在 C++ 中檢查陣列是否包含某元素
- 在 C++ 中使用迴圈來檢查一個陣列是否包含某元素
-
在 C++ 中使用
std::find
來檢查一個陣列是否包含某元素 -
在 C++ 中使用
Std::Count
來檢查一個陣列是否包含某元素 -
在 C++ 中使用
std::binary_search
來檢查一個陣列是否包含某元素 -
在 C++ 中使用
any_of()
函式來檢查一個陣列是否包含某元素 - まとめ
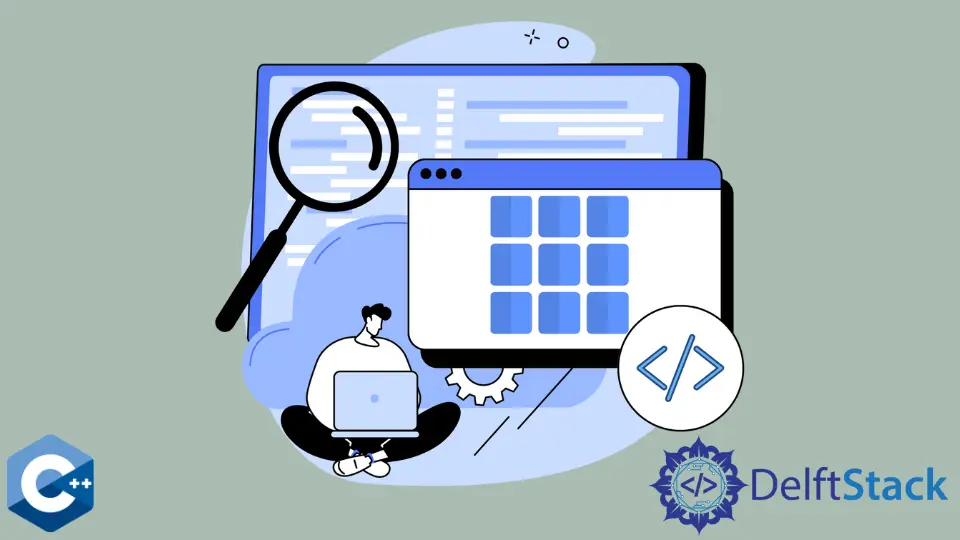
在 C++ 中使用陣列時,可能還需要在 C++ 中檢查一個陣列是否包含一個元素。雖然這可以簡單地使用迴圈來完成,但其他有效的方法也可以做到這一點。
本文將指導你通過各種方法檢查 C++ 中的陣列中是否存在元素。繼續閱讀。
C++ 的標準庫提供了一些演算法和函式,我們可以使用它們來檢查陣列是否包含 C++ 中的元素。但是讓我們首先看看如何使用迴圈來做到這一點。
在 C++ 中使用迴圈來檢查一個陣列是否包含某元素
你可以使用 for
迴圈使事情變得非常簡單。在下面的程式碼中,我們有一個名為 points
的陣列和一個我們必須搜尋的名為 key
的元素。
在 main
塊內,我們使用 for
迴圈線性遍歷所有元素。在每次迭代中,我們檢查當前元素是否與我們正在尋找的元素相同。
如果找到了 key
元素,迴圈中斷,布林變數 present
的值更新為 false
。稍後,根據這個變數的值 present
,我們列印所需的輸出。
示例程式碼:
#include <algorithm>
#include <iostream>
using namespace std;
int main() {
int points[] = {23, 45, 56, 12, 34, 56};
int key = 56;
bool present = false;
for (int i : points) {
if (i == key) {
present = true;
break;
}
}
if (present) {
cout << "The element is present";
} else {
cout << "The elment is not present";
return 0;
}
輸出:
The element is present
雖然這是在陣列中搜尋元素的最簡單方法,但還有其他更好的方法可以做到這一點。我們將在以下部分討論它們。
在 C++ 中使用 std::find
來檢查一個陣列是否包含某元素
std::find
函式主要用於搜尋特定範圍內的元素。此函式在範圍 [first, last)
之間搜尋所需的元素。
語法:
InputIterator find(InputIterator first, InputIterator last, const T& val);
下面是使用 std::find
函式在陣列中搜尋元素的程式碼。在這裡,我們使用布林變數 present
和 std::find
函式來迭代陣列 points
。
std::find
函式接受三個引數:
- 變數
points
,作為陣列初始位置的迭代器 - 表示式
points+x
,作為陣列最後位置的迭代器 - 變數
key
,這是要搜尋的值
如果未找到值,此函式將迭代器返回到陣列的末尾,但我們可以根據變數 present
值列印所需的語句。
示例程式碼:
#include <algorithm>
#include <iostream>
using namespace std;
int main() {
int points[] = {23, 45, 56, 12, 34, 56};
int key = 56;
int x = sizeof(points) / sizeof(*points);
bool present = std::find(points, points + x, key) != points + x;
if (present) {
cout << "The element is present";
} else {
cout << "The element is not present";
}
return 0;
}
輸出:
The element is present
如果傳遞上述引數令人困惑,你還可以分別使用 begin()
和 end()
函式將兩個迭代器傳遞到陣列的開頭和結尾。
示例程式碼:
#include <algorithm>
#include <iostream>
using namespace std;
int main() {
int points[] = {23, 45, 56, 12, 34, 56};
int key = 56;
bool present = std::find(begin(points), end(points), key) != end(points);
if (present) {
cout << "The element is present";
} else {
cout << "The element is not present";
}
return 0;
}
輸出:
The element is present
看看我們如何直接使用 begin()
和 end()
函式來簡化程式碼。它就像前面程式碼中的引數一樣工作。
在 C++ 中使用 Std::Count
來檢查一個陣列是否包含某元素
另一種方法是使用演算法 std::count
。本質上,該演算法計算元素在給定範圍內出現的次數。
如果計數的返回值不為零,這意味著該元素存在於陣列中。std::count
演算法還計算範圍 [first, last)
之間元素的出現次數。
語法:
int counter(Iterator first, Iterator last, T &val)
檢視程式碼以瞭解其工作原理。
#include <algorithm>
#include <iostream>
using namespace std;
int main() {
int points[] = {23, 45, 56, 12, 34, 56};
int key = 56;
cout << std::count(begin(points), end(points), key);
}
輸出:
2
看看我們如何將所需的引數傳遞給這個函式並列印結果。由於 key
值 56
出現在陣列 points
中的兩個位置,我們得到輸出為 2
。
現在,我們將它與布林變數 present
合併,以檢查 key
變數的計數是否大於零。如果是,則僅表示該元素存在於陣列中。
#include <algorithm>
#include <iostream>
using namespace std;
int main() {
int points[] = {23, 45, 56, 12, 34, 56};
int key = 56;
bool present = std::count(begin(points), end(points), key) > 0;
if (present) {
cout << "The element is present";
} else {
cout << "The element is not present";
}
return 0;
}
輸出:
The element is present
自然,該演算法的效能比 std::find
慢,因為它遍歷整個陣列以查詢元素的計數。
在 C++ 中使用 std::binary_search
來檢查一個陣列是否包含某元素
如果陣列已排序,在 C++ 中檢查陣列是否包含元素的最有效方法是使用二進位制搜尋演算法。C++ 的標準庫提供了一個 binary_search
演算法來做同樣的事情。
如果在 [first, last)
範圍內找到元素,std::binary_search
演算法將返回值 true
。否則,它返回 false
。
在下面的程式碼中,我們建立了一個名為 checkEle()
的函式,其中我們首先使用 sort()
函式對陣列進行排序,然後使用 std::binary_search
演算法搜尋 key
元素.
示例程式碼:
#include <algorithm>
#include <iostream>
using namespace std;
bool checkEle(int a[], int x, int key) {
if (x <= 0) {
return false;
}
sort(a, a + x);
return std::binary_search(a, a + x, key);
}
int main() {
int points[] = {23, 45, 56, 12, 34, 56};
int key = 56;
int x = sizeof(points) / sizeof(*points);
bool present = checkEle(points, x, key);
if (present) {
cout << "The element is present";
} else {
cout << "The element is not present";
}
return 0;
}
輸出:
The element is present
這僅在陣列已經排序時有用,因為首先使用 sort()
函式對陣列進行排序會進一步增加時間複雜度。
在 C++ 中使用 any_of()
函式來檢查一個陣列是否包含某元素
我們可以使用 any_of()
函式來檢查謂詞是否符合給定範圍內的任何元素。如果是,則返回 true
;否則,它返回 false
。
語法:
template <class InputIterator, class UnaryPredicate>
bool any_of(InputIterator begin, InputIterator end, UnaryPredicate p);
檢視程式碼以瞭解謂詞是如何定義的。在這裡,除了呼叫 any_of()
函式外,我們還使用 and
條件來同時檢查當前元素是否等於我們正在搜尋的 key
。
如果任何元素都滿足條件,則布林變數 present
的值將更新為 true
。
#include <algorithm>
#include <array>
#include <iostream>
using namespace std;
int main() {
int points[] = {23, 45, 56, 12, 34, 56};
int key = 56;
bool present =
std::any_of(begin(points), end(points), [&](int i) { return i == key; });
if (present) {
cout << "The element is present";
} else {
cout << "The element is not present";
}
return 0;
}
輸出:
The element is present
這就是 any_of()
函式如何在陣列中搜尋元素的方式。這就是我們如何在 C++ 中搜尋陣列中的元素。
まとめ
本文討論了在 C++ 中檢查陣列是否包含元素的各種方法。我們看到了如何在 C++ 中使用簡單的 for
迴圈,並且還使用了諸如 std::find
、std::count
和 std::binary_search
等演算法。
雖然,所有這些方法都達到了相同的目標。完全由你決定你喜歡的最佳方法。