Cómo pasar el array 2D a una función en C++
-
Usar la
[]
Notación para pasar un array 2D como un parámetro de función -
Usar la notación
&
para pasar el array 2D como parámetro de la función
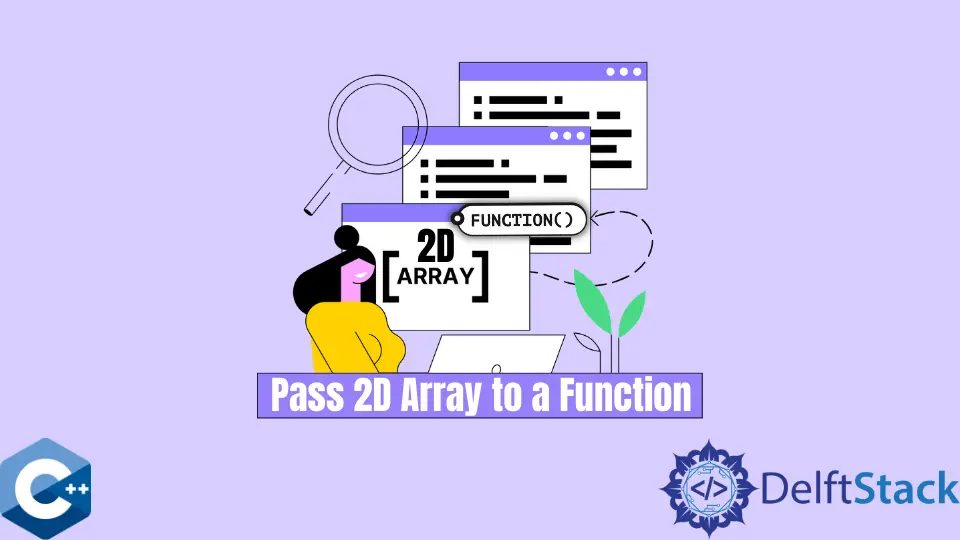
Este artículo presentará cómo pasar un array 2D como parámetro de función en C++.
Usar la []
Notación para pasar un array 2D como un parámetro de función
Para demostrar este método, definimos un array bidimensional de longitud fija llamado c_array
y para multiplicar cada uno de sus elementos por 2 pasaremos como parámetro a una función MultiArrayByTwo
. Noten que esta función es de tipo void
y opera directamente sobre el objeto c_array
. De esta manera, accederemos directamente a la versión multiplicada del array 2D desde la rutina main
.
#include <iomanip>
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::setw;
using std::string;
using std::vector;
constexpr int size = 4;
void MultiplyArrayByTwo(int arr[][size], int len) {
for (int i = 0; i < len; ++i) {
for (int j = 0; j < len; ++j) {
arr[i][j] *= 2;
}
}
}
int main() {
int c_array[size][size] = {
{1, 2, 3, 4}, {5, 6, 7, 8}, {9, 10, 11, 12}, {13, 14, 15, 16}};
cout << "input array\n";
for (int i = 0; i < size; ++i) {
cout << " [ ";
for (int j = 0; j < size; ++j) {
cout << setw(2) << c_array[i][j] << ", ";
}
cout << "]" << endl;
}
MultiplyArrayByTwo(c_array, size);
cout << "output array\n";
for (int i = 0; i < size; ++i) {
cout << " [ ";
for (int j = 0; j < size; ++j) {
cout << setw(2) << c_array[i][j] << ", ";
}
cout << "]" << endl;
}
cout << endl;
return EXIT_SUCCESS;
}
Resultado:
input array
[ 1, 2, 3, 4, ]
[ 5, 6, 7, 8, ]
[ 9, 10, 11, 12, ]
[ 13, 14, 15, 16, ]
output array
[ 2, 4, 6, 8, ]
[ 10, 12, 14, 16, ]
[ 18, 20, 22, 24, ]
[ 26, 28, 30, 32, ]
Usar la notación &
para pasar el array 2D como parámetro de la función
Alternativamente, puedes usar la notación de referencia &
para pasar un array 2D como parámetro. Sin embargo, ten en cuenta que estos 2 métodos son compatibles sólo con arrays de longitudes fijas declaradas en la pila. Note que cambiamos el bucle de la función MultiplyArrayByTwo
con base en el rango sólo por razones de legibilidad.
#include <iomanip>
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::setw;
using std::string;
using std::vector;
constexpr int size = 4;
void MultiplyArrayByTwo(int (&arr)[size][size]) {
for (auto& i : arr) {
for (int& j : i) j *= 2;
}
}
int main() {
int c_array[size][size] = {
{1, 2, 3, 4}, {5, 6, 7, 8}, {9, 10, 11, 12}, {13, 14, 15, 16}};
cout << "input array\n";
for (int i = 0; i < size; ++i) {
cout << " [ ";
for (int j = 0; j < size; ++j) {
cout << setw(2) << c_array[i][j] << ", ";
}
cout << "]" << endl;
}
MultiplyArrayByTwo(c_array);
cout << "output array\n";
for (int i = 0; i < size; ++i) {
cout << " [ ";
for (int j = 0; j < size; ++j) {
cout << setw(2) << c_array[i][j] << ", ";
}
cout << "]" << endl;
}
cout << endl;
return EXIT_SUCCESS;
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook