Comment passer d'un tableau en 2D à une fonction en C++
-
Utiliser la notation
[]
pour passer un tableau 2D en tant que paramètre de fonction -
Utiliser la notation
&
pour passer un tableau 2D comme paramètre de fonction
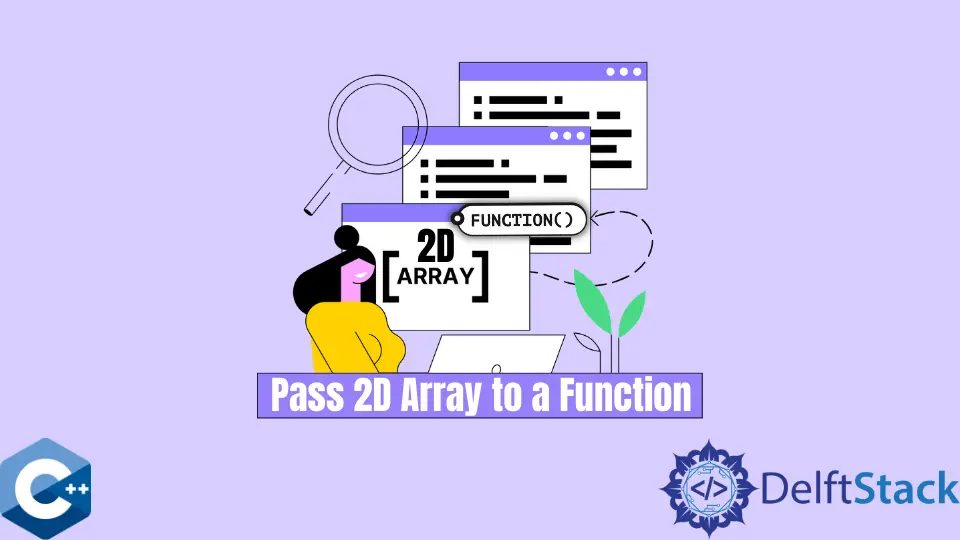
Cet article présente la façon de passer un tableau 2D comme paramètre de fonction en C++.
Utiliser la notation []
pour passer un tableau 2D en tant que paramètre de fonction
Pour démontrer cette méthode, nous définissons un tableau bidimensionnel de longueur fixe nommé c_array
et pour multiplier chaque élément par 2, nous passons en paramètre à une fonction MultiplyArrayByTwo
. Notez que cette fonction est de type void
et qu’elle opère directement sur l’objet c_array
. De cette façon, nous accéderons directement à la version multipliée du tableau 2D depuis la routine main
.
#include <iomanip>
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::setw;
using std::string;
using std::vector;
constexpr int size = 4;
void MultiplyArrayByTwo(int arr[][size], int len) {
for (int i = 0; i < len; ++i) {
for (int j = 0; j < len; ++j) {
arr[i][j] *= 2;
}
}
}
int main() {
int c_array[size][size] = {
{1, 2, 3, 4}, {5, 6, 7, 8}, {9, 10, 11, 12}, {13, 14, 15, 16}};
cout << "input array\n";
for (int i = 0; i < size; ++i) {
cout << " [ ";
for (int j = 0; j < size; ++j) {
cout << setw(2) << c_array[i][j] << ", ";
}
cout << "]" << endl;
}
MultiplyArrayByTwo(c_array, size);
cout << "output array\n";
for (int i = 0; i < size; ++i) {
cout << " [ ";
for (int j = 0; j < size; ++j) {
cout << setw(2) << c_array[i][j] << ", ";
}
cout << "]" << endl;
}
cout << endl;
return EXIT_SUCCESS;
}
Production:
input array
[ 1, 2, 3, 4, ]
[ 5, 6, 7, 8, ]
[ 9, 10, 11, 12, ]
[ 13, 14, 15, 16, ]
output array
[ 2, 4, 6, 8, ]
[ 10, 12, 14, 16, ]
[ 18, 20, 22, 24, ]
[ 26, 28, 30, 32, ]
Utiliser la notation &
pour passer un tableau 2D comme paramètre de fonction
Vous pouvez également utiliser la notation de référence &
pour passer un tableau 2D en paramètre. Attention cependant, ces 2 méthodes ne sont compatibles qu’avec les tableaux de longueur fixe déclarés sur la pile. Notez que nous avons modifié la boucle de la fonction MultiplyArrayByTwo
avec une fonction basée sur la portée uniquement pour des raisons de lisibilité.
#include <iomanip>
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::setw;
using std::string;
using std::vector;
constexpr int size = 4;
void MultiplyArrayByTwo(int (&arr)[size][size]) {
for (auto& i : arr) {
for (int& j : i) j *= 2;
}
}
int main() {
int c_array[size][size] = {
{1, 2, 3, 4}, {5, 6, 7, 8}, {9, 10, 11, 12}, {13, 14, 15, 16}};
cout << "input array\n";
for (int i = 0; i < size; ++i) {
cout << " [ ";
for (int j = 0; j < size; ++j) {
cout << setw(2) << c_array[i][j] << ", ";
}
cout << "]" << endl;
}
MultiplyArrayByTwo(c_array);
cout << "output array\n";
for (int i = 0; i < size; ++i) {
cout << " [ ";
for (int j = 0; j < size; ++j) {
cout << setw(2) << c_array[i][j] << ", ";
}
cout << "]" << endl;
}
cout << endl;
return EXIT_SUCCESS;
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook