Associative Arrays in C++
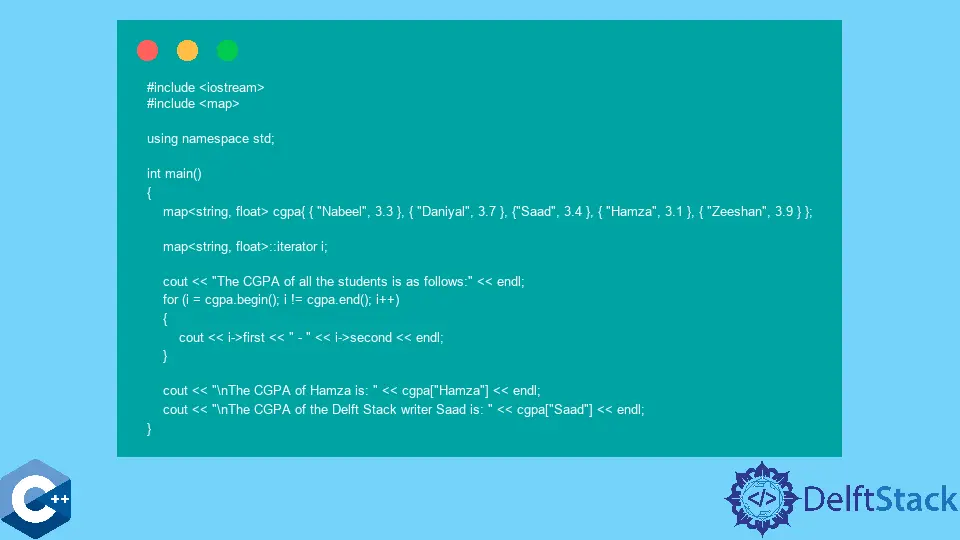
In this article, we shall understand the associative arrays and their implementation in C++ programming language.
An Overview of Arrays in C++
An array is a collection of objects that include a set of variables saved under the same name. All the items are of the same data type; the type can be a list, integer, or string.
You define an array variable, such as numbers, and then use the indexes numbers[0], numbers[1],
and so on up to numbers[99]
to represent individual variables in place of defining each variable individually, such as number0, number1,..., and number99
. A particular one of an array’s elements may be reached by using an index.
There are two array types: indexed and associative arrays. Both indexed and associative arrays have one-of-a-kind keys that can only be used once.
An array with a numeric key is called an indexed array. In its most basic form, it takes the form of an array, with each array key being connected to its own value.
Associative Arrays in C++
In this section, we will concentrate on associative arrays in C++.
A unique kind of array known as an associative array is one in which the value of the index may be of any data type. The index may be any of the following: an integer, character, floating point value, string, etc.
However, the index values must be completely unique to gain access to the data items included in the array. The name given to the index in an associative array is called the key, and the data stored at that particular place is called the value.
As a result, we can conceptualize the associative array as a key-value pair. Therefore, the components of an associative array are pairs consisting of a key and a value.
Implementation of the Associative Arrays in C++
In the programming language C++, associative arrays may also be referred to as maps. Let’s see an example to illustrate how to construct the map in C++ such that it returns a key against the parsed value.
First, we need to import the libraries so that our application can use the map
and the other methods.
#include <iostream>
#include <map>
Create a map cgpa
with the string type as the key and the type float as the value within your program’s main()
method. Fill the map with some string and float values in the appropriate spaces.
map<string, float> cgpa{{"Nabeel", 3.3},
{"Daniyal", 3.7},
{"Saad", 3.4},
{"Hamza", 3.1},
{"Zeeshan", 3.9}};
Create an iterator for the map.
map<string, float>::iterator i;
Show a message that lists all of the values included in the map. Build a for
loop that displays all the values as it iterates across the map from beginning to end.
cout << "The CGPA of all the students is as follows:" << endl;
for (i = cgpa.begin(); i != cgpa.end(); i++) {
cout << i->first << " - " << i->second << endl;
}
Now, let’s get some values from the map based on their keys.
cout << "\nThe CGPA of Hamza is: " << cgpa["Hamza"] << endl;
cout << "\nThe CGPA of the Delft Stack writer Saad is: " << cgpa["Saad"]
<< endl;
Complete Source Code:
#include <iostream>
#include <map>
using namespace std;
int main() {
map<string, float> cgpa{{"Nabeel", 3.3},
{"Daniyal", 3.7},
{"Saad", 3.4},
{"Hamza", 3.1},
{"Zeeshan", 3.9}};
map<string, float>::iterator i;
cout << "The CGPA of all the students is as follows:" << endl;
for (i = cgpa.begin(); i != cgpa.end(); i++) {
cout << i->first << " - " << i->second << endl;
}
cout << "\nThe CGPA of Hamza is: " << cgpa["Hamza"] << endl;
cout << "\nThe CGPA of the Delft Stack writer Saad is: " << cgpa["Saad"]
<< endl;
}
Output:
The CGPA of all the students is as follows:
Daniyal - 3.7
Hamza - 3.1
Nabeel - 3.3
Saad - 3.4
Zeeshan - 3.9
The CGPA of Hamza is: 3.1
The CGPA of the Delft Stack writer Saad is: 3.4
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn