How to Capitalize First Letter of a String in C++
- Capitalize the First Letter of a String in C++
- String Contains Alphabet as a First Character
- String’s First Letter is a Special Character
- String With Multiple Words Containing Multibyte Starting Characters
- Conclusion
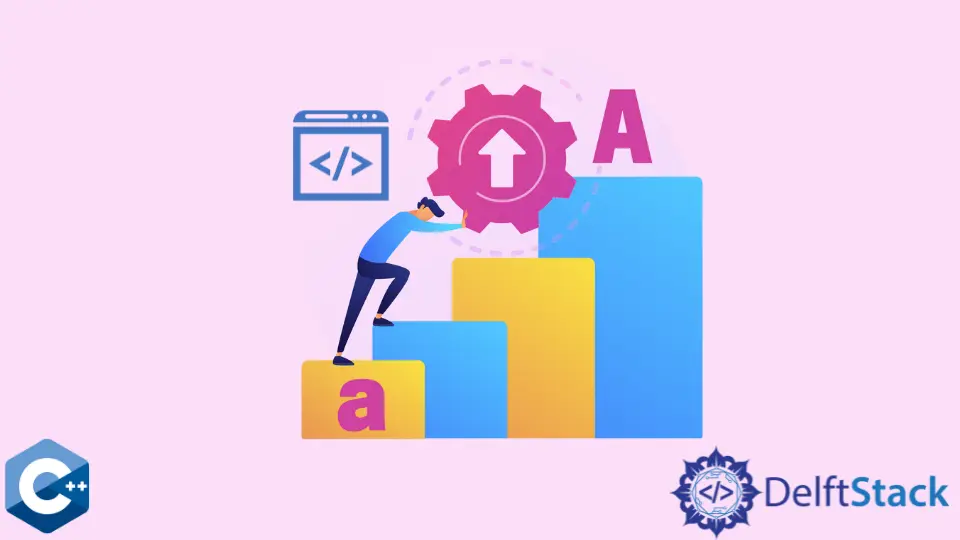
In this article, we explore various ways to capitalize the first letter of a string in C++. Addressing different cases, we tackle scenarios with the aim of capitalizing each word.
Capitalize the First Letter of a String in C++
We will deal with this problem in three different cases:
-
The string begins with an alphabet.
-
The string starts with a special character or a number.
-
The string contains a multibyte starting character, and we need to capitalize each word.
String Contains Alphabet as a First Character
Solutions to this case are straightforward. We can either use the built-in toupper()
library function or can use our user-defined implementation.
We have a method called toupper()
in C++, and this method converts a lowercase alphabet into uppercase. The goal can be achieved by simply calling this function on the string’s initial index.
In the code below, we declare a string variable named str
with the initial value "sudo"
. The objective is to capitalize the first letter of the string.
We achieve this by utilizing the toupper()
function, which converts a lowercase alphabet to uppercase. Specifically, we apply this function to the first character of the string (str[0]
), effectively transforming it to uppercase.
The output of this code will be "Sudo"
, demonstrating the successful capitalization of the initial letter of the string.
Example Code:
#include <iostream>
#include <string>
using namespace std;
int main() {
string str = "sudo";
str[0] = toupper(str[0]);
cout << str;
}
Output:
Sudo
It is always wise to be familiar with the logic behind built-in methods. Let’s look for a solution without using the built-in one.
Lowercase characters from a
to z
have an ASCII value between 97
and 122
, and uppercase characters from A
to Z
have an ASCII value between 65
and 92
. We deduct 32
from the input char’s ASCII value for conversion from lower to upper case.
It is always good to check whether the first character is already an uppercase letter. Otherwise, we may have to face a strange output.
Example Code:
#include <iostream>
#include <string>
using namespace std;
int main() {
string ML_Model = "artificial neural networks";
if (ML_Model[0] >= 97 && ML_Model[0] <= 122) {
ML_Model[0] = ML_Model[0] - 32;
}
cout << ML_Model;
}
Output:
Artificial neural networks
In the code above, we initialize a string variable named ML_Model
with the value "artificial neural networks"
. The objective is to capitalize the first letter of the string if it is initially a lowercase character.
We achieve this by using an if
statement that checks whether the ASCII value of the first character in the string (accessed with ML_Model[0]
) falls within the range of lowercase letters (between 97
and 122
, inclusive). If the condition is true, indicating that the first character is a lowercase letter, we perform the conversion to uppercase by subtracting 32
from its ASCII value.
Finally, we output the modified string using cout
. In the code example, the output will be "Artificial neural networks"
, showcasing the successful capitalization of the initial letter.
String’s First Letter is a Special Character
We will now discuss a case where there can be some special characters or numbers at the start of the string. In that case, subtracting 32
from the first letter can generate a strange symbol.
To handle such types of strings, we will first use the isalpha
method to determine whether or not it is an alphabet. And after this method, we will use the toupper
function on a character.
Example Code:
#include <iostream>
using namespace std;
void upperCaseAlphabet(string &str) {
for (int i = 0; i <= str.length(); i++) {
if (isalpha(str[i])) {
str[i] = toupper(str[i]);
break;
}
}
}
int main() {
string str = "#1delftstack";
upperCaseAlphabet(str);
cout << str;
}
Output:
#1Delftstack
In this code, we defined the upperCaseAlphabet
function that takes a string reference as a parameter. The purpose of this function is to capitalize the first alphabet character in the given string.
We achieve this by iterating through the string using a for
loop and, upon encountering the first alphabet character (determined by the isalpha()
function), we use toupper()
to convert it to uppercase. Importantly, we break out of the loop after this transformation to ensure only the first alphabet character is capitalized.
In the main
function, we initialize a string variable str
with the value "#1delftstack"
, and then we invoke the upperCaseAlphabet
function, modifying the string in place. Finally, we use cout
to display the updated string.
The output of this code will be "#1Delftstack"
, showcasing the successful capitalization of the first alphabet character in the string.
String With Multiple Words Containing Multibyte Starting Characters
In previous examples, we were dealing with strings containing only one word. Let’s now work with longer strings containing more words and special characters and capitalize each word of the string.
We’ll use toupper()
, isalpha()
and isspace()
methods to achieve the goal.
-
toupper()
- converts an alphabet character to uppercase. -
isalpha()
- checks if the character is an alphabet. -
isspace()
- checks if the character is a space.
Example Code:
#include <iostream>
#include <string>
using namespace std;
void upperCaseAlphabet(string &str) {
bool flag = true;
for (int i = 0; i <= str.length(); i++) {
if (isalpha(str[i]) && flag == true) {
str[i] = toupper(str[i]);
flag = false;
} else if (isspace(str[i])) {
flag = true;
}
}
}
int main() {
string str = "i $love #Traveling";
upperCaseAlphabet(str);
cout << str;
}
Output:
I $Love #Traveling
In this code, we define a function called upperCaseAlphabet
that takes a string reference as a parameter. The goal of this function is to capitalize the first alphabet character of each word in the given string while accounting for special characters and spaces.
Then, we use a Boolean flag to keep track of whether we’re processing the first character of a word. In the main function, we initialize a string variable str
with the value "i $love #Traveling"
, and then we invoke the upperCaseAlphabet
function, which modifies the string in place.
Next, the for
loop iterates through each character, and if the character is an alphabet character and the flag
is true,
we capitalize it using toupper()
. Additionally, if space is encountered, we set the flag
to true
, signifying the start of a new word.
The output of this code will be "I $Love #Traveling"
, demonstrating the successful capitalization of the first alphabet character in each word of the string.
Conclusion
We’ve looked at different ways to make the first letter of a word fancy in C++. We started with regular words, showing how to make the first letter big using the built-in toupper()
method. For example, in "Sudo"
, we used the toupper()
method to make the 'S'
stand out.
Next, we tackled words that start with numbers or symbols, and we made a helper function called upperCaseAlphabet
to handle this. For instance, in "#1Delftstack"
, our function made sure the 'D'
in Delftstack
was big, no matter what came before it.
Lastly, we took on the challenge of making every first letter big in sentences with many words and different characters. We mixed a few methods like toupper()
, isalpha()
, and isspace()
to get the job done, and the result, seen in "I $Love #Traveling"
, showed how our approach works smoothly with all kinds of words and spaces.
In a nutshell, these methods give C++ developers useful tools to play around with words and make them look how they want, whether it’s making a single letter pop or styling entire sentences.