How to Check if an Array Contains an Element in C++
-
Use a
for
Loop to Check if an Array Contains an Element in C++ -
Use
std::find
to Check if an Array Contains an Element in C++ -
Use
Std::Count
to Check if an Array Contains an Element in C++ -
Use
std::binary_search
to Check if an Array Contains an Element in C++ -
Use the
any_of()
Function to Check if an Array Contains an Element in C++ - Use a Custom Function to Check if an Array Contains an Element in C++
- Conclusion
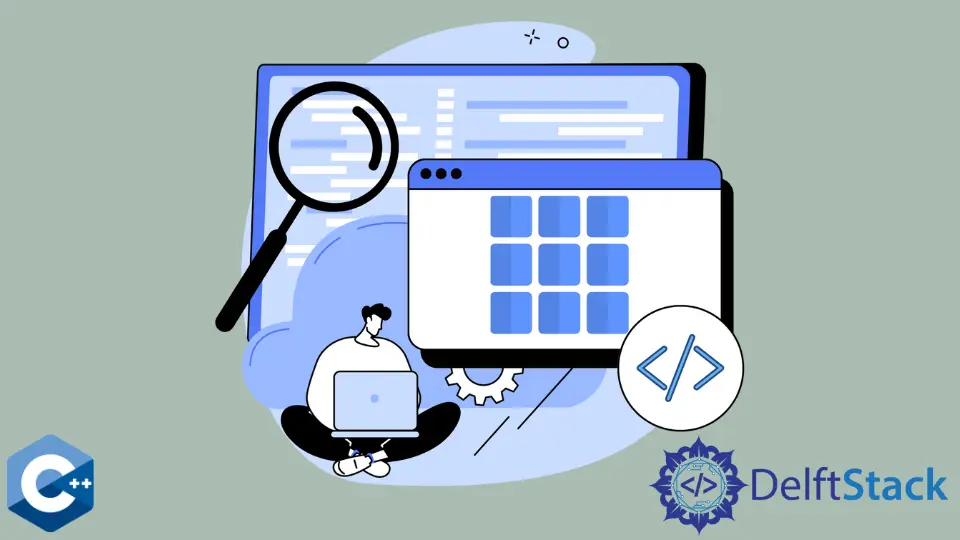
While working with arrays in C++, one might also need to check if an array contains an element. Although this can be done simply by using a loop, other efficient ways can do the same.
This article explores various methods for determining the presence of an element in a C++ array, providing readers with a guide to handling this common task.
Use a for
Loop to Check if an Array Contains an Element in C++
You can use a for
loop to keep things very simple. This method involves iterating through each element of the array and comparing it to the target element to determine if a match is found.
Syntax:
bool present = false;
for (int element : arr) {
if (element == key) {
present = true;
break;
}
}
Parameters:
arr
: This is the name of the array you want to check. It represents the array in which you’re searching for a specific element.key
: This is the element you want to search for within the array. It represents the value you’re trying to find in the array.
Here in the code below, we have an array called valus
and an element we have to search for named target
.
Inside the main
block, we use a for
loop to go over all elements linearly. At each iteration, we check if the current element is the same as the element that we are looking for.
If the target
element is found, the loop breaks and the value of the Boolean variable (present
) gets updated to false
. Later, based on the value of this variable (present
), we print the desired output.
Example code:
#include <algorithm>
#include <iostream>
using namespace std;
int main() {
int values[] = {23, 45, 56, 12, 34, 56};
int target = 56;
bool isPresent = false;
for (int value : values) {
if (value == target) {
isPresent = true;
break;
}
}
if (isPresent) {
cout << "The element is present";
} else {
cout << "The element is not present";
return 0;
}
}
Output:
The element is present
The output of the code is The element is present
because the target
element, which is 56
, is present in the values
array. The for
loop iterates through the array, and when it encounters the value 56
, it sets the present
flag to true
.
While this is the simplest way to search for an element in an array, we’ll explore more efficient methods in the following sections.
Use std::find
to Check if an Array Contains an Element in C++
The std::find
function is primarily used to search for an element within a given range. This function searches for the required element between the range (first, last
).
In the following, the std::find
function, with syntax InputIterator find(InputIterator first, InputIterator last, const T& val);
, helps us search for a value (val
) within a specified range defined by iterators (first
to last
). The iterator type depends on the container or range, such as std::vector<int>::iterator
for a std::vector
.
By calling std::find
with these parameters, we search within the designated range, and if a match is found, it returns an iterator pointing to the first occurrence of the value. Otherwise, it returns the last iterator to signify the absence of the element in the range.
Syntax:
InputIterator find(InputIterator first, InputIterator last, const T& val);
Parameters:
InputIterator first
: This parameter represents the iterator pointing to the beginning of the range where the search is conducted. It specifies the starting point for the search.InputIterator last
: This parameter is another iterator and signifies the end of the search range. It points just beyond the last element in the range, indicating where the search should stop.const T& val
: This parameter is the value that you want to search for within the specified range. It represents the element you are looking for.
Here, in the code below, we used the std::find
function to search for an element in an array. Here, we use a Boolean variable, present
, and the std::find
function to iterate over the array points
.
The function std::find
takes three arguments: the variable data
, the expression data+x
, and the variable target
. The variable data
acts as the iterator for the initial position of the array.
Then, the expression data+x
acts as the iterator for the last position of the array. Lastly, the variable target
acts as the value to be searched for.
If a value is not found, this function returns the iterator to the end of the array, but we can print the desired statement based on the variable present
value.
Example code:
#include <algorithm>
#include <iostream>
using namespace std;
int main() {
int data[] = {23, 45, 56, 12, 34, 56};
int target = 56;
int arraySize = sizeof(data) / sizeof(*data);
bool isPresent = std::find(data, data + arraySize, target) != data + arraySize;
if (isPresent) {
cout << "The element is present";
} else {
cout << "The element is not present";
}
return 0;
}
Output:
The element is present
In the output, the message The element is present
is displayed because the target
value 56
does exist within the data
array.
If passing the arguments mentioned above is confusing, a more convenient approach is to use the begin()
and end()
functions, which directly pass two iterators to the beginning and end of the array, simplifying the code while achieving the same result.
Example code:
#include <algorithm>
#include <iostream>
using namespace std;
int main() {
int values[] = {23, 45, 56, 12, 34, 56};
int target = 56;
bool isElementPresent = std::find(begin(values), end(values), target) != end(values);
if (isElementPresent) {
cout << "The element is present";
} else {
cout << "The element is not present";
}
return 0;
}
Output:
The element is present
In this case, the target
value 56
does indeed exist within the values
array, resulting in the display of the message The element is present
in the output.
See how we can directly use the begin()
and end()
functions to simplify the code. It works just like the arguments in the previous code.
Use Std::Count
to Check if an Array Contains an Element in C++
One more way could be to use the algorithm std::count
. Essentially, this algorithm counts the number of times an element is seen in a given range.
If the returned value of the count is not zero, this implies that the element is present in the array. The std::count
algorithm also counts the occurrences of an element between the range (first, last
).
Syntax:
int counter(Iterator first, Iterator last, T &val)
Parameters:
Iterator first
: This parameter represents the iterator pointing to the beginning of the range. It marks the starting point for the search.Iterator last
: This is another iterator parameter, indicating the end of the range. It points just beyond the last element in the range, defining where the search should conclude.T &val
: This parameter is a reference to the value that you want to count within the specified range. It represents the element whose occurrences you wish to tally.
Here in the code below, we have a set of numbers in an array called value
and a specific number we’re interested in, which is 56
. We want to know how many times 56
appears in the array.
So, we use the std::count
function to count the occurrences of 56
within the values
array.
#include <algorithm>
#include <iostream>
using namespace std;
int main() {
int values[] = {23, 45, 56, 12, 34, 56};
int target = 56;
cout << std::count(begin(values), end(values), target);
}
Output:
2
See how we pass the required arguments to this function and print the result. Since the target
value 56
is present at two places in the array values
, we get the output as 2
.
Now, we will merge this with a Boolean variable, present
, to check if the count of the target
variable is greater than zero or not. If yes, it simply means that the element is present in the array.
#include <algorithm>
#include <iostream>
using namespace std;
int main() {
int values[] = {23, 45, 56, 12, 34, 56};
int target = 56;
bool isElementPresent = std::count(begin(values), end(values), target) > 0;
if (isElementPresent) {
cout << "The element is present";
} else {
cout << "The element is not present";
}
return 0;
}
Output:
The element is present
In our case, the target
value does indeed exist in the values
array, and this is reflected in the output message The element is present
. Naturally, this algorithm is slower in performance than std::find
since it traverses the entire array to find the count of an element.
Use std::binary_search
to Check if an Array Contains an Element in C++
If an array is sorted, the binary search algorithm is the most efficient approach to check if an array contains an entry in C++. The standard library of C++ provides a binary_search
algorithm for doing the same.
The std::binary_search
algorithm returns the value true
if the element is found in the range [first, last)
. Else, it returns false
.
Syntax:
bool std::binary_search(ForwardIterator first, ForwardIterator last, const T& val);
Parameters:
ForwardIterator first
: This parameter represents the iterator pointing to the beginning of the range where the binary search begins. It specifies the start of the search range.ForwardIterator last
: This is another iterator parameter and denotes the end of the search range. It points just beyond the last element in the range, indicating where the search should conclude.const T& val
: This parameter is a reference to the value you want to check for within the specified range. It represents the element you are searching for using the binary search algorithm.
In the code below, we have created a function called checkEle()
inside which we first sort the array using the sort()
function and then use the std::binary_search
algorithm to search for the key
element.
Example code:
#include <algorithm>
#include <iostream>
using namespace std;
bool isElementInArray(int arr[], int size, int target) {
if (size <= 0) {
return false;
}
sort(arr, arr + size);
return std::binary_search(arr, arr + size, target);
}
int main() {
int data[] = {23, 45, 56, 12, 34, 56};
int searchKey = 56;
int size = sizeof(data) / sizeof(*data);
bool isPresent = isElementInArray(data, size, searchKey);
if (isPresent) {
cout << "The element is present";
} else {
cout << "The element is not present";
}
return 0;
}
Output:
The element is present
In our case, the searchKey
value 56
is indeed present in the data
array, which is evident from the output message The element is present
.
It’s important to note that this method is most useful when the array is already sorted. Sorting the array first using the sort()
function increases the time complexity.
Use the any_of()
Function to Check if an Array Contains an Element in C++
Another efficient method to determine if an array contains an element in C++ is by using the any_of()
function. This function applies a specified condition (a unary predicate) to each element in the array, and if the condition evaluates to true
for at least one element, it signifies that the element exists in the array.
In the following syntax, we define a function template called any_of
. It’s designed to check if any element within a specified range, defined by iterators begin
and end
, satisfies a given condition represented by the unary predicate p
.
If the condition is met for at least one element in the range, the function returns true
; otherwise, it returns false
.
Syntax:
template <class InputIterator, class UnaryPredicate>
bool any_of(InputIterator begin, InputIterator end, UnaryPredicate p);
Look at the code to understand how the predicate is defined. Here, along with calling the any_of()
function, we also use the and
condition that simultaneously determines whether the current element is equal to the target
that we are looking for.
If the condition is satisfied for any element, then the value of the Boolean variable present
gets updated to true
.
#include <algorithm>
#include <array>
#include <iostream>
using namespace std;
int main() {
int values[] = {23, 45, 56, 12, 34, 56};
int target = 56;
bool isElementPresent =
std::any_of(begin(values), end(values), [&](int i) { return i == target; });
if (isElementPresent) {
cout << "The element is present";
} else {
cout << "The element is not present";
}
return 0;
}
Output:
The element is present
This method confirms that the target
value 56
indeed exists within the values
array, as indicated by the The element is present
message in the output.
Use a Custom Function to Check if an Array Contains an Element in C++
Using a custom function to check if an array contains an element in C++ involves defining our function that takes as input an array, its size, and the target element we want to find. The function then iterates through the array elements to determine if the target element is present.
Syntax:
template <typename T>
bool customContains(const T* arr, const size_t size, const T& target) {
for (size_t i = 0; i < size; i++) {
if (arr[i] == target) {
return true;
}
}
return false;
}
Parameters:
const T* arr
: This parameter is a pointer to the array that you want to search. It allows the function to work with arrays of any data type, as the template typeT
can be replaced with the appropriate data type.const size_t size
: This parameter represents the size or length of the array. It indicates the number of elements in the array and helps the function iterate through the elements.const T& target
: This parameter specifies the element you want to search for within the array. It’s the element you’re checking for existence in the array.
In the code below, we’ve created a custom function called customContains
that checks if a specific element exists in an array. It’s versatile, working with various data types. The function takes three parameters: an array pointer, array size, and the target element.
Next, we iterate through the array, and if we find a match, the function returns true
, indicating the element is present; otherwise, it returns false
.
Then, in the main
function, we use this custom function to check if the searchKey
value (56
) is in the data
array. The result is stored in present
, and based on its value, we print "The element is present"
or "The element is not present"
.
#include <algorithm>
#include <iostream>
using namespace std;
// Custom function to check if an element exists in an array
template <typename T>
bool doesContain(const T* array, const size_t size, const T& target) {
for (size_t i = 0; i < size; i++) {
if (array[i] == target) {
return true;
}
}
return false;
}
int main() {
int data[] = {23, 45, 56, 12, 34, 56};
int searchKey = 56;
bool isPresent = doesContain(data, sizeof(data) / sizeof(data[0]), searchKey);
if (isPresent) {
cout << "The element is present";
} else {
cout << "The element is not present";
}
return 0;
}
Output:
The element is present
In this case, the searchKey
value 56
is indeed found within the data
array, as indicated by the output message The element is present
.
Conclusion
In this article, we discussed different methods for checking the presence of an element in a C++ array. While a basic for
loop provides a simple solution, we also explored more efficient techniques using standard C++ library functions and algorithms.
The methods mentioned, such as std::find
, std::count
, and std::binary_search
, offer diverse options to achieve the same goal, catering to various needs and preferences. All these methods achieve the same goal, and it’s totally up to you to decide the best approach you like.