout << val << " ";
}
cout << endl;
}
}
int main() {
vector<vector<int>> arr = {{1, 2, 3}, {4, 5, 6}};
printArray(arr);
return 0;
}
Output:
1 2 3
4 5 6
In this example, we use std::vector
to create a 2D array. The printArray
function accepts a constant reference to a vector of vectors. This method is highly flexible, allowing you to create arrays of varying sizes at runtime. The use of range-based for loops also simplifies iteration through the elements, making the code cleaner and more readable.
Method 4: Using Template Functions
If you want to create a function that can handle 2D arrays of different sizes, template functions are the way to go. This method allows you to write generic code that can work with any dimensions. Here’s an example:
#include <iostream>
using namespace std;
template<size_t rows, size_t cols>
void printArray(int (&arr)[rows][cols]) {
for (size_t i = 0; i < rows; i++) {
for (size_t j = 0; j < cols; j++) {
cout << arr[i][j] << " ";
}
cout << endl;
}
}
int main() {
int arr[3][4] = {{1, 2, 3, 4}, {5, 6, 7, 8}, {9, 10, 11, 12}};
printArray(arr);
return 0;
}
Output:
1 2 3 4
5 6 7 8
9 10 11 12
With this method, the function printArray
is defined as a template that takes an array reference as a parameter. The template parameters rows
and cols
automatically adjust based on the dimensions of the array passed to the function. This approach is powerful as it provides the flexibility of handling various array sizes while maintaining type safety.
Conclusion
Passing 2D arrays to functions in C++ can be accomplished through various methods, each with its own advantages and use cases. Whether you choose to use pointers, references, std::vector
, or template functions, understanding these techniques will enhance your programming skills and allow for more efficient code. By mastering these methods, you can handle complex data structures with ease and flexibility. Remember to choose the method that best fits your needs, and happy coding!
FAQ
-
What is the simplest way to pass a 2D array in C++?
Using pointers is often considered the simplest method to pass a 2D array to a function. -
Can I pass a 2D array of different sizes to a function?
Yes, usingstd::vector
or template functions allows you to handle 2D arrays of varying sizes. -
Are there performance differences between these methods?
Yes, using references orstd::vector
can be more efficient than pointers in some cases, especially with modern C++ optimizations. -
Can I modify the 2D array inside the function?
Yes, unless you pass it as a constant reference, you can modify the elements of the array within the function. -
What is the advantage of using
std::vector
over traditional arrays?
std::vector
provides dynamic sizing, automatic memory management, and easier iteration, making it more flexible and safer to use.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook