C++ 函式中的使用者輸入陣列
Mohd Mohtashim Nawaz
2023年10月12日
C++
C++ Array
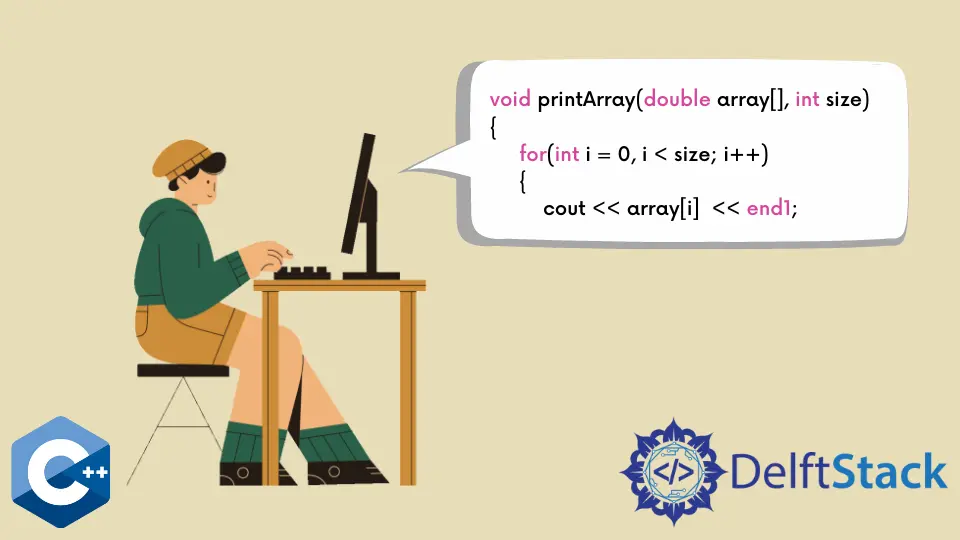
本文討論了在 C++ 中將使用者的輸入輸入到函式中的陣列中的方法。
將陣列作為 C++ 函式中的使用者輸入
在 c++ 的函式中,有三種方法可以將陣列作為使用者輸入。
- 宣告一個全域性陣列。
- 在函式中宣告一個陣列。
- 在 main 函式中宣告一個陣列,傳給函式。
通過宣告全域性陣列在函式中輸入陣列
要獲取使用者對陣列的輸入,你必須有權訪問該陣列。最簡單的方法之一是宣告一個全域性陣列。
你可以在 C++ 中宣告一個全域性陣列,只需在全域性範圍內的所有函式之外宣告它即可。你還必須宣告一個儲存陣列大小的全域性變數。
宣告陣列後,必須使用任意迴圈遍歷所有陣列元素,並將使用者輸入儲存到當前陣列索引中。
原始碼:
#include <iostream>
using namespace std;
int size = 5;
int arr[5];
void userInput() {
cout << "Enter array elements" << endl;
for (int i = 0; i < size; i++) {
cin >> arr[i];
}
}
void print() {
cout << "Array elements are:" << endl;
for (int i = 0; i < size; i++) cout << arr[i] << " ";
cout << endl;
}
int main() {
userInput();
print();
return 0;
}
請注意,在這種情況下,你必須通過整數指定陣列的大小,而不是通過諸如 size
之類的變數。
輸出:
Enter array elements
1 8 5 3 7
Array elements are:
1 8 5 3 7
通過在函式中宣告陣列,使用者在函式中輸入陣列
訪問陣列並將使用者資料輸入其中的另一種方法是在函式本身內宣告陣列。
這樣,函式就有了一個區域性陣列,你可以使用迴圈遍歷它。然後,你可以請求使用者輸入並將其儲存在陣列中。
原始碼:
#include <iostream>
using namespace std;
void userInput() {
int size = 5;
int arr[size];
cout << "Enter array elements" << endl;
for (int i = 0; i < size; i++) {
cin >> arr[i];
}
cout << "Array elements are:" << endl;
for (int i = 0; i < size; i++) cout << arr[i] << " ";
cout << endl;
}
int main() {
userInput();
return 0;
}
輸出:
Enter array elements
8 4 7 2 2
Array elements are:
8 4 7 2 2
通過在主函式中宣告陣列,使用者在函式中輸入陣列
如果在主函式中宣告陣列,則不能在接受使用者輸入的函式內部直接訪問它。因此,你必須將陣列作為引數傳遞給函式和陣列的大小。
由於預設情況下陣列是通過引用傳遞的,因此你在函式中對陣列所做的更改將顯示在主函式的陣列中。你將能夠以這種方式完成作業。
原始碼:
#include <iostream>
using namespace std;
void userInput(int arr[], int size) {
cout << "Enter array elements" << endl;
for (int i = 0; i < size; i++) {
cin >> arr[i];
}
}
void print(int arr[], int size) {
cout << "Array elements are:" << endl;
for (int i = 0; i < size; i++) cout << arr[i] << " ";
cout << endl;
}
int main() {
int size = 5;
int arr[size];
userInput(arr, size);
print(arr, size);
return 0;
}
陣列可以作為指標傳送,例如 int arr
。它不會有任何區別。
輸出:
Enter array elements
4 5 9 7 2
Array elements are:
4 5 9 7 2
まとめ
你已經看到了在 C++ 中將使用者資料輸入到函式陣列中的三種不同方法。
所有三種方法都可以正常工作,但建議在主函式中定義你的陣列(如在最後一種方法中),以便你也可以將其傳遞給其他函式,而不會產生與全域性變數相關的歧義.
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe