Array of Arrays in C++
- Understanding Array of Arrays
- Declaring and Initializing Arrays
- Accessing Array Elements
- Looping Through an Array of Arrays
- Practical Applications of Array of Arrays
- Conclusion
- FAQ
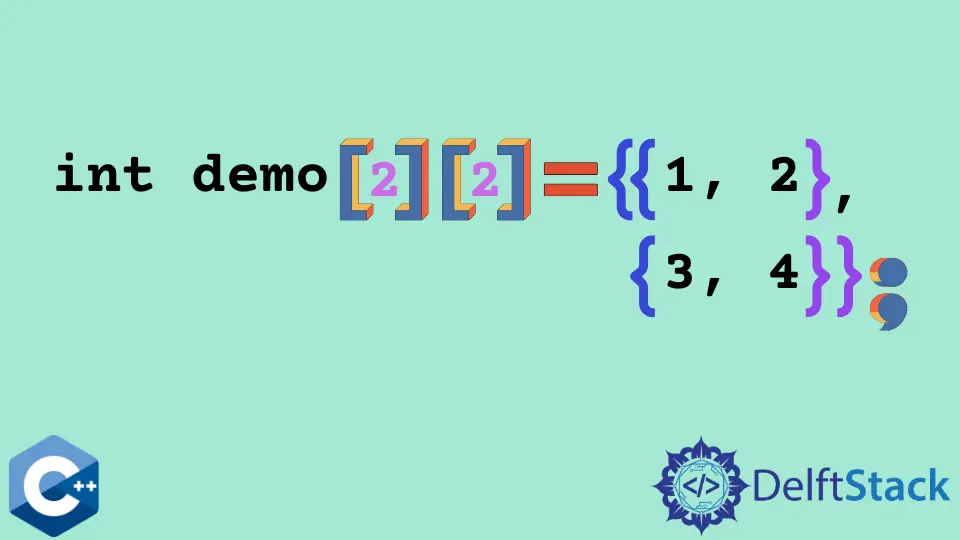
In C++, a multi-dimensional array, often referred to as an array of arrays, is a powerful construct that allows developers to store homogeneous data types in a single block of contiguous memory. This feature is particularly useful when dealing with matrices or tables, where data is organized in rows and columns. Understanding how to effectively utilize arrays of arrays can significantly enhance your programming skills, especially when it comes to handling complex data structures.
In this article, we will explore the concept of arrays of arrays in C++, including their declaration, initialization, and practical examples. Whether you’re a beginner or looking to brush up on your C++ skills, this guide will provide you with the insights you need to master this essential topic.
Understanding Array of Arrays
An array of arrays in C++ allows you to create multi-dimensional data structures. The syntax may initially seem complex, but once you grasp the basics, it becomes intuitive. A multi-dimensional array is essentially an array where each element is also an array. This structure is particularly beneficial for representing matrices, grids, or any data that can be organized in a tabular format.
To declare an array of arrays, you need to specify the size of each dimension. For example, a two-dimensional array can be declared as follows:
int array[3][4];
In this case, array
can hold three rows and four columns of integers. Each element can be accessed using two indices: the first for the row and the second for the column.
Declaring and Initializing Arrays
Declaring and initializing an array of arrays in C++ is straightforward. You can do this in a single line or in multiple lines for better readability. Here’s how you can initialize a two-dimensional array:
int array[2][3] = {
{1, 2, 3},
{4, 5, 6}
};
This code snippet initializes a 2x3 array with specific values. Each row is enclosed in curly braces, and the entire array is enclosed in another pair of braces.
Output:
array[0][0] = 1
array[0][1] = 2
array[0][2] = 3
array[1][0] = 4
array[1][1] = 5
array[1][2] = 6
When you access the elements of this array, you can do so using the indices. For example, array[0][1]
will return the value 2
.
This method of initialization is not only clean but also allows you to set up your data structure in a way that is easy to read and maintain.
Accessing Array Elements
Accessing elements in an array of arrays is done using indices. The first index indicates the row, while the second index indicates the column. Let’s look at a simple example:
#include <iostream>
using namespace std;
int main() {
int array[2][3] = {
{1, 2, 3},
{4, 5, 6}
};
cout << array[1][2]; // Output the element at row 1, column 2
return 0;
}
In this example, we are accessing the element located at the second row and third column, which will output 6
.
Output:
6
Understanding how to access elements in a multi-dimensional array is crucial for manipulating data effectively. You can use loops to traverse through the array, which is particularly useful when dealing with larger datasets.
Looping Through an Array of Arrays
Looping through an array of arrays allows you to perform operations on each element. This is especially useful when you need to process or modify the data. Here’s an example of how to iterate through a two-dimensional array:
#include <iostream>
using namespace std;
int main() {
int array[2][3] = {
{1, 2, 3},
{4, 5, 6}
};
for (int i = 0; i < 2; i++) {
for (int j = 0; j < 3; j++) {
cout << array[i][j] << " ";
}
cout << endl;
}
return 0;
}
In this code, we use nested loops to traverse the rows and columns of the array. Each element is printed in a formatted manner.
Output:
1 2 3
4 5 6
This method of looping is not only efficient but also allows you to perform complex operations, such as summing elements, finding maximum or minimum values, and more.
Practical Applications of Array of Arrays
Arrays of arrays have numerous practical applications in programming. They are widely used in scenarios such as:
- Matrix Operations: Performing calculations on matrices, such as addition, subtraction, and multiplication.
- Game Development: Storing game boards or grids, such as chess or tic-tac-toe.
- Image Processing: Managing pixel data in images, where each pixel can be represented as an RGB value in a two-dimensional array.
By leveraging the capabilities of arrays of arrays, you can create more efficient and organized code. This structure allows you to handle complex data types and improve the overall performance of your applications.
Conclusion
In conclusion, arrays of arrays in C++ are a fundamental concept that can greatly enhance your programming capabilities. By understanding how to declare, initialize, access, and manipulate these multi-dimensional arrays, you can work with complex data structures more effectively. Whether you’re developing algorithms, games, or applications that require data organization, mastering arrays of arrays will be a valuable asset in your programming toolkit.
FAQ
-
What is an array of arrays in C++?
An array of arrays in C++ is a multi-dimensional array that allows you to store homogeneous data in a single block of contiguous memory. -
How do I declare a two-dimensional array in C++?
You can declare a two-dimensional array by specifying the number of rows and columns, like this:int array[rows][columns];
. -
Can I initialize an array of arrays in C++?
Yes, you can initialize an array of arrays at the time of declaration using curly braces. -
How do I access elements in a two-dimensional array?
You access elements by using two indices: the first for the row and the second for the column, likearray[row][column];
. -
What are some practical uses of arrays of arrays?
Arrays of arrays are commonly used in matrix operations, game development, and image processing.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook