How to Get the Last Character From a String in C++
-
Use
std::string::at
to Get the Last Character From a String in C++ -
Use
std::string::back()
to Get the Last Character From a String in C++ -
Use
std::string::rbegin()
to Get the Last Character From a String in C++ -
Use
std::string:begin()
andreverse()
to Get the Last Character From a String in C++ -
Use
std::string::substr()
to Get the Last Character From a String in C++ - Use Array Indexing to Access the Characters of a String in C++
- Conclusion
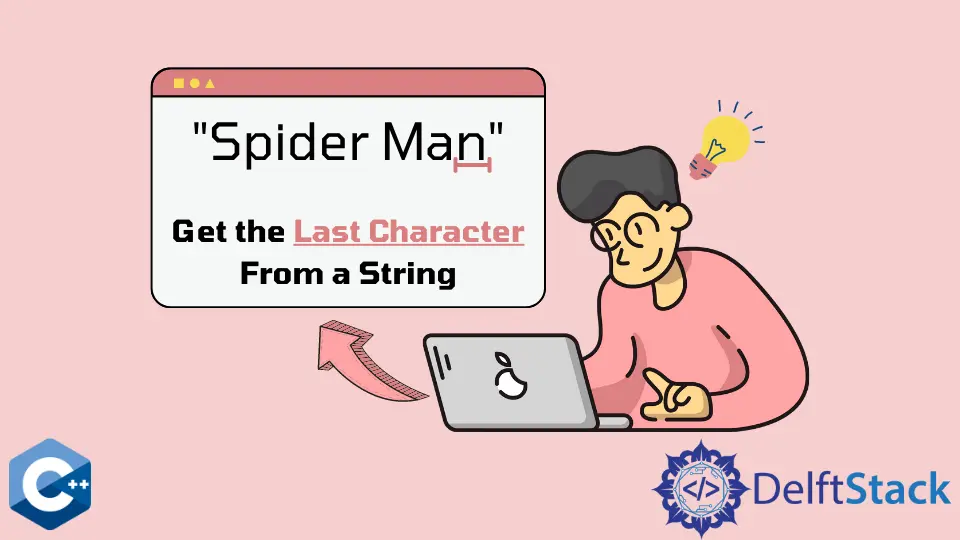
In C++, retrieving the last character from a string is a common task in various programming scenarios. This article explores different approaches to accomplish this task efficiently.
Use std::string::at
to Get the Last Character From a String in C++
We can use std::string::at
to extract a character present at a given position from a string.
Syntax:
char& string::at(size_type indexNo)
It returns the character at the specified position, denoted by indexNo
. However, it throws an out_of_range
exception if an invalid index is provided, such as an index less than zero or greater than or equal to string.size()
.
Example Code:
#include <iostream>
using namespace std;
int main() {
// Create a string named 'str' with the value "Spider Man"
string str("Spider Man");
// Output: Character at position 3: d
cout << "Character at position 3: " << str.at(3) << endl;
// Get the length of the string
int length = str.size();
// Output: Character at last position: n
// Use 'length - 1' as the index to get the last character
cout << "Character at last position: " << str.at(length - 1) << endl;
}
length-1
as the argument to std::string::at()
.Output:
Character at position 3: d
Character at last position: n
This code creates a string "Spider Man"
and then uses std::string::at()
to retrieve and print the character at position 3
(zero-based index) and the last character in the string.
Use std::string::back()
to Get the Last Character From a String in C++
In C++, std::string::back()
provides a reference to the last character of a string, but this works only for non-empty strings. It can also be used to replace the last character.
Syntax:
To access the last character:
char ch = string_name.back();
To replace the character present at the end of the string:
str.back() = 'new_char_we_to_replace_with';
This function takes no parameters and exhibits undefined behavior when applied to an empty string.
Example Code:
#include <iostream>
using namespace std;
int main() {
// Create a string named 'str' with the value "Spider Man"
string str("Spider Man");
// Use 'std::string::back()' to get the last character and store it in 'ch'
char ch = str.back();
// Output: Character at the end: n
cout << "Character at the end: " << ch << endl;
// Use 'std::string::back()' to replace the last character with '@'
str.back() = '@';
// Output: Replace the last character: Spider Ma@
cout << "Replace the last character: " << str << endl;
}
Output:
Character at the end: n
Replace the last character: Spider Ma@
In this code, std::string::back()
is used to retrieve and store the last character 'n'
in the variable 'ch'
in the string. Then, the same function is used to replace the last character with '@'
, resulting in "Spider Ma@"
as the new string.
Use std::string::rbegin()
to Get the Last Character From a String in C++
In C++, std::string::rbegin()
is short for “reverse beginning”. It provides a reverse iterator that points directly to the last character of the string, making it a convenient way to access that final character.
Syntax:
reverse_iterator it = string_name.rbegin();
This function doesn’t require any input parameters and provides a reverse iterator that points to the final character of the string.
Example Code:
#include <iostream>
#include <string>
using namespace std;
int main() {
// Create a string named 'str' with the value "Spider Man"
string str("Spider Man");
// Create a reverse iterator 'it' pointing to the last character of 'str'
string::reverse_iterator it = str.rbegin();
// Output: The last character of the string is: n
cout << "The last character of the string is: " << *it;
}
Output:
The last character of the string is: n
A reverse iterator 'it'
is initialized using std::string::rbegin()
, which points to the last character 'n'
in the string. Finally, the value pointed to by 'it'
(which is the last character) is printed to the console.
Use std::string:begin()
and reverse()
to Get the Last Character From a String in C++
By combining std::string::begin()
and the std::reverse
function, you can effectively access the last character of a string. This method involves reversing the string and then using begin()
to access the formerly last character.
std::string::begin()
in C++ returns the iterator to the beginning of the string, taking no parameter.
Syntax:
string::iterator it = string_name.begin();
std::string::reverse()
is a built-in function in C++ to directly reverse a string. It takes begin
and end
iterators as arguments.
Syntax:
reverse(string_name.begin(), string_name.end());
To get the last character from the string, reverse the string using the reverse()
method and then use begin()
to access the string’s first character (which was the last character before reversal).
Example Code:
#include <algorithm>
#include <iostream>
#include <string>
using namespace std;
int main() {
// Create a string named 'str' with the value "Spider Man"
string str("Spider Man");
// Reverse the string using 'std::reverse'
reverse(str.begin(), str.end());
// Create an iterator 'it' pointing to the first character (now the last) of
// the reversed 'str'
string::iterator it = str.begin();
// Output: The last character of the string is: n
cout << "The last character of the string is: " << *it;
}
Output:
The last character of the string is: n
In this code, the std::reverse
function is used to reverse the characters in the string, making 'n'
the first character. Then, an iterator 'it'
is initialized to point to the first character (which was the last character before the reversal).
Finally, the value pointed to by 'it'
(which is the last character) is printed to the console.
Use std::string::substr()
to Get the Last Character From a String in C++
Another way to extract the last character is by using std::string::substr()
. You can create a substring starting from the last character’s position and with a length of 1
.
Example Code:
#include <iostream>
using namespace std;
int main() {
// Create a string 'str' with the value "Spider Man"
string str("Spider Man");
// Use 'std::string::substr()' to extract the last character
// 'str.length() - 1' calculates the index of the last character
// '1' specifies the length of the substring, which is 1 character
string lastCharacter = str.substr(str.length() - 1, 1);
// Output the extracted last character
cout << "Last character extracted: " << lastCharacter << endl;
}
Output:
Last character extracted: n
In this code, we use std::string::substr()
to extract the last character. The starting position for the substring is calculated as str.length() - 1
, which is the index of the last character in the string.
We specify a length of 1
to extract only one character, and the result is stored in the lastCharacter
variable. Finally, we print the extracted last character, which in this case is "n"
.
Use Array Indexing to Access the Characters of a String in C++
You can access the characters of a string using array indexing by specifying the index number within square brackets []
.
Example Code:
#include <iostream>
using namespace std;
int main() {
// Create a string named 'str' with the value "Spider Man"
string str("Spider Man");
// Get the length (number of characters) of the string
int length = str.size();
// Output: The last character of the string is n
// Use 'length - 1' as the index to access the last character
cout << "The last character of the string is " << str[length - 1];
}
To retrieve the last character, find the length of the string and access the character using length-1
as the index.
Output:
The last character of the string is n
In this code, the std::string::size()
function is used to get the length (number of characters) of the string. Then, the last character is accessed using length - 1
as the index, and it’s printed to the console, resulting in "n"
as the output.
Conclusion
This article has explored multiple methods for extracting the last character from a C++ string. Each method has its advantages and is suited to different coding scenarios.
Whether you prioritize precision, simplicity, elegance, or efficiency, you now have a variety of techniques to choose from. By utilizing these methods, you’ll boost your string manipulation skills and create more efficient C++ applications.