Comparison Between Signed and Unsigned Integer Expressions in C++
- Understanding Signed and Unsigned Integers in C++
- Comparison Between Signed and Unsigned Integer Expressions in C++
- Arithmetic Operations and Overflow
- Comparison in Loops
- Comparison in Function Arguments
- Conclusion
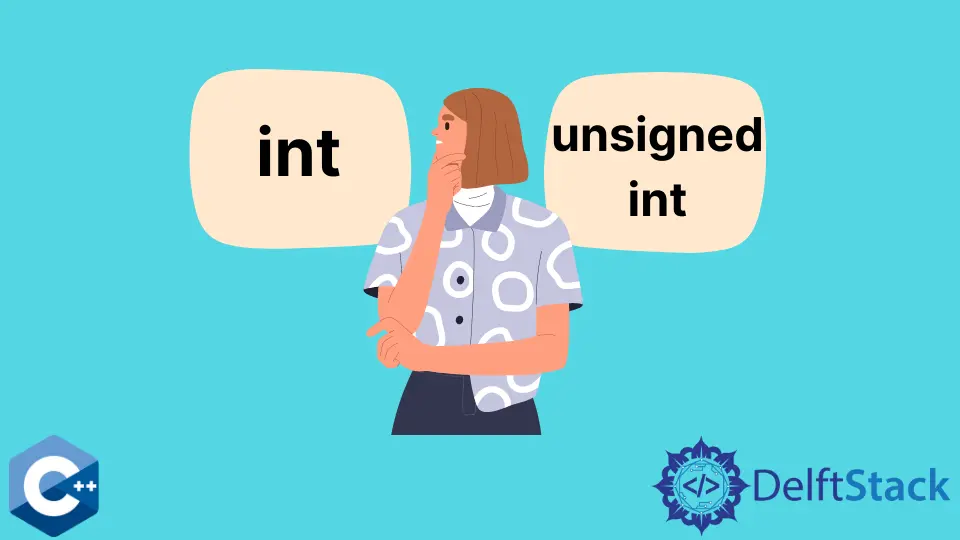
In C++, both signed and unsigned integer types play a crucial role in representing and manipulating data. Understanding the differences between signed and unsigned integer expressions is essential for writing robust and bug-free code.
In this article, we will explore the characteristics of signed and unsigned integers, their impact on arithmetic operations, and common scenarios where each type is best suited.
Understanding Signed and Unsigned Integers in C++
Signed Integers
In C++, the int
data type is signed by default. Signed integers can represent both positive and negative numbers, including zero.
The leftmost bit (most significant bit) is reserved for the sign, where 0
denotes a positive number, and 1
denotes a negative number. The range of a signed int
is typically from -2,147,483,648
to 2,147,483,647
on systems using 32 bits.
Unsigned Integers
The unsigned int
data type represents only non-negative integers. It uses all bits to represent the magnitude of the number.
As a result, unsigned integers can represent a larger positive range but cannot represent negative numbers. The range of an unsigned int
is usually from 0
to 4,294,967,295
on systems using 32 bits.
Comparison Between Signed and Unsigned Integer Expressions in C++
C++ supports signed and unsigned integers, which are written as int
and unsigned int
. When using signed integers, you can use the relational operators to compare two values.
The relational operators will not work because they always evaluate false
or true
when using unsigned integers. Signed numbers are typically faster than unsigned integers on most processors because they use less memory than unsigned integers.
Signed integers require more memory than unsigned integers because they store negative and positive numbers. This means that signed integers will use more memory for a given amount of data than unsigned ones.
While unsigned integers can represent only positive numbers if you add two different values, you will always get a result with the same sign (+
or -
) for unsigned integers. For signed integers, if you add two different values, you will get a result with two different signs (+
or -
).
C++ Compiler Warning
Compilers warn comparisons between signed and unsigned types because the ranges of signed and unsigned int
s vary and can deliver unexpected performance.
It also cannot guarantee the safety of your program because signed and unsigned integer expressions are mixed. This can happen when a negative number is converted to an unsigned type or a positive number is converted to a signed one.
The compiler will not warn you if the expression has only one operand, a signed type, whose value fits in the destination type.
Let’s discuss an example to understand better how the compiler warns:
#include <iostream>
#include <string>
using namespace std;
int helloWorld(string input);
int main() {
string input;
input = "sample";
helloWorld(input);
}
int helloWorld(string input) {
int demo = 0;
for (int x = 0; x < input.length(); x++) {
if (input.substr(x, 4) == " ") {
demo++;
}
}
cout << demo;
}
Compiler output:
main.cpp: In function ‘int helloWorld(std::string)’:
main.cpp:26:1: warning: no return statement in function returning non-void [-Wreturn-type]
26 | }
| ^
0
Click this link to check the live demonstration of the code.
This program counts the occurrences of a specific substring (" "
) within a given input string ("sample"
). It defines a function helloWorld
that iterates through each character in the input, checking for occurrences of the substring.
The count is then printed to the console. In this example, as there are no spaces in the input string, the output is 0
.
Arithmetic Operations and Overflow
Signed Integers
One critical consideration when working with signed integers is the potential for overflow. Overflow occurs when the result of an arithmetic operation exceeds the maximum representable value or falls below the minimum representable value.
For example:
int a = INT_MAX; // Maximum value for a signed int
int b = 1;
int result = a + b; // Overflow occurs here
In this case, the result of adding 1 to INT_MAX
causes overflow, leading to undefined behavior.
Unsigned Integers
Unsigned integers, on the other hand, do not have negative values and do not experience overflow in the same way. Instead, they wrap around to zero when the maximum representable value is exceeded.
For example:
unsigned int a = UINT_MAX; // Maximum value for an unsigned int
unsigned int b = 1;
unsigned int result = a + b; // Wraps around to 0
In this scenario, adding 1 to UINT_MAX
results in the value wrapping around to 0.
Comparison in Loops
When using loops, especially for
loops, the choice between signed and unsigned integers can impact loop termination conditions. Mixing signed and unsigned types in loop conditions can lead to unintended consequences.
Example: Loop Termination
for (int i = 10; i >= 0; --i) {
// Loop body
}
This loop is prone to an infinite loop because i
will never be less than 0 due to the wrap-around behavior of signed integers. A more suitable approach is to use an unsigned integer:
for (unsigned int i = 10; i >= 0; --i) {
// Loop body
}
Comparison in Function Arguments
When designing functions that accept integer parameters, the choice between signed and unsigned types influences the function’s behavior and flexibility.
Example: Function Parameter
void printValue(int value) {
// Function body
}
Using int
as the parameter type implies that negative values are valid inputs. If negative values are not intended, using an unsigned integer may be more appropriate:
void printValue(unsigned int value) {
// Function body
}
Conclusion
Choosing between signed and unsigned integers in C++ involves considering the range of values, the potential for overflow, and the specific requirements of your program. Understanding the implications of each type on arithmetic operations, loops, and function parameters is crucial for writing robust and error-free code.
While signed integers offer a broader range that includes negative values, unsigned integers provide a larger positive range and avoid certain overflow issues. Carefully selecting the appropriate type for each scenario is essential for efficient and bug-free C++ programming.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook