C++에서 2D 배열 할당 해제
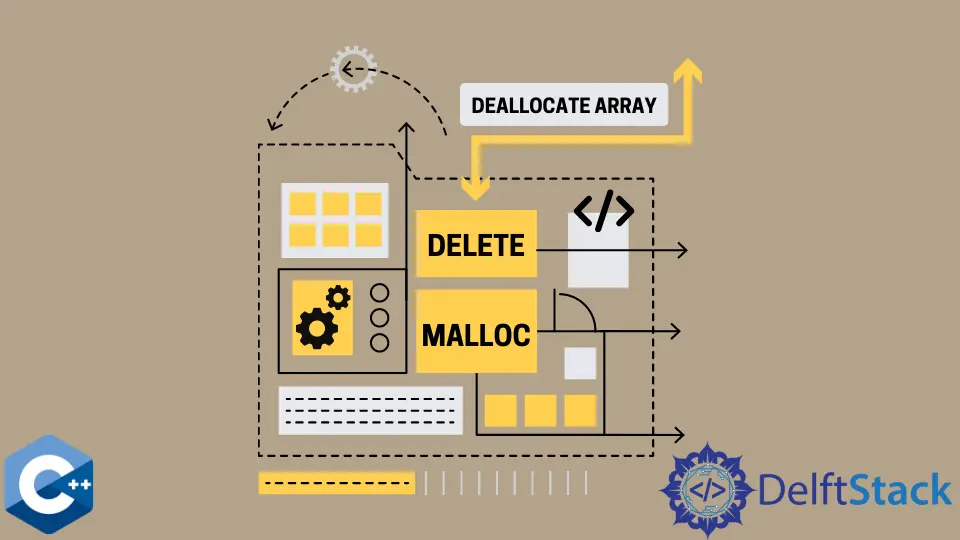
이 기사에서는 C++ 프로그래밍 언어에서 2차원 배열을 초기화하고 할당 해제하는 방법을 설명합니다.
C++에서 만들 수 있는 가장 기본적인 다차원 배열 버전은 2차원 배열입니다. 매트릭스
는 2차원 배열의 또 다른 이름입니다. 초기화 방법에 따라 정수, 문자, 부동 소수점 등을 포함한 모든 유형이 될 수 있습니다.
C++의 new
연산자는 2차원 배열의 동적 할당을 가능하게 하는 반면, delete
연산자는 일단 사용된 배열의 후속 할당 해제를 가능하게 합니다. new
연산자의 도움으로 2차원 배열을 할당할 때 사용할 수 있는 몇 가지 다른 접근 방식이 있습니다.
자유 저장소를 사용하여 동적 메모리를 할당하면 생성된 객체에 대한 참조를 얻을 수 있습니다. 그러나 이 포인터는 할당된 영역의 첫 번째 바이트를 참조합니다.
C++에서 2D 배열 초기화
먼저 5개의 행과 2개의 열이 있는 arr
이라는 2차원 배열을 사용하여 배열 배열을 만듭니다.
int arr[5][2] = {
{11, 12}, {13, 14}, {10, 12}, {14, 16}, {15, 17},
}
다음은 5개의 행과 2개의 열이 있는 2차원 배열을 설정하는 데 사용할 수 있는 또 다른 방법입니다.
int arr[5][2] = {11, 12}
2차원 배열을 초기화하는 과정을 마쳤습니다. 아직 인쇄를 하지 않았기 때문에 작업이 제대로 수행되었는지 확인할 수 없습니다.
#include <iostream>
using namespace std;
int main() {
int arr[5][2] = {
{11, 12}, {13, 14}, {10, 12}, {14, 16}, {15, 17},
};
int i, j;
cout << "The array is shown as follows:\n" << endl;
for (i = 0; i < 5; i++) {
for (j = 0; j < 2; j++) {
cout << arr[i][j] << "\t";
}
cout << endl;
}
}
출력:
The array is shown as follows:
11 12
13 14
10 12
14 16
15 17
delete
연산자를 사용하여 C++에서 2D 배열 할당 해제
배열 할당 해제는 C++에서 가장 중요한 기능입니다.
동적으로 할당된 RAM을 해제하려면 프로그래머 개입이 필요합니다. 데이터 변수, 포인터 또는 배열이 차지하는 메모리 공간 할당 해제 또는 해제는 delete
연산자를 사용하여 수행됩니다.
다음 예제에서는 배열에 대한 메모리 할당을 해제하고 배열의 첫 번째 정수만 0으로 설정하고 나머지는 그대로 둡니다.
프로그램의 main()
메서드 내에서 i
및 n
이라는 int
데이터 유형을 가진 두 개의 변수를 선언합니다.
int i, n;
이제 저장하려는 정수의 총 개수에 대한 입력을 수집할 시간입니다.
cout << "How many integers do you want to store?" << endl;
cin >> n;
포인터를 사용하여 pointerArray
라는 int
유형의 배열을 초기화합니다.
int* pointerArray = new int[n];
배열을 일부 숫자로 채울 수 있도록 사용자가 일부 입력을 제공하도록 합니다. 우리는 시스템에 저장하려는 정수의 수와 같은 값을 가질 때까지 반복하고 입력을 받아들이는 for
루프를 사용합니다.
for (int i = 0; i < n; i++) {
cout << endl;
cout << "Please input an integer\n";
cin >> pointerArray[i];
}
이제 배열에 있는 정수를 출력합니다. 이를 위해 for
루프를 사용합니다.
cout << "Stored array values\n";
for (int i = 0; i < n; i++) {
cout << pointerArray[i] << endl;
}
배열 할당을 해제하려면 먼저 delete[]
연산자를 사용해야 합니다. 다시 한 번 배열을 인쇄하여 효율적으로 할당이 해제되었는지 확인합니다.
cout << "Deallocating array\n";
delete[] pointerArray;
cout << "Print the array to check if the array is deallocated\n";
for (i = 0; i < n; i++) {
cout << pointerArray[i] << endl;
}
전체 소스 코드:
#include <iostream>
using namespace std;
int main() {
int i, n;
cout << "How many integers do you want to store?" << endl;
cin >> n;
int* pointerArray = new int[n];
for (int i = 0; i < n; i++) {
cout << endl;
cout << "Please input an integer\n";
cin >> pointerArray[i];
}
cout << "Stored array values\n";
for (int i = 0; i < n; i++) {
cout << pointerArray[i] << endl;
}
cout << "Deallocating array\n";
delete[] pointerArray;
cout << "Print the array to check if the array is deallocated\n";
for (i = 0; i < n; i++) {
cout << pointerArray[i] << endl;
}
}
출력:
How many integers do you want to store?
2
Please input an integer
2
Please input an integer
3
Stored array values
2
3
Deallocating array
Print the array to check if the array is deallocated
0
0
malloc()
및 free()
연산자를 사용하여 C++에서 2D 배열 할당 해제
이 경우에는 malloc()
함수를 사용하여 newPointer
포인터에 0
크기의 int
메모리를 할당했습니다.
int* newPointer = (int*)malloc(0);
그런 다음 if
문을 사용하여 malloc()
이 주소 또는 null
포인터를 제공했는지 확인합니다. 주소가 생성되면 다음 단계로 넘어갑니다.
if (newPointer == NULL) {
cout << "Null value";
} else {
cout << "Address = " << newPointer;
}
궁극적으로 할당 해제 메서드 free()
를 사용하여 메모리를 확보했습니다.
free(newPointer);
전체 소스 코드:
#include <cstdlib>
#include <iostream>
using namespace std;
int main() {
int *newPointer = (int *)malloc(0);
if (newPointer == NULL) {
cout << "Null value";
} else {
cout << "Address = " << newPointer;
}
free(newPointer);
return 0;
}
출력:
Address = 0x56063cf64eb0
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn