C++ での文字列と文字の比較
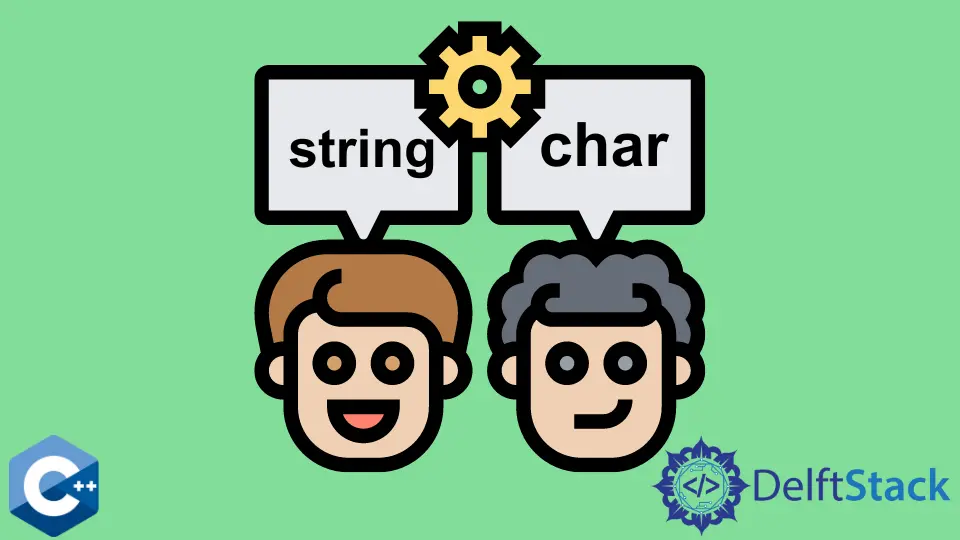
この簡単なガイドでは、C++ での文字列の使用と、これらの文字列を他のリテラルと比較する方法について説明します。先に進む前に、C++ で文字列を簡単に紹介します。
C++ では、文字列は 2つの異なる方法で分類できます。
文字
配列を作成して文字列を形成します- C++ で標準の
String
ライブラリを使用します
C++ で文字配列を作成する
C 言語と同様に、C++ も文字配列、つまり文字列リテラルとして使用できる char
配列を提供します。これは、null
で終了する文字の 1 次元配列です。
したがって、文字列は、char
配列を形成し、それを null
文字で終了することによって作成されます。
集約メソッド(cout<< charArray
など)を使用して文字配列を印刷するには、null
文字で文字配列を終了する必要があることに注意してください。C++ は\0
を NULL
文字として使用し、メソッドを集約して文字列の終わりを検出するのに役立ちます(終了マーク記号がないと配列の終わりを検出できません)。
したがって、以下の例では、C-language
には 10 文字しか含まれていませんが、サイズが 11
の文字配列を宣言しています。
char word[11] = {'C', '-', 'l', 'a', 'n', 'g', 'u', 'a', 'g', 'e', '\0'};
初期化ルールに従えば、上記のステートメントは次のように書くこともできます。
char word[] = "C-language";
null
文字を最後に置くのを忘れた場合、コンパイラは暗黙的に null
文字を最後に置きます。以下のプログラムを見てみましょう。
#include <iostream>
using namespace std;
int main() {
char word[11] = {'C', '-', 'l', 'a', 'n', 'g', 'u', 'a', 'g', 'e', '\0'};
cout << "First message: ";
cout << word << endl;
return 0;
}
上記のコードスニペットの Line#06 は、出力に First Message:
を提供し、line#07 は、\0
に遭遇するまで word
変数のすべての文字を表示します。したがって、上記のコードスニペットは次の出力を生成します。
First Message: C-language
C++ で文字列
ライブラリを使用する
C++ には、標準ライブラリの一部として組み込みの string.h
ヘッダーファイルがあります。これは、C スタイルの文字列(つまり、null で終了する文字列)を操作するための機能のバンドル(たとえば、strcpy
、strlen
など)を提供します。
.h
なしで含まれているすべての最新の string
ライブラリは、string.h
とは異なることに注意してください。string
クラスライブラリは最新の C++ 文字列を操作するための C++ ライブラリであり、string.h
は C スタイルの文字列(つまり、null で終了する文字列)を操作するための C ヘッダーファイルです。
string.h
ライブラリを理解するために、以下のコードを見てみましょう。
#include <string.h>
#include <iostream>
using namespace std;
int main() {
char country[] = "Pakistan";
char countryTemp[50] = "abc";
cout << "countryTemp length before initializing is:";
cout << strlen(countryTemp) << endl;
// strcpy()
cout << "Let's copy country to countryTemp" << endl;
strcpy(countryTemp, country);
cout << "countryTemp=" << countryTemp << endl;
cout << "countryTemp length after copying country is:";
cout << strlen(countryTemp) << endl;
return 0;
}
上記のプログラムは、最初に 2つの文字配列(C 文字列)を宣言し、最初の配列を Pakistan
で初期化します。Line#10 は、countryTemp
の長さである 3 を出力します。
countryTemp
の合計サイズは 50 バイトですが、strcpy
は NULL
文字(配列の 4 番目のバイトに配置)に基づいてのみサイズを計算できます。したがって、strcpy
は 3 を返します。
Line#14 は、strcpy
関数を使用して、country
配列の内容を countryTemp
にコピーします。したがって、countryTemp
の新しい長さは 8
になります。
出力:
countryTemp length before initializing is:3
Let's copy country to countryTemp
countryTemp=Pakistan
countryTemp length after copying country is:8
注:
NULL
で終了する文字列(C スタイルの文字列とも呼ばれます)のstring.h
ヘッダーファイルによって提供される関数は多数あります。その他の利用可能なライブラリ関数はここにあります。
C++ での文字列と文字の比較
文字列を文字定数と比較することは、人々にとって一般的な問題です。これは事実上不可能です。
以下のコードを検討してください。
#include <iostream>
using namespace std;
int main() {
cout << "Do you want to proceed (y or n)?\n";
char inp;
cin >> inp;
if (inp == "y") // error here
cout << "Hello again" << endl;
else
cout << "Good Bye" << endl;
return 0;
}
この関数では、char
変数であるユーザーからの入力を行い、if
条件で、二重引用符で囲まれた文字列リテラルと比較します。このコードは、error: ISO C++ forbids comparison between pointer and integer
エラーを生成します。
このエラーを回避するために、char
変数ではなく string
変数に入力することをお勧めします。
#include <iostream>
#include <string>
using namespace std;
int main() {
cout << "Do you want to proceed (y or n)?\n";
string ans;
cin >> ans;
if (ans == "y") // error here
cout << "Hello again" << endl;
else
cout << "Good Bye" << endl;
return 0;
}
出力:
Do you want to proceed (y or n)?
n
Good Bye
Husnain is a professional Software Engineer and a researcher who loves to learn, build, write, and teach. Having worked various jobs in the IT industry, he especially enjoys finding ways to express complex ideas in simple ways through his content. In his free time, Husnain unwinds by thinking about tech fiction to solve problems around him.
LinkedIn