How to Compare String and Character in C++
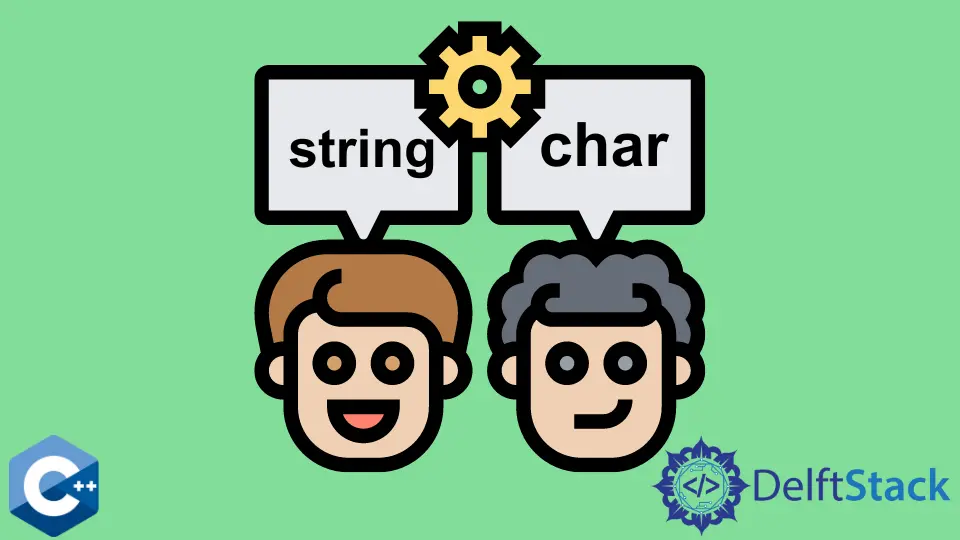
This trivial guide is about using strings in C++ and how these strings are compared with other literals. Before moving further, we will briefly introduce strings in C++.
In C++, strings can be categorized in two different ways:
- Create a
Character
array to form a string - Use the standard
String
library in C++
Create a Character Array in C++
Like in C-language, C++ also provides us with character arrays, i.e., char
array, which can be used as a string literal. It is a one-dimensional array of characters terminated by a null
.
So the string is created by forming a char
array and terminating it with a null
character.
Please note that to print a character array using an aggregate method (e.g., cout<< charArray
), a null
character must terminate the character array. C++ uses \0
as a NULL
character, and it helps aggregate methods to detect the end of the character string (it is impossible to detect the end of an array without some end-marking symbol).
Therefore, the below example declares a character array of size 11
though C-language
contains only ten characters.
char word[11] = {'C', '-', 'l', 'a', 'n', 'g', 'u', 'a', 'g', 'e', '\0'};
The above statement can also be written as the following, provided that you follow the initialization rules.
char word[] = "C-language";
If you forget to put the null
character at the end, the compiler implicitly places the null
character at the end itself. Let’s have a look at the program below.
#include <iostream>
using namespace std;
int main() {
char word[11] = {'C', '-', 'l', 'a', 'n', 'g', 'u', 'a', 'g', 'e', '\0'};
cout << "First message: ";
cout << word << endl;
return 0;
}
Line#06 in the above code snippet will contribute First Message:
to the output, while line#07 will display all the characters in the word
variable until encountering a \0
. Therefore, the above code snippet will generate the following output.
First Message: C-language
Use String
Library in C++
C++ has a built-in string.h
header file as part of its standard library. It provides a bundle of functionalities (e.g., strcpy
, strlen
, etc.) to work with C-style strings (i.e., null-terminated strings).
Please note that all modern string
library included without .h
is different from the string.h
. The string
class library is a C++ library to manipulate modern C++ strings while string.h
is a C header file to manipulate the C-style strings(i.e., null-terminated strings).
Let’s look at the below code to understand the string.h
library.
#include <string.h>
#include <iostream>
using namespace std;
int main() {
char country[] = "Pakistan";
char countryTemp[50] = "abc";
cout << "countryTemp length before initializing is:";
cout << strlen(countryTemp) << endl;
// strcpy()
cout << "Let's copy country to countryTemp" << endl;
strcpy(countryTemp, country);
cout << "countryTemp=" << countryTemp << endl;
cout << "countryTemp length after copying country is:";
cout << strlen(countryTemp) << endl;
return 0;
}
The above program first declares two character arrays (C-strings) and initializes the first one with Pakistan
. Line#10 prints the length of countryTemp
, which is 3.
Though the total size of the countryTemp
is 50 bytes, the strcpy
can only calculate size based on the NULL
character (placed in the fourth byte of the array). Therefore, the strcpy
returns 3.
Line#14 uses the strcpy
function to copy the contents of the country
array to countryTemp
. Therefore, the new length of countryTemp
becomes 8
.
Output:
countryTemp length before initializing is:3
Let's copy country to countryTemp
countryTemp=Pakistan
countryTemp length after copying country is:8
string.h
header file for NULL
terminated strings (also known as C-style strings). More available library functions can be found here.Comparison of Strings with Char in C++
It is a common problem for people to compare strings with character constants. This is practically not possible.
Consider the code below.
#include <iostream>
using namespace std;
int main() {
cout << "Do you want to proceed (y or n)?\n";
char inp;
cin >> inp;
if (inp == "y") // error here
cout << "Hello again" << endl;
else
cout << "Good Bye" << endl;
return 0;
}
Here in this function, we have made an input from the user, which is a char
variable, and in the if
condition, we compare it with a string literal enclosed in double-quotes. This code will generate an error: ISO C++ forbids comparison between pointer and integer
error.
It is recommended to take the input in the string
variable instead of the char
variable to avoid this error.
#include <iostream>
#include <string>
using namespace std;
int main() {
cout << "Do you want to proceed (y or n)?\n";
string ans;
cin >> ans;
if (ans == "y") // error here
cout << "Hello again" << endl;
else
cout << "Good Bye" << endl;
return 0;
}
Output:
Do you want to proceed (y or n)?
n
Good Bye
Husnain is a professional Software Engineer and a researcher who loves to learn, build, write, and teach. Having worked various jobs in the IT industry, he especially enjoys finding ways to express complex ideas in simple ways through his content. In his free time, Husnain unwinds by thinking about tech fiction to solve problems around him.
LinkedIn