Passa un array 2D a una funzione in C++
-
Usa la notazione
[]
per passare array 2D come parametro di funzione -
Usa la notazione
&
per passare array 2D come parametro di funzione
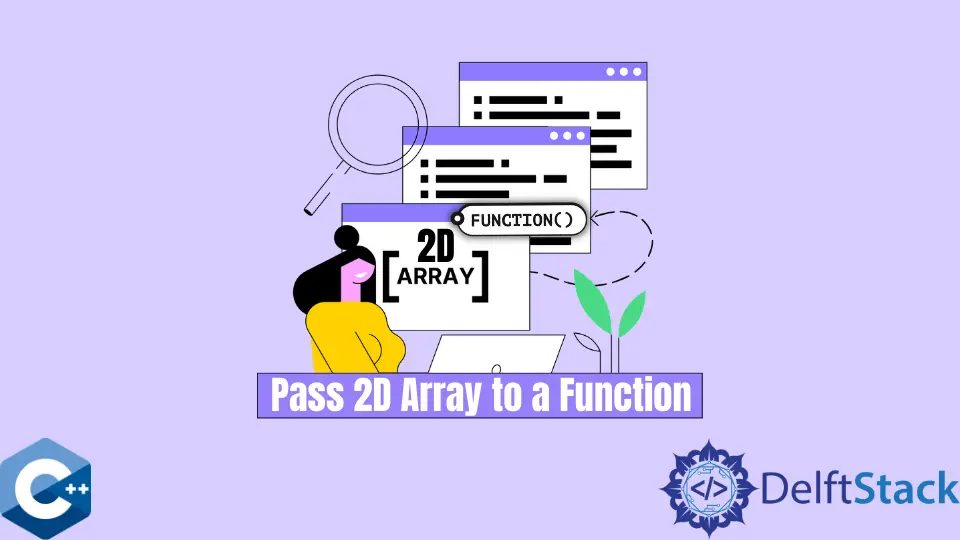
Questo articolo introdurrà come passare un array 2D come parametro di funzione in C++.
Usa la notazione []
per passare array 2D come parametro di funzione
Per dimostrare questo metodo, definiamo un array bidimensionale di lunghezza fissa chiamato c_array
e per moltiplicare ogni suo elemento per 2 passeremo come parametro a una funzione MultiplyArrayByTwo
. Si noti che questa funzione è di tipo void
e opera direttamente sull’oggetto c_array
. In questo modo, accediamo direttamente alla versione moltiplicata dell’array 2D dalla routine main
.
#include <iomanip>
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::setw;
using std::string;
using std::vector;
constexpr int size = 4;
void MultiplyArrayByTwo(int arr[][size], int len) {
for (int i = 0; i < len; ++i) {
for (int j = 0; j < len; ++j) {
arr[i][j] *= 2;
}
}
}
int main() {
int c_array[size][size] = {
{1, 2, 3, 4}, {5, 6, 7, 8}, {9, 10, 11, 12}, {13, 14, 15, 16}};
cout << "input array\n";
for (int i = 0; i < size; ++i) {
cout << " [ ";
for (int j = 0; j < size; ++j) {
cout << setw(2) << c_array[i][j] << ", ";
}
cout << "]" << endl;
}
MultiplyArrayByTwo(c_array, size);
cout << "output array\n";
for (int i = 0; i < size; ++i) {
cout << " [ ";
for (int j = 0; j < size; ++j) {
cout << setw(2) << c_array[i][j] << ", ";
}
cout << "]" << endl;
}
cout << endl;
return EXIT_SUCCESS;
}
Produzione:
input array
[ 1, 2, 3, 4, ]
[ 5, 6, 7, 8, ]
[ 9, 10, 11, 12, ]
[ 13, 14, 15, 16, ]
output array
[ 2, 4, 6, 8, ]
[ 10, 12, 14, 16, ]
[ 18, 20, 22, 24, ]
[ 26, 28, 30, 32, ]
Usa la notazione &
per passare array 2D come parametro di funzione
In alternativa, puoi usare la notazione di riferimento &
per passare un array 2D come parametro. Tieni presente, tuttavia, che questi 2 metodi sono compatibili solo con array di lunghezza fissa dichiarati nello stack. Si noti che abbiamo modificato il bucle della funzione MultiplyArrayByTwo
con un intervallo basato solo per motivi di leggibilità.
#include <iomanip>
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::setw;
using std::string;
using std::vector;
constexpr int size = 4;
void MultiplyArrayByTwo(int (&arr)[size][size]) {
for (auto& i : arr) {
for (int& j : i) j *= 2;
}
}
int main() {
int c_array[size][size] = {
{1, 2, 3, 4}, {5, 6, 7, 8}, {9, 10, 11, 12}, {13, 14, 15, 16}};
cout << "input array\n";
for (int i = 0; i < size; ++i) {
cout << " [ ";
for (int j = 0; j < size; ++j) {
cout << setw(2) << c_array[i][j] << ", ";
}
cout << "]" << endl;
}
MultiplyArrayByTwo(c_array);
cout << "output array\n";
for (int i = 0; i < size; ++i) {
cout << " [ ";
for (int j = 0; j < size; ++j) {
cout << setw(2) << c_array[i][j] << ", ";
}
cout << "]" << endl;
}
cout << endl;
return EXIT_SUCCESS;
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook