Trova il valore massimo nell'array in C++
- Usa il metodo iterativo per trovare il valore massimo in un array C++
-
Usa l’algoritmo
std::max_element
per trovare il valore massimo in un array C++ -
Usa l’algoritmo
std::minmax_element
per trovare il valore massimo in un array C++
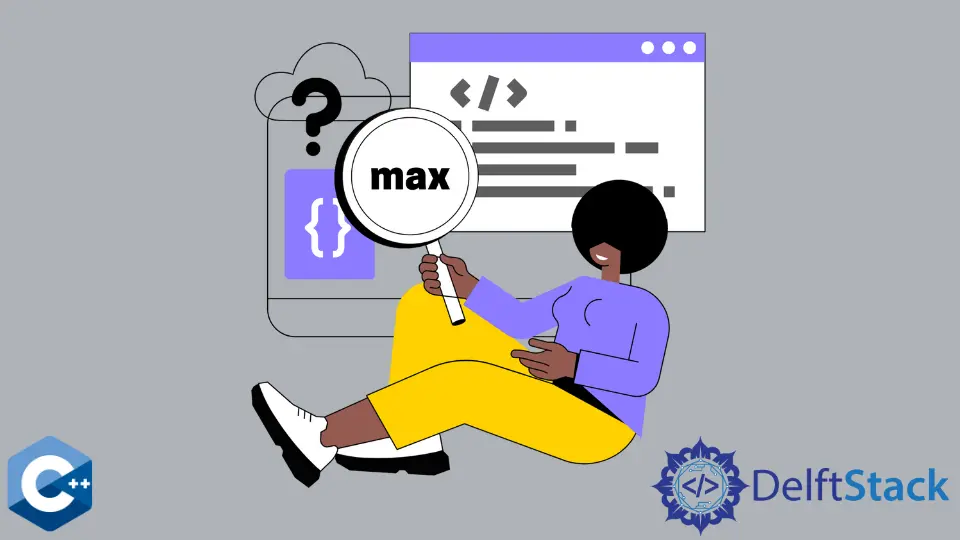
Questo articolo introdurrà come trovare il valore massimo in un array in C++.
Usa il metodo iterativo per trovare il valore massimo in un array C++
Il modo più semplice per implementare una funzione personalizzata per la ricerca del valore massimo consiste nell’usare il metodo iterativo. Il codice di esempio seguente ha la struttura del cicli for
che scorre ogni elemento dell’array e verifica se il valore corrente è maggiore del valore massimo corrente. Si noti che il valore massimo corrente viene inizializzato con il valore del primo elemento nell’array e modificato quando la condizione if
è vera.
#include <sys/time.h>
#include <ctime>
#include <iostream>
using std::cout;
using std::endl;
void generateNumbers(int arr[], size_t &width) {
std::srand(std::time(nullptr));
for (size_t i = 0; i < width; i++) {
arr[i] = std::rand();
}
}
template <typename T>
T FindMax(T *arr, size_t n) {
int max = arr[0];
for (size_t j = 0; j < n; ++j) {
if (arr[j] > max) {
max = arr[j];
}
}
return max;
}
float time_diff(struct timeval *start, struct timeval *end) {
return (end->tv_sec - start->tv_sec) + 1e-6 * (end->tv_usec - start->tv_usec);
}
int main() {
struct timeval start {};
struct timeval end {};
size_t width = 100000;
int *arr = new int[width];
generateNumbers(arr, width);
gettimeofday(&start, nullptr);
cout << "Maximum element is: " << FindMax(arr, width) << endl;
gettimeofday(&end, nullptr);
printf("FindMax: %0.8f sec\n", time_diff(&start, &end));
delete[] arr;
return EXIT_SUCCESS;
}
Produzione:
Maximum element is: 2147460568
FindMax: 0.00017500 sec
Usa l’algoritmo std::max_element
per trovare il valore massimo in un array C++
std::max_element
è un altro metodo per trovare il valore massimo nell’intervallo specificato. Fa parte degli algoritmi STL e l’overload più semplice richiede solo due iteratori per indicare i bordi dell’intervallo da cercare. std::max_element
restituisce un iteratore all’elemento di valore massimo. Se più elementi hanno lo stesso valore e contemporaneamente sono massimi, la funzione restituisce l’iteratore che punta al primo.
#include <sys/time.h>
#include <ctime>
#include <iostream>
using std::cout;
using std::endl;
void generateNumbers(int arr[], size_t &width) {
std::srand(std::time(nullptr));
for (size_t i = 0; i < width; i++) {
arr[i] = std::rand();
}
}
template <typename T>
T FindMax2(T *arr, size_t n) {
return *std::max_element(arr, arr + n);
}
float time_diff(struct timeval *start, struct timeval *end) {
return (end->tv_sec - start->tv_sec) + 1e-6 * (end->tv_usec - start->tv_usec);
}
int main() {
struct timeval start {};
struct timeval end {};
size_t width = 100000;
int *arr = new int[width];
generateNumbers(arr, width);
gettimeofday(&start, nullptr);
cout << "Maximum element is: " << FindMax2(arr, width) << endl;
gettimeofday(&end, nullptr);
printf("FindMax2: %0.8f sec\n", time_diff(&start, &end));
delete[] arr;
return EXIT_SUCCESS;
}
Produzione:
Maximum element is: 2147413532
FindMax2: 0.00023700 sec
Usa l’algoritmo std::minmax_element
per trovare il valore massimo in un array C++
In alternativa, possiamo usare l’algoritmo std::minmax_element
da STL per trovare sia gli elementi minimo che massimo nell’intervallo dato e restituirli come std::pair
. La funzione minmax_element
può opzionalmente prendere una funzione di confronto binario personalizzata come terzo argomento. In caso contrario, ha gli stessi parametri di max_element
e si comporta in modo simile quando nell’intervallo vengono trovati più elementi min/max.
#include <sys/time.h>
#include <ctime>
#include <iostream>
using std::cout;
using std::endl;
void generateNumbers(int arr[], size_t &width) {
std::srand(std::time(nullptr));
for (size_t i = 0; i < width; i++) {
arr[i] = std::rand();
}
}
template <typename T>
auto FindMinMax(T *arr, size_t n) {
return std::minmax_element(arr, arr + n);
}
float time_diff(struct timeval *start, struct timeval *end) {
return (end->tv_sec - start->tv_sec) + 1e-6 * (end->tv_usec - start->tv_usec);
}
int main() {
struct timeval start {};
struct timeval end {};
size_t width = 100000;
int *arr = new int[width];
generateNumbers(arr, width);
gettimeofday(&start, nullptr);
auto ret = FindMinMax(arr, width);
gettimeofday(&end, nullptr);
cout << "MIN element is: " << *ret.first << " MAX element is: " << *ret.second
<< endl;
printf("FindMinMax: %0.8f sec\n", time_diff(&start, &end));
delete[] arr;
return EXIT_SUCCESS;
}
Produzione:
MIN element is: 3843393 MAX element is: 2147251693
FindMinMax: 0.00000400 sec
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook