How to Specify 64-Bit Integer in C++
- Understanding 64-Bit Integers
- Using the Standard Library
- Handling Large Numbers with Libraries
- Conclusion
- FAQ
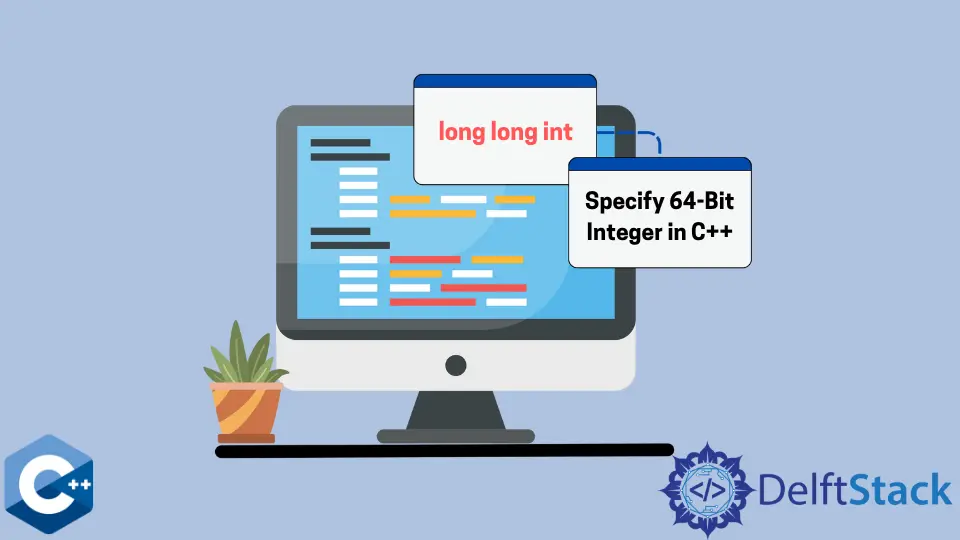
When working with large numbers in C++, specifying a 64-bit integer can be crucial for applications that require high precision and large ranges.
This tutorial will guide you through the process of defining and using 64-bit integers in C++. Whether you’re developing applications for data processing, scientific computing, or any domain that requires handling large integers, understanding how to work with 64-bit integers is essential. We’ll explore different methods to achieve this, including the use of standard data types and libraries that facilitate working with large numbers. By the end of this article, you’ll have a solid grasp of how to specify and manipulate 64-bit integers in C++.
Understanding 64-Bit Integers
A 64-bit integer can hold significantly larger values than its 32-bit counterpart. In C++, the standard data type for a 64-bit integer is long long
or int64_t
from the <cstdint>
library. Using these types allows you to perform arithmetic operations on very large numbers without overflow issues.
Here’s how you can declare a 64-bit integer using both methods:
#include <iostream>
#include <cstdint>
int main() {
long long largeNumber1 = 9223372036854775807; // Maximum value for signed 64-bit
int64_t largeNumber2 = -9223372036854775807; // Minimum value for signed 64-bit
std::cout << "First 64-bit integer: " << largeNumber1 << std::endl;
std::cout << "Second 64-bit integer: " << largeNumber2 << std::endl;
return 0;
}
Output:
First 64-bit integer: 9223372036854775807
Second 64-bit integer: -9223372036854775807
In this code snippet, we declare two 64-bit integers using both long long
and int64_t
. The long long
type is a built-in type in C++, while int64_t
is defined in the <cstdint>
library, which provides fixed-width integer types. The program then outputs the maximum and minimum values for signed 64-bit integers, demonstrating the range these types can cover.
Using the Standard Library
C++ offers a robust standard library that can simplify the handling of 64-bit integers. The std::int64_t
type is part of the <cstdint>
header, which provides a clear and portable way to work with fixed-width integers. This is particularly useful when you’re writing code that needs to be compatible across different platforms.
Here’s how to use std::int64_t
in a practical scenario:
#include <iostream>
#include <cstdint>
int main() {
std::int64_t totalBytes = 1LL << 40; // 1 Terabyte in bytes
std::int64_t usedBytes = 512LL << 20; // 512 Megabytes in bytes
std::cout << "Total Bytes: " << totalBytes << std::endl;
std::cout << "Used Bytes: " << usedBytes << std::endl;
std::cout << "Free Bytes: " << (totalBytes - usedBytes) << std::endl;
return 0;
}
Output:
Total Bytes: 1099511627776
Used Bytes: 536870912
Free Bytes: 1099511622144
In this example, we calculate the total and used bytes in a storage system. The std::int64_t
type allows us to handle large values comfortably. The left shift operator (<<
) is used to compute powers of 2, making it easy to represent sizes in bytes. This example not only highlights the usage of 64-bit integers but also demonstrates how they can be applied in real-world scenarios, such as memory management.
Handling Large Numbers with Libraries
For even more complex operations involving large numbers, consider using libraries like GMP (GNU Multiple Precision Arithmetic Library). This library provides a way to handle integers of arbitrary size, which can be useful when dealing with numbers larger than what 64-bit integers can store.
Here’s a simple example using GMP:
#include <iostream>
#include <gmpxx.h>
int main() {
mpz_class largeNum1("123456789012345678901234567890");
mpz_class largeNum2("987654321098765432109876543210");
mpz_class sum = largeNum1 + largeNum2;
std::cout << "Sum: " << sum << std::endl;
return 0;
}
Output:
Sum: 1111111110111111111011111111100
In this code, we utilize the mpz_class
from the GMP library to handle extremely large integers. The numbers are defined as strings to avoid overflow issues. The library automatically manages memory and operations on these large numbers. This approach is especially useful in applications involving cryptography, large-scale simulations, or any domain where precision is paramount.
Conclusion
Specifying and working with 64-bit integers in C++ is straightforward when you use the right data types and libraries. Whether you choose to use long long
, int64_t
, or even specialized libraries like GMP, understanding how to handle large numbers is essential for developing robust applications. With this knowledge, you can ensure that your applications can handle a wide range of numerical data without running into overflow issues.
FAQ
-
What is a 64-bit integer in C++?
A 64-bit integer in C++ is a data type that can hold large integer values, typically represented aslong long
orint64_t
. -
How do I ensure portability when using 64-bit integers?
Use theint64_t
type from the<cstdint>
library, which provides a fixed-width integer type that is consistent across different platforms. -
Can I perform arithmetic operations on 64-bit integers?
Yes, you can perform standard arithmetic operations like addition, subtraction, multiplication, and division on 64-bit integers just like any other integer type. -
What should I do if I need to work with numbers larger than 64 bits?
Consider using libraries like GMP, which allows you to work with integers of arbitrary size, making it suitable for applications that require high precision. -
Are there any performance considerations when using 64-bit integers?
While 64-bit integers provide a larger range, they may consume more memory than 32-bit integers. Always consider the trade-offs based on your application’s requirements.