How to Remove Last Character From a String in C++
-
Remove the Last Character in a String Using the
pop_back()
Function -
Remove the Last Character in a String Using the
erase()
Function -
Remove the Last Character From a String Using the
substr()
Function -
Remove the Last Character From a String Using the
resize()
Function - Remove the Last Character From a String Using Index Access
- Conclusion
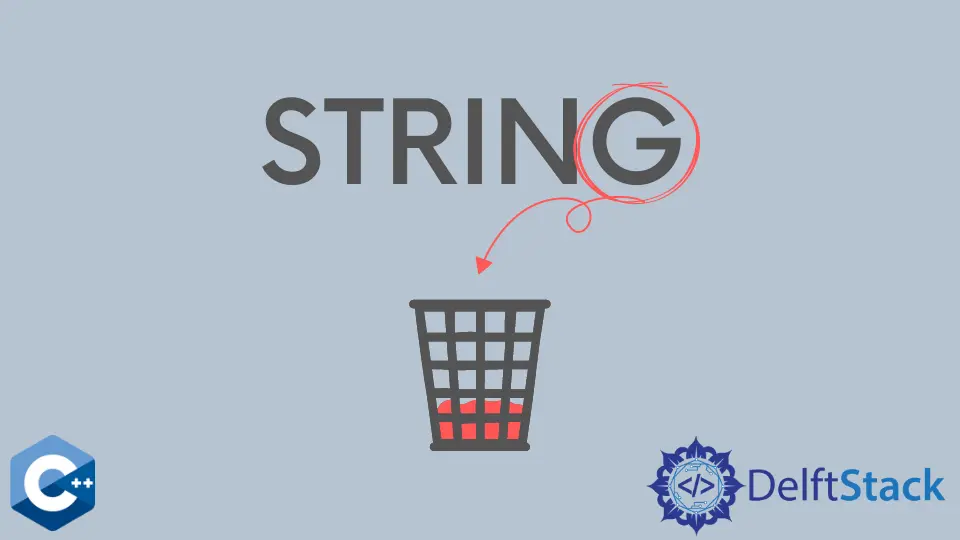
String manipulation is a fundamental operation in C++, especially when dealing with text. In C++, you can declare strings in two primary ways: as an array of characters or using the standard string class.
However, removing the last character from an array of characters can be tricky due to its fixed size and non-instantiated nature. Fortunately, C++ provides built-in functions within the string class to make this task easier.
In this article, we’ll explore different methods for removing the last character from a C++ string, including pop_back()
, erase()
, substr()
, resize()
, and index access
.
Remove the Last Character in a String Using the pop_back()
Function
The pop_back()
is a built-in function in C++ STL that removes the last element from a string. It simply deletes the last element and adjusts the length of the string accordingly.
The syntax below your_string.pop_back();
operates on a string variable named your_string
. It uses the pop_back()
member function of the std::string
class to remove the last character from the string.
Syntax:
your_string.pop_back();
In this code example, we have a string variable named str
to store user input. We use cin
to read a string from the user, store it in the str
variable, and print the original input string using cout
.
Then, we call the pop_back()
function on the str
variable, which removes the last character from the string. Finally, it prints the modified string after removing the last character.
Code Snippet:
#include <iostream>
using namespace std;
int main() {
string str;
cin >> str;
cout << "Original String: " << str << endl;
str.pop_back();
cout << "Required String: " << str << endl;
return 0;
}
Output:
Original String: Welcome
Required String: Welcom
As you can see, the pop_back()
function effectively removes the last character ('e'
) from the string.
Remove the Last Character in a String Using the erase()
Function
The erase()
method is a built-in method of the string class. This method can delete a single character or a range of characters by specifying their indexes.
There are three different variations in which the erase()
method can be used. We’ll discuss two of them since we only need to remove the last character.
Remove the Last Character in a String Using the erase(pos,Len)
Function
In this function, two parameters are given, and one is the pos
that specifies the character to be removed with its index. The second is the Len
, which tells us the length or the number of characters to be removed from the string.
In the syntax below, your_string.erase(pos, len);
operates on a string object, where your_string
is the name of the string variable. This statement is used to remove a portion of the string specified by the pos
(position) and len
(length) parameters.
Syntax:
your_string.erase(pos, len);
The following code declares a string variable named str
to store user input. It uses cin
to read a string from the user, store it in the str
variable, and print the original input string using cout
.
Then calls the erase()
function on the str
variable to remove the last character from the string. The str.size() - 1
calculates the index of the last character, and 1
specifies that one character should be erased starting from that position.
Lastly, it prints the modified string after removing the last character.
Code Snippet:
#include <iostream>
using namespace std;
int main() {
string str;
cin >> str;
cout << "Original String: " << str << endl;
str.erase(str.size() - 1, 1);
cout << "Required String: " << str << endl;
return 0;
}
Output:
Original String: Hello
Required String: Hell
In the output, the erase(pos, len)
function removes the last character ('o'
) from the string Hello
.
Remove the Last Character in a String Using the erase(iterator)
Function
This function takes only one parameter, an iterator
that points to the character to be removed. Therefore, we’ll pass size()-1
as the parameter since the indexing starts from 0
to size()-1
will point to the last character of the string.
The syntax below, your_string.erase(your_string.end() - 1);
operates on a string object, where your_string
is the name of the string variable. The erase()
is a member function of the std::string
class and is used to remove characters from the string.
The your_string.end()
returns an iterator pointing to the end of the string. In C++, strings are often manipulated using iterators, and your_string.end() - 1
gives you an iterator pointing to the character just before the end of the string (i.e., the last character).
Lastly, the - 1
is used to subtract 1 from the iterator obtained from your_string.end()
, effectively moving it one position back from the end.
Syntax:
your_string.erase(your_string.end() - 1);
In this code example, we declare a string variable named str
to store user input. Then, cin
reads a string from the user and stores it in the str
variable, and prints the original input string using cout
.
Next, it calls the erase()
function on the str
variable to remove the last character from the string. The str.size() - 1
calculates the index of the last character, and the erase()
function removes the character at that index.
Finally, it prints the modified string after removing the last character.
Code Snippet:
#include <iostream>
using namespace std;
int main() {
string str;
cin >> str;
cout << "Original String: " << str << endl;
str.erase(str.size() - 1);
cout << "Required String: " << str << endl;
return 0;
}
Output:
Original String: Hello
Required String: Hell
The str.erase(str.size() - 1)
function removes the last character ('o'
) from the string(Hello
).
Remove the Last Character From a String Using the substr()
Function
The substr()
method returns a substring from the original string. It takes two parameters: the substring’s starting index (0
) and the substring’s length that we want (your_string.size() - 1
).
If you do not provide the starting index, it will return the default value 0
. If the length is not specified, it will take all the characters of the string.
The size()
method gives the length of the string while size()-1
will provide you the length till the second last character.
Syntax:
your_string.substr(0, your_string.size() - 1);
In the code below, we declare a string variable named str
to store user input. Then, we use cin
to read a string from the user, store it in the str
variable, and print the original input string using cout
.
The substr()
function extracts a substring from the original string. The parameters passed to substr()
are (0, str.size() - 1)
, which means it starts from index 0
(the beginning of the string) and goes up to, but doesn’t include, the last character (index str.size() - 1
) and this effectively removes the last character from the string.
Lastly, it prints the modified substring as the "Required String"
.
Code Snippet:
#include <iostream>
using namespace std;
int main() {
string str;
cin >> str;
cout << "Original String: " << str << endl;
cout << "Required String: " << str.substr(0, str.size() - 1) << endl;
return 0;
}
Output:
Original String: Welcome
Required String: Welcom
When you provide the input Welcome
, the program extracts a substring from index 0
to str.size() - 1
, effectively removing the last character (e
).
Remove the Last Character From a String Using the resize()
Function
The resize()
method in C++ modifies a string by adjusting its length, allowing for the insertion or removal of elements. To remove the last character from a string using this method, you must specify the desired length or size of the string.
The syntax your_string.resize(your_string.size() - 1);
operates on a string object, where your_string
is the name of the string variable. This statement is used to resize the string by reducing its length by one character.
The resize
is a member function of the std::string
class. It is used to change the size (length) of the string. The your_string.size()
returns the current size (length) of the string, which is the number of characters in the string, and the - 1
subtracts 1 from the current size of the string to reduce the length of the string by one character.
Syntax:
your_string.resize(your_string.size() - 1);
In the example code, let’s declare a string variable named str
to store user input. Then, we use cin
to read a string from the user, store it in the str
variable, and print the original input string using cout
.
Next, we call the resize()
function on the str
variable to resize the string. The size is reduced by one character using str.size() - 1
, effectively removing the last character from the string.
Finally, it prints the modified string as the "Required String"
.
Code Snippet:
#include <iostream>
using namespace std;
int main() {
string str;
cin >> str;
cout << "Original String: " << str << endl;
str.resize(str.size() - 1);
cout << "Required String: " << str << endl;
return 0;
}
Output:
Original String: Welcome
Required String: Welcom
When you provide the input Welcome
, the program resizes the string by removing the last character (e
), resulting in the output above.
Remove the Last Character From a String Using Index Access
This method removes the last character from a string by directly accessing its characters using index notation.
The syntax below checks if your_string
is not empty and, if so, converts it into a string by replacing its last character with a null character ('\0'
). It first checks if the string is not empty using if (!your_string.empty())
and then modifies the string by assigning '\0'
to its last character using your_string[your_string.size() - 1] = '\0';
.
Syntax:
if (!your_string.empty()) {
your_string[your_string.size() - 1] = '\0';
}
The following code example initializes a string variable named myString
and initializes it with the value "Hello, World!"
.
Then, it enters an if
statement to check if the string myString
is not empty using the empty()
member function. If the string is not empty, it proceeds to the next step; otherwise, it skips the modification.
Inside the if
statement, it accesses the last character of the string using myString.length() - 1
as the index. The length()
member function returns the number of characters in the string, and subtracting 1 gives the index of the last character.
It assigns the null character '\0'
to the last character, effectively terminating the string at that point. This converts the string into a character array by replacing the last character (!
) with a null character.
Finally, it prints the modified string using std::cout
without the last character of the string.
Code Snippet:
#include <iostream>
#include <string>
int main() {
std::string myString = "Hello, World!";
if (!myString.empty()) {
myString[myString.length() - 1] = '\0';
}
std::cout << myString << std::endl;
return 0;
}
Output:
Hello, World
The output prints the modified string "Hello, World"
, effectively removing the last character(!
).
Conclusion
Manipulating strings in C++, including removing the last character, is a common task. We’ve explored various methods to achieve this, providing valuable tools for string manipulation in a wide range of applications.
Depending on your needs, you can use any of the four methods we discussed in this article to remove the last character of a string in C++.