How to Convert String to Int in C++
-
Use
std::stoi
Method to Convert String to Int in C++ -
Use
std::from_chars
Method to Convert String to Int in C++
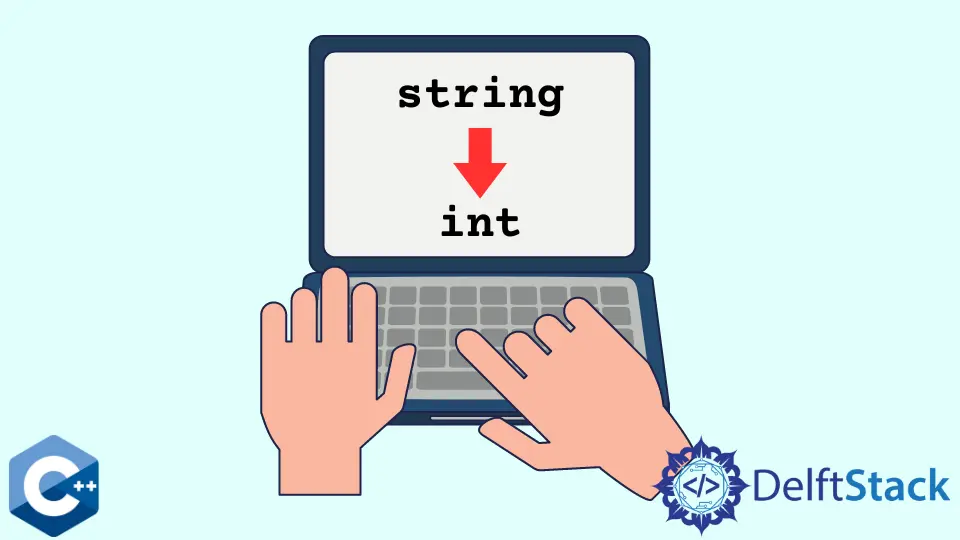
This article introduces multiple methods for converting string
to int
in C++.
Use std::stoi
Method to Convert String to Int in C++
stoi
method is a built-in string
container feature for converting to a signed integer. The method takes one mandatory parameter of the type string
to operate on. We could also pass some optional parameters like the starting position in the string and the number base. The following code sample demonstrates multiple input string
scenarios and corresponding stoi
use cases.
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl using std::string;
using std::stoi;
int main() {
string s1 = "123";
string s2 = "-123";
string s3 = "123xyz";
string s4 = "-123xyz";
string s5 = "7B";
string s6 = "-7B";
string s7 = "1111011";
string s8 = "-1111011";
int num1, num2, num3, num4, num5, num6, num7, num8;
num1 = stoi(s1);
num2 = stoi(s2);
num3 = stoi(s3);
num4 = stoi(s4);
num5 = stoi(s5, nullptr, 16);
num6 = stoi(s6, nullptr, 16);
num7 = stoi(s7, nullptr, 2);
num8 = stoi(s8, nullptr, 2);
cout << "num1: " << num1 << " | num2: " << num2 << endl;
cout << "num3: " << num3 << " | num4: " << num4 << endl;
cout << "num5: " << num5 << " | num6: " << num6 << endl;
cout << "num7: " << num7 << " | num8: " << num8 << endl;
return EXIT_SUCCESS;
}
Output:
num1: 123 | num2: -123
num3: 123 | num4: -123
num5: 123 | num6: -123
num7: 123 | num8: -123
Notice that stoi
can handle leading whitespace characters, +/-
signs, hexadecimal prefixes(0x
or 0X
), even multiple zeros and return the integer correctly. It can’t handle other characters before the digits, and if it finds one, the exception std::invalid_argument
is thrown. You can see the behavior with the following code sample:
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl using std::string;
using std::stoi;
int main() {
string s1 = " 123";
;
string s2 = "xyz123";
;
int num1, num2;
num1 = stoi(s1);
cout << "num1: " << num1 << endl;
try {
num2 = stoi(s2);
cout << "num2: " << num2 << endl;
} catch (std::exception& e) {
cout << e.what() << " caused the error!!!\n";
}
return EXIT_SUCCESS;
}
Output:
num1: 123
stoi caused the error!!!
There are multiple stoi
style methods to convert to different number types like: std::stoi, std::stol, std::stoll
, std::stoul, std::stoull
, std::stof, std::stod, std::stold
which can be utilized to implement specific functionality as needed.
Use std::from_chars
Method to Convert String to Int in C++
from_chars
method is a C++17 addition to the utilities
library, defined in the <charconv>
header file. It can analyze character sequences with specified patterns. The benefit of this is much faster processing time and better handling of exceptions in failed parsing cases. Although there are some restrictions on the input string, namely: leading whitespaces/other characters, even base 16 0x
/0X
prefixes can not be handled. Only the leading minus sign is recognized for signed integers.
Syntax of std::from_chars
from_chars(const char* first, const char* last, TYPE& value, int base = 10)
Parameter | Description |
---|---|
[first, last) |
character address range to parse |
value |
the variable of the parsed result if successful |
base |
int base. Default is 10 |
#include <charconv>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl using std::string;
using std::from_chars;
using std::stoi;
int main() {
string s1 = "123";
string s2 = "-123";
string s3 = "123xyz";
string s4 = "-123xyz";
string s5 = "7B";
string s6 = "-7B";
string s7 = "1111011";
string s8 = "-1111011";
string s9 = " 123";
string s10 = "x123";
int num1, num2, num3, num4, num5, num6, num7, num8, num9, num10;
from_chars(s1.c_str(), s1.c_str() + s1.length(), num1);
from_chars(s2.c_str(), s2.c_str() + s2.length(), num2);
from_chars(s3.c_str(), s3.c_str() + s3.length(), num3);
from_chars(s4.c_str(), s4.c_str() + s4.length(), num4);
from_chars(s5.c_str(), s5.c_str() + s5.length(), num5, 16);
from_chars(s6.c_str(), s6.c_str() + s6.length(), num6, 16);
from_chars(s7.c_str(), s7.c_str() + s7.length(), num7, 2);
from_chars(s8.c_str(), s8.c_str() + s8.length(), num8, 2);
from_chars(s9.c_str(), s9.c_str() + s9.length(), num9);
from_chars(s10.c_str(), s10.c_str() + s10.length(), num10);
cout << "num1: " << num1 << " | num2: " << num2 << endl;
cout << "num3: " << num3 << " | num4: " << num4 << endl;
cout << "num5: " << num5 << " | num6: " << num6 << endl;
cout << "num7: " << num7 << " | num8: " << num8 << endl;
cout << "num9: " << num9 << " | num10: " << num10 << endl;
return EXIT_SUCCESS;
}
Output:
num1: 123 | num2: -123
num3: 123 | num4: -123
num5: 123 | num6: -123
num7: 123 | num8: -123
num9: -16777216 | num10: -1 // incorrect values varying over runs
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookRelated Article - C++ String
- How to Capitalize First Letter of a String in C++
- How to Find the Longest Common Substring in C++
- How to Find the First Repeating Character in a String in C++
- How to Compare String and Character in C++
- How to Get the Last Character From a String in C++
- How to Remove Last Character From a String in C++