C++의 문자열에서 마지막 문자 가져오기
-
std::string::at
을 사용하여 C++의 문자열에서 마지막 문자 가져오기 -
std::string::back()
을 사용하여 C++의 문자열에서 마지막 문자 가져오기 -
std::string::rbegin()
을 사용하여 C++의 문자열에서 마지막 문자 가져오기 -
std::string:begin()
및reverse()
를 사용하여 C++의 문자열에서 마지막 문자 가져오기 - 배열 인덱싱을 사용하여 C++에서 문자열의 문자에 액세스
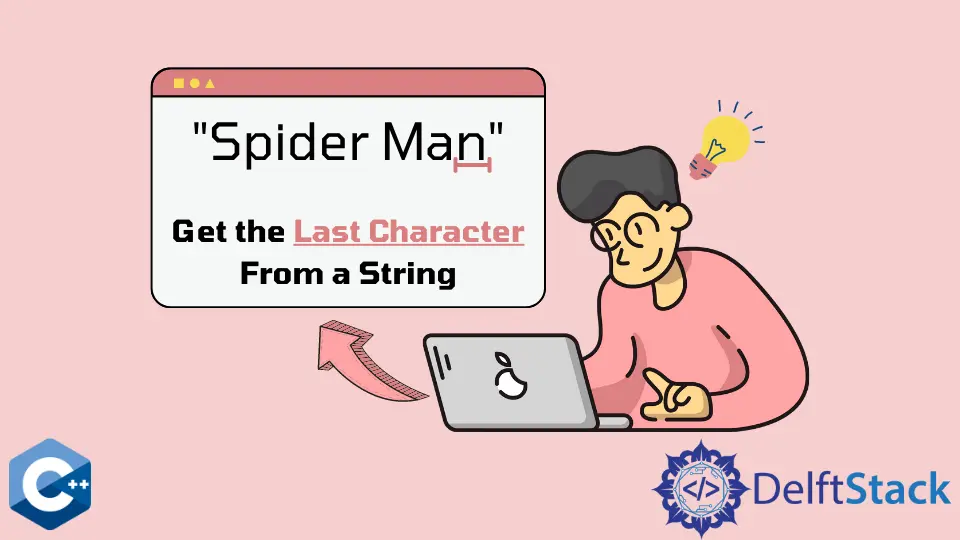
이 기사에서는 C++의 문자열에서 마지막 문자를 가져오는 다양한 방법을 설명합니다.
std::string::at
을 사용하여 C++의 문자열에서 마지막 문자 가져오기
std::string::at
을 사용하여 문자열에서 주어진 위치에 있는 문자를 추출할 수 있습니다.
통사론:
char& string::at(size_type indexNo)
이것은 사용자가 지정한 indexNo
의 지정된 위치에 있는 문자를 반환합니다. 0보다 작은 인덱스 또는 string.size()
보다 크거나 같은 인덱스와 같이 유효하지 않은 인덱스 번호가 전달되면 메서드에서 out_of_range
예외가 발생합니다.
예: 주어진 위치에서 마지막 문자와 문자 추출.
#include <iostream>
using namespace std;
int main() {
string str("Spider Man");
cout << "Character at position 3: " << str.at(3) << endl;
int length = str.size();
cout << "Character at last position: " << str.at(length - 1) << endl;
}
std::string::at()
메서드에서 length-1
을 전달합니다.출력:
Character at position 3: d
Character at last position: n
std::string::back()
을 사용하여 C++의 문자열에서 마지막 문자 가져오기
C++의 std::string::back()
은 문자열의 마지막 문자에 대한 참조를 제공합니다. 이것은 비어 있지 않은 문자열에서만 작동합니다.
이 함수는 문자열 끝에 있는 문자를 바꿀 수도 있습니다.
통사론:
-
마지막 문자에 액세스합니다.
char ch = string_name.back();
-
문자열 끝에 있는 문자를 대체합니다.
```c++
str.back() = 'new_char_we_to_replace_with';
```
이 함수는 매개변수를 사용하지 않으며 문자열의 마지막 문자에 대한 참조를 제공합니다. 빈 문자열에 적용될 때 정의되지 않은 동작을 보여줍니다.
예제 코드:
#include <iostream>
using namespace std;
int main() {
string str("Spider Man");
char ch = str.back();
cout << "Character at the end " << ch << endl;
str.back() = '@';
cout << "String after replacing the last character: " << str << endl;
}
출력:
Character at the end n
String after replacing the last character: Spider Ma@
std::string::rbegin()
을 사용하여 C++의 문자열에서 마지막 문자 가져오기
C++의 std::string::rbegin()
은 역 시작을 나타냅니다. 이 함수는 문자열의 마지막 문자를 가리키는 데 사용됩니다.
통사론:
reverse_iterator it = string_name.rbegin();
이 함수는 매개변수를 포함하지 않으며 문자열의 마지막 문자를 가리키는 역방향 반복자를 반환합니다.
예제 코드:
#include <iostream>
#include <string>
using namespace std;
int main() {
string str("Spider Man");
string::reverse_iterator it = str.rbegin();
cout << "The last character of the string is: " << *it;
}
출력:
The last character of the string is: n
std::string:begin()
및 reverse()
를 사용하여 C++의 문자열에서 마지막 문자 가져오기
C++의 std::string::begin()
은 매개변수를 사용하지 않고 문자열의 시작 부분으로 반복자를 반환합니다.
통사론:
string::iterator it = string_name.begin();
std::string::reverse()
는 문자열을 직접 반전시키는 C++의 내장 함수입니다. begin
및 end
반복자를 인수로 사용합니다.
이 기능은 algorithm
헤더 파일에 정의되어 있습니다.
통사론:
reverse(string_name.begin(), string_name.end());
문자열에서 마지막 문자를 가져오려면 먼저 reverse
메서드를 사용하여 문자열을 뒤집은 다음 begin()
메서드를 사용하여 문자열의 첫 번째 문자(역전 이전의 마지막 문자)에 대한 참조를 가져오고 다음을 인쇄합니다. 그것.
예제 코드:
#include <algorithm>
#include <iostream>
#include <string>
using namespace std;
int main() {
string str("Spider Man");
reverse(str.begin(), str.end());
string::iterator it = str.begin();
cout << "The last character of the string is: " << *it;
}
출력:
The last character of the string is: n
배열 인덱싱을 사용하여 C++에서 문자열의 문자에 액세스
인덱스 번호를 사용하여 문자열을 참조하고 대괄호 []
안에 전달하여 문자열의 문자에 액세스할 수 있습니다.
예제 코드:
#include <iostream>
using namespace std;
int main() {
string str("Bruce banner");
int length = str.size();
cout << "The last character of the string is " << str[length - 1];
}
마지막 문자를 얻으려면 먼저 문자열의 길이를 찾고 대괄호 안에 length-1
을 전달합니다.
출력:
The last character of the string is r