從 C++ 中的字串中刪除最後一個字元
- 從 C++ 中的字串中刪除最後一個字元
-
使用
pop_back()
函式從 C++ 中的字串中刪除最後一個字元 -
使用
erase()
方法從 C++ 中的字串中刪除最後一個字元 -
使用
erase(pos,Len)
函式刪除字串中的最後一個字元 -
使用
erase(iterator)
函式刪除字串中的最後一個字元 -
使用
substr()
方法從字串中刪除最後一個字元 -
使用
resize()
方法從字串中刪除最後一個字元 - まとめ
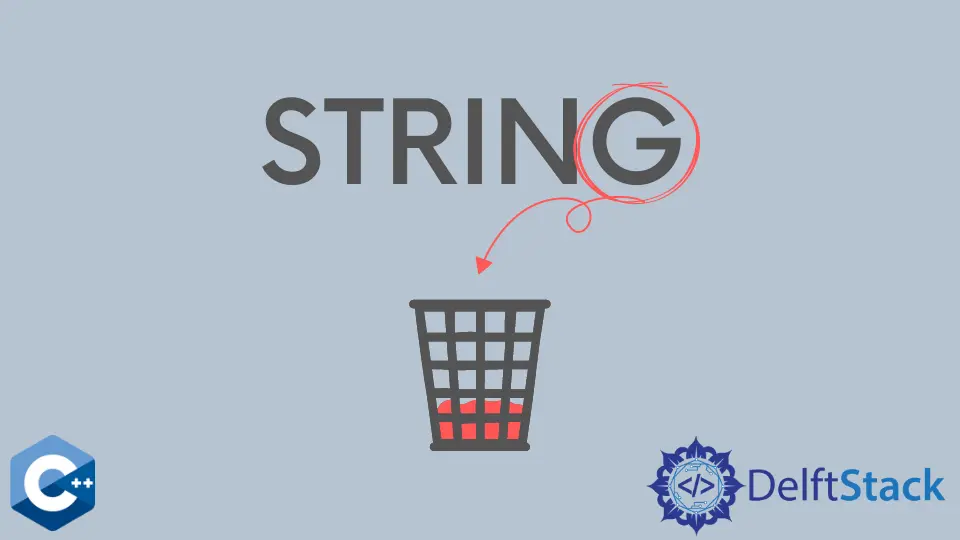
C++ 中的字串用於儲存一系列字元以對其執行不同的操作。在本文中,我們將使用字串並從中刪除最後一個字元。
從 C++ 中的字串中刪除最後一個字元
在 C++ 中,可以通過兩種方式宣告字串:字元陣列或標準字串類。但是,你不能從字元陣列中刪除最後一個元素,因為陣列具有固定大小並且不是例項化物件。
c++ 中的字串類包含幾個內建函式,這些函式在刪除字串的最後一個字元後給出字串。pop_back()
函式和 erase()
函式是其中的兩個。
使用 pop_back()
函式從 C++ 中的字串中刪除最後一個字元
pop_back()
是 C++ STL 中的內建函式,用於從字串中刪除最後一個元素。它只是刪除最後一個元素並相應地調整字串的長度。
程式碼片段:
#include <iostream>
using namespace std;
int main() {
string str;
cin >> str;
cout << "Original String: " << str << endl;
str.pop_back();
cout << "Required String: " << str << endl;
return 0;
}
輸出:
Original String: Welcome
Required String: Welcom
使用 erase()
方法從 C++ 中的字串中刪除最後一個字元
erase()
方法是字串類的內建方法。此方法可以通過指定索引來刪除單個字元或一系列字元。
erase()
方法可以使用三種不同的變體,但我們將討論其中的兩種,因為我們只需要刪除最後一個字元。
使用 erase(pos,Len)
函式刪除字串中的最後一個字元
在這個函式中,給出了兩個引數,一個是 pos
,它指定要刪除的字元及其索引。第二個是 Len
,它告訴我們要從字串中刪除的長度或字元數。
程式碼片段:
#include <iostream>
using namespace std;
int main() {
string str;
cin >> str;
cout << "Original String: " << str << endl;
str.erase(str.size() - 1, 1);
cout << "Required String: " << str << endl;
return 0;
}
輸出:
Original String: Hello
Required String: Hell
使用 erase(iterator)
函式刪除字串中的最後一個字元
這個函式只接受一個引數,一個指向要刪除的字元的 iterator
。因此,我們將 size()-1
作為引數傳遞,因為索引從 0
開始到 size()-1
將指向字串的最後一個字元。
程式碼片段:
#include <iostream>
using namespace std;
int main() {
string str;
cin >> str;
cout << "Original String: " << str << endl;
str.erase(str.size() - 1);
cout << "Required String: " << str << endl;
return 0;
}
輸出:
Original String: Hello
Required String: Hell
使用 substr()
方法從字串中刪除最後一個字元
substr()
方法從原始字串返回一個子字串。它有兩個引數,子字串的起始索引和我們想要的子字串的長度。
如果不提供起始索引,則返回預設值 0
,如果未指定長度,則取字串的所有字元。size()
方法給出字串的長度,而 size()-1
將提供直到倒數第二個字元的長度。
程式碼片段:
#include <iostream>
using namespace std;
int main() {
string str;
cin >> str;
cout << "Original String: " << str << endl;
cout << "Required String: " << str.substr(0, str.size() - 1) << endl;
return 0;
}
輸出:
Original String: Welcome
Required String: Welcom
這個函式的一個重要點是它不會修改原始字串,而是建立一個新字串。
使用 resize()
方法從字串中刪除最後一個字元
resize()
方法調整字串大小並通過在容器中插入或刪除元素來更改內容。由於我們需要將字串的長度減 1,因此,我們可以使用它。
我們需要傳遞我們想要的字串的長度。如前所述,size-1
將通過刪除其最後一個字元來給出字串。
程式碼片段:
#include <iostream>
using namespace std;
int main() {
string str;
cin >> str;
cout << "Original String: " << str << endl;
str.resize(str.size() - 1);
cout << "Required String: " << str << endl;
return 0;
}
輸出:
Original String: Welcome
Required String: Welcom
まとめ
在本教程中,我們討論了四種不同的方法,通過這些方法我們可以從字串中刪除最後一個字元,並使用上述方法顯示程式碼示例。
我們瞭解了 C++ 標準模板庫 (STL) 的 pop_back()
、erase()
和 resize()
和 substr()
方法的語法。