从 C++ 中的字符串中获取最后一个字符
Suraj P
2023年10月12日
C++
C++ String
-
在 C++ 中使用
std::string::at
从字符串中获取最后一个字符 -
在 C++ 中使用
std::string::back()
从字符串中获取最后一个字符 -
使用
std::string::rbegin()
从 C++ 中的字符串中获取最后一个字符 -
在 C++ 中使用
std::string:begin()
和reverse()
从字符串中获取最后一个字符 - 在 C++ 中使用数组索引访问字符串的字符
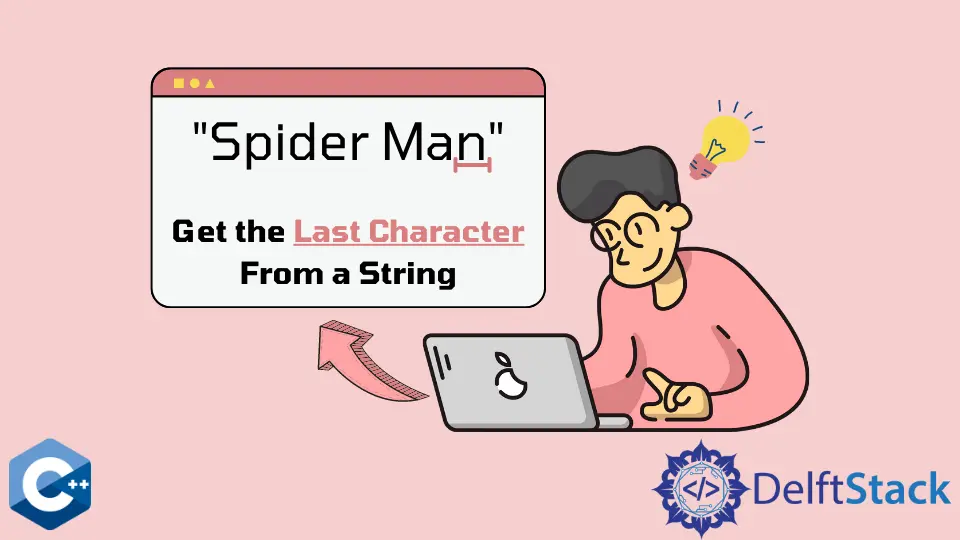
本文将教授 C++ 中从字符串中获取最后一个字符的不同方法。
在 C++ 中使用 std::string::at
从字符串中获取最后一个字符
我们可以使用 std::string::at
从字符串中提取出现在给定位置的字符。
语法:
char& string::at(size_type indexNo)
这将返回用户指定的 indexNo
处指定位置的字符。当传递了无效的索引号时,该方法会抛出 out_of_range
异常,例如小于零的索引或大于或等于 string.size()
的索引。
示例:提取最后一个字符和给定位置的字符。
#include <iostream>
using namespace std;
int main() {
string str("Spider Man");
cout << "Character at position 3: " << str.at(3) << endl;
int length = str.size();
cout << "Character at last position: " << str.at(length - 1) << endl;
}
解释
为了得到最后一个位置的字符,我们首先找到字符串的长度,并在
std::string::at()
方法中传递 length-1
。输出:
Character at position 3: d
Character at last position: n
在 C++ 中使用 std::string::back()
从字符串中获取最后一个字符
C++ 中的 std::string::back()
给出了对字符串最后一个字符的引用。这仅适用于非空字符串。
此函数还可以替换字符串末尾的字符。
语法:
- 访问最后一个字符。
char ch = string_name.back();
- 替换出现在字符串末尾的字符。
str.back() = 'new_char_we_to_replace_with';
此函数不带参数,它提供对字符串最后一个字符的引用。当应用于空字符串时,它会显示未定义的行为。
示例代码:
#include <iostream>
using namespace std;
int main() {
string str("Spider Man");
char ch = str.back();
cout << "Character at the end " << ch << endl;
str.back() = '@';
cout << "String after replacing the last character: " << str << endl;
}
输出:
Character at the end n
String after replacing the last character: Spider Ma@
使用 std::string::rbegin()
从 C++ 中的字符串中获取最后一个字符
C++ 中的 std::string::rbegin()
代表反向开始。该函数用于指向字符串的最后一个字符。
语法:
reverse_iterator it = string_name.rbegin();
该函数不包含任何参数,它返回指向字符串最后一个字符的反向迭代器。
示例代码:
#include <iostream>
#include <string>
using namespace std;
int main() {
string str("Spider Man");
string::reverse_iterator it = str.rbegin();
cout << "The last character of the string is: " << *it;
}
输出:
The last character of the string is: n
在 C++ 中使用 std::string:begin()
和 reverse()
从字符串中获取最后一个字符
C++ 中的 std::string::begin()
将迭代器返回到字符串的开头,不带参数。
语法:
string::iterator it = string_name.begin();
std::string::reverse()
是 C++ 中用于直接反转字符串的内置函数。它将 begin
和 end
迭代器作为参数。
该函数在 algorithm
头文件中定义。
语法:
reverse(string_name.begin(), string_name.end());
要从字符串中获取最后一个字符,我们首先使用 reverse
方法反转字符串,然后使用 begin()
方法获取字符串第一个字符的引用(这是反转之前的最后一个字符)并打印它。
示例代码:
#include <algorithm>
#include <iostream>
#include <string>
using namespace std;
int main() {
string str("Spider Man");
reverse(str.begin(), str.end());
string::iterator it = str.begin();
cout << "The last character of the string is: " << *it;
}
输出:
The last character of the string is: n
在 C++ 中使用数组索引访问字符串的字符
我们可以通过使用它的索引号引用它并在方括号 []
中传递它来访问字符串的字符。
示例代码:
#include <iostream>
using namespace std;
int main() {
string str("Bruce banner");
int length = str.size();
cout << "The last character of the string is " << str[length - 1];
}
为了得到最后一个字符,我们首先找到字符串的长度并在方括号中传递 length-1
。
输出:
The last character of the string is r
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Suraj P