How to Represent the Deck of Cards in C++ Array
- Importance of Representing a Deck of Cards in C++ Arrays
- Using a One-Dimensional Array to Represent a Deck of Cards in C++
- Using a Two-Dimensional Array to Represent a Deck of Cards in C++
- Using a Single Array With Enumerated Values to Represent a Deck of Cards in C++
- Using a Custom Class to Represent a Deck of Cards in C++
- Conclusion
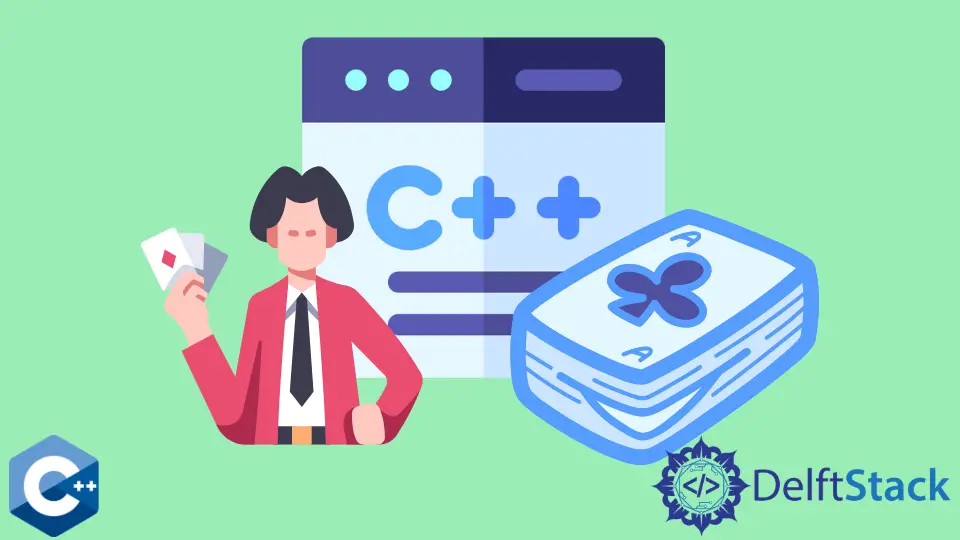
This tutorial will present a representation of a deck of cards through C++ arrays.
This article explores the significance of representing a deck of cards in C++ using arrays, discussing various methods to achieve efficient organization and manipulation of card-based data.
Importance of Representing a Deck of Cards in C++ Arrays
Representing a deck of cards in C++ arrays is important because it provides a structured and efficient way to organize and manipulate the cards within a program. Arrays allow us to store the cards in sequential order, making it easy to access individual cards, shuffle the deck, deal cards to players, and perform other operations typical in card games or simulations.
Additionally, using arrays simplifies the implementation of algorithms and logic related to card manipulation, leading to cleaner and more maintainable code. Overall, representing a deck of cards in C++ arrays streamlines programming tasks and enhances the readability and functionality of card-based applications.
Using a One-Dimensional Array to Represent a Deck of Cards in C++
Using a one-dimensional array to represent a deck of cards in C++ is important because it simplifies the storage and access of individual cards. With a one-dimensional array, each card can be stored as a single element, allowing for straightforward indexing and manipulation.
This approach reduces complexity in code, making it easier to implement common operations such as shuffling, dealing, and checking for specific cards. Additionally, using a one-dimensional array conserves memory and improves efficiency compared to multi-dimensional arrays, ensuring optimal performance in card-based applications.
Overall, leveraging a one-dimensional array enhances the simplicity and effectiveness of representing a deck of cards in C++ programming.
Below is a complete C++ code example demonstrating how to represent a deck of cards using a one-dimensional array.
#include <iostream>
enum Suit { CLUBS, DIAMONDS, HEARTS, SPADES };
enum Rank {
ACE,
TWO,
THREE,
FOUR,
FIVE,
SIX,
SEVEN,
EIGHT,
NINE,
TEN,
JACK,
QUEEN,
KING
};
struct Card {
Suit suit;
Rank rank;
};
const int DECK_SIZE = 52;
Card deck[DECK_SIZE];
void initializeDeck() {
int index = 0;
for (int s = CLUBS; s <= SPADES; ++s) {
for (int r = ACE; r <= KING; ++r) {
deck[index].suit = static_cast<Suit>(s);
deck[index].rank = static_cast<Rank>(r);
++index;
}
}
}
void displayDeck() {
for (int i = 0; i < DECK_SIZE; ++i) {
std::cout << "Card " << (i + 1) << ": ";
switch (deck[i].rank) {
case ACE:
std::cout << "Ace";
break;
case JACK:
std::cout << "Jack";
break;
case QUEEN:
std::cout << "Queen";
break;
case KING:
std::cout << "King";
break;
default:
std::cout << (deck[i].rank + 1);
break;
}
std::cout << " of ";
switch (deck[i].suit) {
case CLUBS:
std::cout << "Clubs";
break;
case DIAMONDS:
std::cout << "Diamonds";
break;
case HEARTS:
std::cout << "Hearts";
break;
case SPADES:
std::cout << "Spades";
break;
}
std::cout << std::endl;
}
}
int main() {
initializeDeck();
displayDeck();
return 0;
}
The code follows a straightforward approach to representing a deck of cards using a one-dimensional array in C++. It begins by defining two enumerations for the possible suits and ranks of a card.
Then, a struct
named Card
is created to hold the suit and rank of each card. The constant DECK_SIZE
is declared to specify the size of the deck, which is set to 52
.
The initializeDeck()
function is responsible for populating the deck
array with all 52 cards. It achieves this by iterating over each combination of suits and ranks and assigning them to the corresponding elements of the deck
array.
The displayDeck()
function is implemented to print out the contents of the deck
array in a human-readable format, showing each card’s rank and suit. Finally, in the main()
function, the deck is initialized using initializeDeck()
and then displayed using displayDeck()
.
This approach provides a simple and concise way to represent and display a deck of cards using a one-dimensional array in C++.
When you run this code, you’ll see the output displaying all 52 cards of the deck, each in the format Rank of Suit
.
This output demonstrates the effective representation of a deck of cards using a one-dimensional array in C++.
Using a Two-Dimensional Array to Represent a Deck of Cards in C++
Using a two-dimensional array to represent a deck of cards in C++ is important because it allows for a structured organization of cards based on both their suits and ranks.
With a two-dimensional array, each row can represent a suit, and each column can represent a rank.
We can also take two parallel arrays. One array to store card type, and the other array to store value at the corresponding position.
This organization simplifies access to specific cards and facilitates operations such as shuffling, dealing, and checking for card combinations. Additionally, a two-dimensional array provides a visual representation of the deck, making it easier to understand and manipulate.
Overall, leveraging a two-dimensional array enhances the efficiency and clarity of representing a deck of cards in C++ programming.
Below is a complete C++ code example demonstrating how to represent a deck of cards using a two-dimensional array.
#include <iostream>
enum Suit { CLUBS, DIAMONDS, HEARTS, SPADES };
enum Rank {
ACE,
TWO,
THREE,
FOUR,
FIVE,
SIX,
SEVEN,
EIGHT,
NINE,
TEN,
JACK,
QUEEN,
KING
};
const int NUM_SUITS = 4;
const int NUM_RANKS = 13;
int deck[NUM_SUITS][NUM_RANKS];
void initializeDeck() {
for (int suit = 0; suit < NUM_SUITS; ++suit) {
for (int rank = 0; rank < NUM_RANKS; ++rank) {
deck[suit][rank] = (suit * NUM_RANKS) + rank;
}
}
}
void displayDeck() {
for (int suit = 0; suit < NUM_SUITS; ++suit) {
for (int rank = 0; rank < NUM_RANKS; ++rank) {
std::cout << "Card " << deck[suit][rank] + 1 << ": ";
switch (rank) {
case ACE:
std::cout << "Ace";
break;
case JACK:
std::cout << "Jack";
break;
case QUEEN:
std::cout << "Queen";
break;
case KING:
std::cout << "King";
break;
default:
std::cout << (rank + 1);
break;
}
std::cout << " of ";
switch (suit) {
case CLUBS:
std::cout << "Clubs";
break;
case DIAMONDS:
std::cout << "Diamonds";
break;
case HEARTS:
std::cout << "Hearts";
break;
case SPADES:
std::cout << "Spades";
break;
}
std::cout << std::endl;
}
}
}
int main() {
initializeDeck();
displayDeck();
return 0;
}
The code demonstrates the use of a two-dimensional array to represent a deck of cards in C++. It starts by defining enumerations for the possible suits and ranks of a card.
Then, constants are declared to specify the dimensions of the two-dimensional array deck
, where each row represents a suit, and each column represents a rank. The initializeDeck()
function fills the deck
array with unique values, iterating over each suit and rank combination to assign a value to each card.
Subsequently, the displayDeck()
function prints out the rank and suit of each card in a human-readable format by iterating through the deck
array. Finally, in the main()
function, initializeDeck()
is called to populate the deck, followed by displayDeck()
to show its contents.
This approach provides a clear and structured method for representing and displaying a deck of cards using a two-dimensional array in C++.
Upon running the code, you’ll see the output displaying all 52 cards of the deck, each in the format Rank of Suit
.
This output showcases the effective representation of a deck of cards using a two-dimensional array in C++.
Utilizing a two-dimensional array to represent a deck of cards in C++ offers a structured and intuitive approach, with each row representing a suit and each column representing a rank. This method simplifies operations such as shuffling, dealing, and checking for specific cards.
Understanding this concept is pivotal for developing various card-based applications or games in C++.
Using a Single Array With Enumerated Values to Represent a Deck of Cards in C++
Using a single array with enumerated values to represent a deck of cards in C++ is important because it provides a compact and efficient way to organize and access the cards. With this approach, each card in the deck is assigned a unique identifier using enumerated values, simplifying card manipulation operations such as shuffling, dealing, and checking for specific cards.
Additionally, using a single array saves memory compared to multi-dimensional arrays, leading to better performance in memory-constrained environments. Overall, leveraging a single array with enumerated values enhances the simplicity and effectiveness of representing a deck of cards in C++ programming.
Below is a complete C++ code example demonstrating how to represent a deck of cards using a single array with enumerated values.
#include <iostream>
enum Suit { CLUBS, DIAMONDS, HEARTS, SPADES };
enum Rank {
ACE,
TWO,
THREE,
FOUR,
FIVE,
SIX,
SEVEN,
EIGHT,
NINE,
TEN,
JACK,
QUEEN,
KING
};
const int DECK_SIZE = 52;
int deck[DECK_SIZE];
void initializeDeck() {
for (int i = 0; i < DECK_SIZE; ++i) {
deck[i] = i;
}
}
void displayDeck() {
for (int i = 0; i < DECK_SIZE; ++i) {
std::cout << "Card " << (i + 1) << ": ";
Suit suit = static_cast<Suit>(deck[i] / 13);
Rank rank = static_cast<Rank>(deck[i] % 13);
switch (rank) {
case ACE:
std::cout << "Ace";
break;
case JACK:
std::cout << "Jack";
break;
case QUEEN:
std::cout << "Queen";
break;
case KING:
std::cout << "King";
break;
default:
std::cout << (rank + 1);
break;
}
std::cout << " of ";
switch (suit) {
case CLUBS:
std::cout << "Clubs";
break;
case DIAMONDS:
std::cout << "Diamonds";
break;
case HEARTS:
std::cout << "Hearts";
break;
case SPADES:
std::cout << "Spades";
break;
}
std::cout << std::endl;
}
}
int main() {
initializeDeck();
displayDeck();
return 0;
}
The code demonstrates a simple and concise method for representing a deck of cards in C++ using a single array with enumerated values. Initially, two enumerations, Suit
and Rank
, are defined to represent the possible suits and ranks of a card.
A constant, DECK_SIZE
, is then declared to specify the size of the deck
, set to 52
. The initializeDeck()
function fills the deck
array with enumerated values, assigning each card a unique identifier based on its position in the deck
.
Subsequently, the displayDeck()
function traverses the deck
array, printing out each card’s rank and suit in a human-readable format using the enumerated values. Lastly, in the main()
function, initializeDeck()
is invoked to populate the deck
, followed by calling displayDeck()
to showcase its contents.
This approach provides a straightforward and efficient way to represent and display a deck of cards in C++ using a single array with enumerated values.
Upon running the code, you’ll see the output displaying all 52 cards of the deck, each in the format Rank of Suit
.
This output demonstrates the effective representation of a deck of cards using a single array with enumerated values in C++.
Utilizing a single array with enumerated values to represent a deck of cards in C++ offers a concise and structured approach. Enumerated values simplify the assignment of unique identifiers to each card, facilitating easy access and manipulation.
Understanding this concept is essential for developing card-based applications or games in C++.
Using a Custom Class to Represent a Deck of Cards in C++
Using a custom class to represent a deck of cards in C++ is important because it encapsulates the properties and behaviors of the deck within a single entity, promoting code organization, reusability, and maintainability. By defining a Deck
class, we can abstract away the complexities of managing individual cards and instead focus on high-level deck operations such as shuffling, dealing, and checking for specific cards or combinations.
This encapsulation helps to modularize the code, making it easier to understand and modify. Additionally, a custom class allows us to define custom methods and operators tailored to deck manipulation, further enhancing the clarity and functionality of the code.
Overall, using a custom class enhances the structure and efficiency of representing a deck of cards in C++ programming, making it an essential approach for developing card-based applications or games.
Below is a complete C++ code example demonstrating how to represent a deck of cards using a custom class.
#include <algorithm>
#include <iostream>
#include <random>
#include <vector>
enum class Suit { CLUBS, DIAMONDS, HEARTS, SPADES };
enum class Rank {
ACE,
TWO,
THREE,
FOUR,
FIVE,
SIX,
SEVEN,
EIGHT,
NINE,
TEN,
JACK,
QUEEN,
KING
};
class Card {
public:
Card(Suit s, Rank r) : suit(s), rank(r) {}
Suit getSuit() const { return suit; }
Rank getRank() const { return rank; }
void display() const {
switch (rank) {
case Rank::ACE:
std::cout << "Ace";
break;
case Rank::JACK:
std::cout << "Jack";
break;
case Rank::QUEEN:
std::cout << "Queen";
break;
case Rank::KING:
std::cout << "King";
break;
default:
std::cout << static_cast<int>(rank) + 1;
break;
}
std::cout << " of ";
switch (suit) {
case Suit::CLUBS:
std::cout << "Clubs";
break;
case Suit::DIAMONDS:
std::cout << "Diamonds";
break;
case Suit::HEARTS:
std::cout << "Hearts";
break;
case Suit::SPADES:
std::cout << "Spades";
break;
}
std::cout << std::endl;
}
private:
Suit suit;
Rank rank;
};
class Deck {
public:
Deck() {
for (int s = 0; s < 4; ++s) {
for (int r = 0; r < 13; ++r) {
cards.push_back(Card(static_cast<Suit>(s), static_cast<Rank>(r)));
}
}
}
void shuffle() {
std::random_device rd;
std::mt19937 g(rd());
std::shuffle(cards.begin(), cards.end(), g);
}
void display() const {
for (const auto& card : cards) {
card.display();
}
}
private:
std::vector<Card> cards;
};
int main() {
Deck deck;
deck.shuffle();
deck.display();
return 0;
}
We start by defining two enumerations: Suit
and Rank
, which represent the possible suits and ranks of a card, respectively. This provides a structured way to categorize cards based on their properties.
Next, we define a Card
class to encapsulate individual cards. Each card object contains attributes for its suit and rank, along with methods to retrieve and display these properties. This abstraction simplifies card management and enhances code readability.
Subsequently, we define a Deck
class to represent the entire deck of cards. The constructor initializes the deck by creating 52 cards, covering all possible combinations of suits and ranks. This centralizes the creation and management of the deck within a single entity.
The shuffle()
method of the Deck
class employs the Fisher-Yates shuffle algorithm to randomize the order of cards in the deck. This ensures fair and unpredictable card distribution for various applications.
The display()
method of the Deck
class iterates through the cards in the deck, calling the display()
method of each card object to print out its suit and rank. This facilitates easy visualization of the deck’s contents.
In the main()
function, we instantiate a Deck
object, shuffle it to randomize the card order, and then display the shuffled deck. This demonstrates the practical usage of the Deck
class for managing and manipulating a deck of cards.
Upon running the code, you’ll see the output displaying all 52 cards of the shuffled deck, each in the format Rank of Suit
.
This output demonstrates the effective representation of a deck of cards using a custom class in C++. The encapsulation of card properties and behaviors within the Card
and Deck
classes promotes code clarity and maintainability, making it easier to work with decks of cards in C++ programs.
Conclusion
Representing a deck of cards in C++ is crucial for various applications, especially in the development of card games or simulations. Using arrays provides a simple and efficient way to organize and manipulate the cards within a program.
We explored different methods for representing a deck of cards, including using single arrays, two-dimensional arrays, enumerated values, and custom classes. Each method offers its own advantages, such as simplicity, flexibility, or encapsulation of card properties and behaviors.
By choosing the appropriate method based on the requirements of the project, developers can effectively manage and work with decks of cards in C++, enhancing the functionality and user experience of their applications.