How to Deallocate a 2D Array in C++
-
Deallocating 2D Arrays in C++ Using
new
anddelete
-
Deallocating 2D Arrays in C++ Using
malloc
andfree
- Conclusion
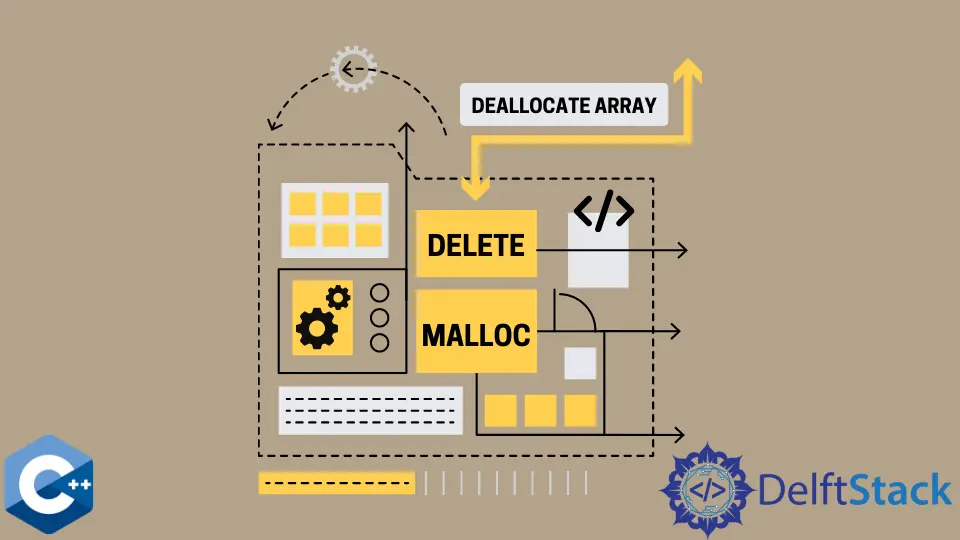
Deallocating memory is crucial to prevent memory leaks and optimize resource usage.
In this article, we will explore the process of deallocating a dynamically allocated 2D array in C++.
When working with 2D arrays, especially dynamically allocated ones, it’s essential to release the allocated memory once it’s no longer needed. Failure to do so can lead to memory leaks, which can degrade the performance of your program over time and, in extreme cases, cause it to crash due to insufficient memory.
In order to avoid having these issues, we are going to discuss how to deallocate a 2D array using various methods in C++.
Deallocating 2D Arrays in C++ Using new
and delete
The new
operator in C++ enables dynamic allocation of a two-dimensional array, while the delete
operator enables subsequent deallocation of the array once it has been used.
In C++, deallocating a 2D array created using new
involves using nested delete
statements. For each memory allocation with new
, you need a corresponding delete
.
When dealing with a 2D array, you release memory for each sub-array (rows) and then delete the array of pointers (columns). This ensures proper deallocation and prevents memory leaks.
Example:
#include <iostream>
// Function to allocate and print a 2D array
int** allocateAndPrint(int rows, int cols) {
int** array2D = new int*[rows];
for (int i = 0; i < rows; ++i) {
array2D[i] = new int[cols];
for (int j = 0; j < cols; ++j) {
array2D[i][j] = i * cols + j;
std::cout << array2D[i][j] << " ";
}
std::cout << std::endl;
}
return array2D;
}
// Function to deallocate a 2D array
void deallocateArray(int** array2D, int rows) {
for (int i = 0; i < rows; ++i) {
delete[] array2D[i];
}
delete[] array2D;
}
int main() {
const int rows = 3;
const int cols = 3;
// Allocate and print the 2D array
int** myArray = allocateAndPrint(rows, cols);
std::cout << "\nDeallocating array\n";
// Deallocate the 2D array
deallocateArray(myArray, rows);
std::cout << "Print array to check if array is deallocated\n";
for (int i = 0; i < rows; ++i) {
std::cout << std::endl;
}
return 0;
}
In order to allocate memory for a 2D array in C++, the process involves creating an array of pointers (array2D
) to represent the rows, with each element pointing to another array of integers representing the columns.
Inside the for
loop, memory is allocated for each row, and array2D[i]
is assigned a pointer to an array of integers representing the columns. The nested for
loop initializes each element with sample data, and the resulting 2D array is printed.
The deallocateArray
function is then employed to iterate through each row, utilizing delete[]
to free the memory allocated for the columns of each row. Finally, delete[]
is used again to release the memory allocated for the array of pointers (rows).
In the main
function, the entire process is encapsulated, calling allocateAndPrint
to create and display the 2D array and subsequently invoking deallocateArray
to release the allocated memory.
Output:
0 1 2
3 4 5
6 7 8
Deallocating array
Print array to check if array is deallocated
The delete
method ensures that both the rows and columns are properly deallocated. By understanding and implementing these concepts, programmers can create more efficient and robust applications.
Deallocating 2D Arrays in C++ Using malloc
and free
The malloc()
and free()
functions in C++ provide a manual and flexible approach to memory allocation and deallocation.
When deallocating a 2D array in C++ created using malloc
, you release memory for each sub-array (rows) using a loop with free
and then free the array of pointers (columns). This process ensures proper deallocation, preventing memory leaks.
Example:
#include <iostream>
#include <cstdlib>
// Function to allocate and print a 2D array
int** allocateAndPrint(int rows, int cols) {
int** array2D = (int**)malloc(rows * sizeof(int*));
for (int i = 0; i < rows; ++i) {
array2D[i] = (int*)malloc(cols * sizeof(int));
for (int j = 0; j < cols; ++j) {
array2D[i][j] = i * cols + j; // Sample data
std::cout << array2D[i][j] << " ";
}
std::cout << std::endl;
}
return array2D;
}
// Function to deallocate a 2D array using malloc and free
void deallocateArray(int** array2D, int rows) {
for (int i = 0; i < rows; ++i) {
free(array2D[i]);
}
free(array2D);
}
int main() {
const int rows = 2;
const int cols = 2;
std::cout << "ARRAY:"<< std::endl;
// Allocate and print the 2D array
int** myArray = allocateAndPrint(rows, cols);
std::cout << "\nDeallocating array\n";
// Deallocate the 2D array
deallocateArray(myArray, rows);
std::cout << "\nPrint array to check if array is deallocated\n";
for (int i = 0; i < rows; ++i) {
std::cout <<std::endl;
}
return 0;
}
The allocateAndPrint
function begins by utilizing malloc
to allocate memory for an array of pointers (array2D
) that represents the rows of our 2D array.
Within the for
loop, malloc
is employed again to allocate memory for each row, creating an array of integers ((int*)malloc(cols * sizeof(int))
) for each row. The nested for
loop fills the array with sample data, and the resulting 2D array is printed to the console.
The deallocateArray
function is responsible for iterating through each row, using free
to release the memory allocated for the columns of each row. Finally, free
is used again to deallocate the memory allocated for the array of pointers (rows).
In the main
function, the entire process can be found, calling allocateAndPrint
to create and display the 2D array and subsequently invoking deallocateArray
to release the allocated memory.
Output:
ARRAY:
0 1
2 3
Deallocating array
Print array to check if array is deallocated
Understanding how to deallocate a 2D array using malloc
and free
is essential for C++ programmers. While modern C++ often favors the use of new
and delete[]
, familiarity with these concepts can be beneficial, especially when working on legacy code or in environments where these functions are preferred.
Conclusion
This article has provided a comprehensive overview of dynamically allocating
and deallocating
2D arrays in C++, offering valuable insights for developers looking to enhance their memory management skills.
The new
and delete
operators are used for dynamic memory allocation and deallocation, respectively. In the context of 2D arrays, they allow us to allocate memory for rows and columns dynamically.
While C++ offers new
and delete
operators for dynamic memory, malloc()
and free()
remain crucial in scenarios requiring compatibility with C, legacy codebases, or specialized memory management.
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn