如何在 C++ 中将 2D 数组传递给函数
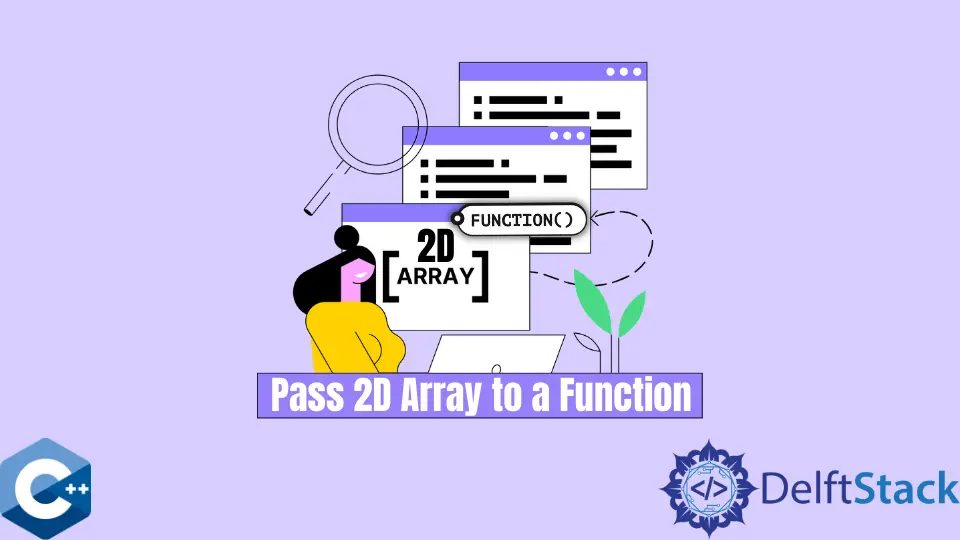
本文将介绍如何在 C++ 中传递一个 2D 数组作为函数参数。
使用 []
记号传递 2D 数组作为函数参数
为了演示这个方法,我们定义一个固定长度的二维数组,命名为 c_array
,为了将它的每个元素乘以 2,我们将传递一个 MultiplyArrayByTwo
函数作为参数。请注意,这个函数是一个 void
类型,直接对 c_array
对象进行操作。这样,我们将直接从 main
例程中访问 2D 数组的乘法版本。
#include <iomanip>
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::setw;
using std::string;
using std::vector;
constexpr int size = 4;
void MultiplyArrayByTwo(int arr[][size], int len) {
for (int i = 0; i < len; ++i) {
for (int j = 0; j < len; ++j) {
arr[i][j] *= 2;
}
}
}
int main() {
int c_array[size][size] = {
{1, 2, 3, 4}, {5, 6, 7, 8}, {9, 10, 11, 12}, {13, 14, 15, 16}};
cout << "input array\n";
for (int i = 0; i < size; ++i) {
cout << " [ ";
for (int j = 0; j < size; ++j) {
cout << setw(2) << c_array[i][j] << ", ";
}
cout << "]" << endl;
}
MultiplyArrayByTwo(c_array, size);
cout << "output array\n";
for (int i = 0; i < size; ++i) {
cout << " [ ";
for (int j = 0; j < size; ++j) {
cout << setw(2) << c_array[i][j] << ", ";
}
cout << "]" << endl;
}
cout << endl;
return EXIT_SUCCESS;
}
输出:
input array
[ 1, 2, 3, 4, ]
[ 5, 6, 7, 8, ]
[ 9, 10, 11, 12, ]
[ 13, 14, 15, 16, ]
output array
[ 2, 4, 6, 8, ]
[ 10, 12, 14, 16, ]
[ 18, 20, 22, 24, ]
[ 26, 28, 30, 32, ]
使用&
符号传递 2D 数组作为函数参数
另外,你也可以使用&
引用符号来传递一个 2D 数组作为参数。但要注意,这两种方法只与堆栈上声明的固定长度数组兼容。请注意,我们把 MultiplyArrayByTwo
函数循环改成了基于范围的,这只是出于可读性的考虑。
#include <iomanip>
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::setw;
using std::string;
using std::vector;
constexpr int size = 4;
void MultiplyArrayByTwo(int (&arr)[size][size]) {
for (auto& i : arr) {
for (int& j : i) j *= 2;
}
}
int main() {
int c_array[size][size] = {
{1, 2, 3, 4}, {5, 6, 7, 8}, {9, 10, 11, 12}, {13, 14, 15, 16}};
cout << "input array\n";
for (int i = 0; i < size; ++i) {
cout << " [ ";
for (int j = 0; j < size; ++j) {
cout << setw(2) << c_array[i][j] << ", ";
}
cout << "]" << endl;
}
MultiplyArrayByTwo(c_array);
cout << "output array\n";
for (int i = 0; i < size; ++i) {
cout << " [ ";
for (int j = 0; j < size; ++j) {
cout << setw(2) << c_array[i][j] << ", ";
}
cout << "]" << endl;
}
cout << endl;
return EXIT_SUCCESS;
}
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Jinku Hu