C++ での 2D 配列の割り当て解除
- C++ での 2D 配列の初期化
-
C++ で
delete
演算子を使用して 2D 配列の割り当てを解除する -
malloc()
およびfree()
演算子を使用して C++ で 2D 配列の割り当てを解除する
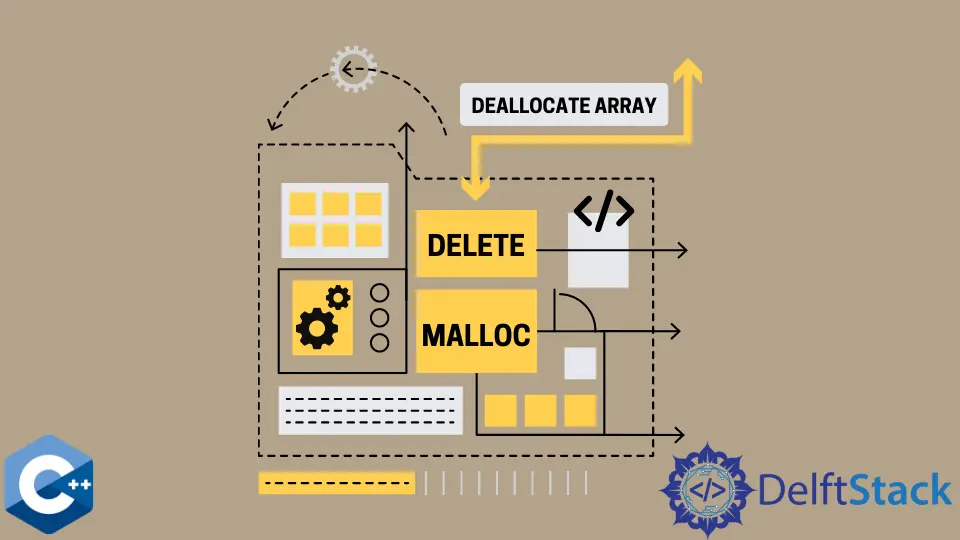
この記事では、C++ プログラミング言語で 2 次元配列を初期化および解放する方法について説明します。
C++ で作成できる多次元配列の最も基本的なバージョンは 2 次元配列です。 matrix
は 2 次元配列の別名です。 初期化方法に応じて、整数、文字、浮動小数点数など、任意の型にすることができます。
C++ の new
演算子は、2 次元配列の動的な割り当てを有効にしますが、delete
演算子は、配列が使用されると、その後の配列の割り当て解除を有効にします。 new
演算子を使用して 2 次元配列を割り当てる場合、いくつかの異なるアプローチを利用できます。
フリー ストアを使用して動的メモリが割り当てられると、作成されたオブジェクトへの参照を取得できます。 ただし、このポインタは、割り当てられた領域の最初のバイトを参照します。
C++ での 2D 配列の初期化
まず、5 行 2 列の arr
という 2 次元配列を使用して、配列の配列を作成します。
int arr[5][2] = {
{11, 12}, {13, 14}, {10, 12}, {14, 16}, {15, 17},
}
以下は、5 行 2 列の 2 次元配列を作成するために使用できる別の方法です。
int arr[5][2] = {11, 12}
2 次元配列の初期化プロセスが完了しました。 ただし、まだ印刷していないため、操作が正しく行われたかどうかは確認できません。
#include <iostream>
using namespace std;
int main() {
int arr[5][2] = {
{11, 12}, {13, 14}, {10, 12}, {14, 16}, {15, 17},
};
int i, j;
cout << "The array is shown as follows:\n" << endl;
for (i = 0; i < 5; i++) {
for (j = 0; j < 2; j++) {
cout << arr[i][j] << "\t";
}
cout << endl;
}
}
出力:
The array is shown as follows:
11 12
13 14
10 12
14 16
15 17
C++ で delete
演算子を使用して 2D 配列の割り当てを解除する
配列の割り当て解除は、C++ で最も重要な機能です。
動的に割り当てられた RAM を解放するには、プログラマの介入が必要です。 データ変数、ポインタ、または配列によって占有されているメモリ領域の割り当て解除または解放は、delete
演算子を使用して実行されます。
次の例では、配列のメモリの割り当てを解除しようとします。配列の最初の整数のみを 0 に設定し、残りはそのままにします。
プログラムの main()
メソッド内で i
と n
というデータ型 int
を持つ 2つの変数を宣言します。
int i, n;
ここで、格納する整数の総数について入力を収集します。
cout << "How many integers do you want to store?" << endl;
cin >> n;
ポインタを使用して pointerArray
と呼ばれる int
型の配列を初期化します。
int* pointerArray = new int[n];
配列にいくつかの数値を入力できるように、ユーザーに何らかの入力を提供させます。 システムに保存したい整数の数に等しい値になるまで、繰り返し入力を受け入れる for
ループを使用します。
for (int i = 0; i < n; i++) {
cout << endl;
cout << "Please input an integer\n";
cin >> pointerArray[i];
}
現在配列に保持されている整数を出力します。 この目的のために、for
ループを使用します。
cout << "Stored array values\n";
for (int i = 0; i < n; i++) {
cout << pointerArray[i] << endl;
}
配列の割り当てを解除するには、まず delete[]
演算子を使用する必要があります。 もう一度、配列を出力して、効果的に割り当てが解除されたことを確認します。
cout << "Deallocating array\n";
delete[] pointerArray;
cout << "Print the array to check if the array is deallocated\n";
for (i = 0; i < n; i++) {
cout << pointerArray[i] << endl;
}
完全なソース コード:
#include <iostream>
using namespace std;
int main() {
int i, n;
cout << "How many integers do you want to store?" << endl;
cin >> n;
int* pointerArray = new int[n];
for (int i = 0; i < n; i++) {
cout << endl;
cout << "Please input an integer\n";
cin >> pointerArray[i];
}
cout << "Stored array values\n";
for (int i = 0; i < n; i++) {
cout << pointerArray[i] << endl;
}
cout << "Deallocating array\n";
delete[] pointerArray;
cout << "Print the array to check if the array is deallocated\n";
for (i = 0; i < n; i++) {
cout << pointerArray[i] << endl;
}
}
出力:
How many integers do you want to store?
2
Please input an integer
2
Please input an integer
3
Stored array values
2
3
Deallocating array
Print the array to check if the array is deallocated
0
0
malloc()
および free()
演算子を使用して C++ で 2D 配列の割り当てを解除する
この例では、malloc()
関数を使用して、int
メモリを 0
のサイズで newPointer
ポインタに割り当てました。
int* newPointer = (int*)malloc(0);
その後、if
ステートメントを使用して、malloc()
がアドレスまたは null
ポインターを提供したかどうかを判断します。 アドレスが生成された場合は、次のステップに進みます。
if (newPointer == NULL) {
cout << "Null value";
} else {
cout << "Address = " << newPointer;
}
最終的に、解放メソッド free()
を使用してメモリを解放しました。
free(newPointer);
完全なソース コード:
#include <cstdlib>
#include <iostream>
using namespace std;
int main() {
int *newPointer = (int *)malloc(0);
if (newPointer == NULL) {
cout << "Null value";
} else {
cout << "Address = " << newPointer;
}
free(newPointer);
return 0;
}
出力:
Address = 0x56063cf64eb0
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn