How to Check if a Number Is Prime in C++
- Method 1: Trial Division
- Method 2: Sieve of Eratosthenes
- Method 3: Optimized Trial Division
- Conclusion
- FAQ
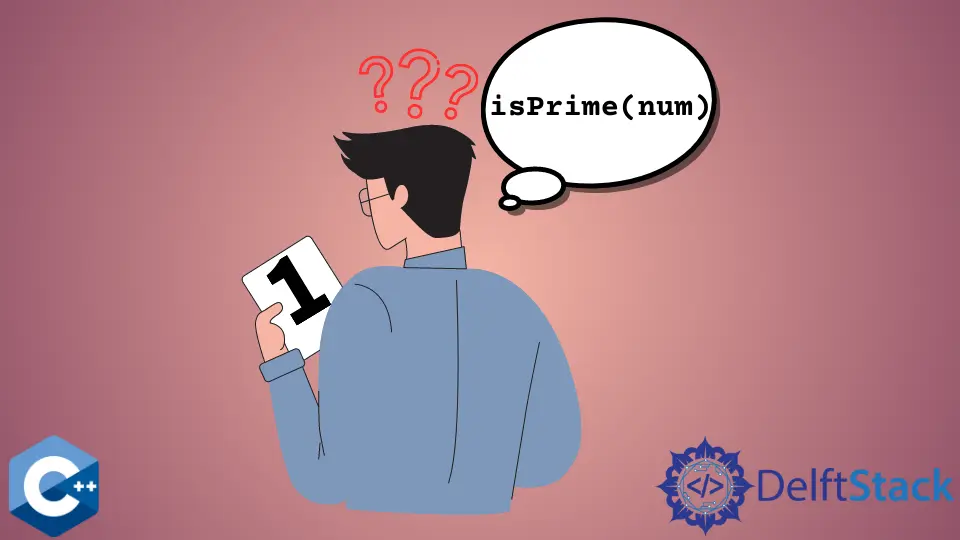
Determining whether a number is prime is a fundamental task in computer science and mathematics. A prime number is defined as a natural number greater than 1 that has no positive divisors other than 1 and itself.
This article will demonstrate how to check if a number is prime using C++. We will explore various methods, including trial division, the Sieve of Eratosthenes, and optimized algorithms. By the end of this guide, you’ll have a solid understanding of how to implement these techniques in your C++ programs. Whether you’re a beginner or an experienced programmer, this article is designed to enhance your coding skills and deepen your understanding of prime numbers.
Method 1: Trial Division
The simplest way to check for a prime number is through trial division. This method involves dividing the number by every integer up to its square root. If any division results in a whole number, the number is not prime. Here’s how you can implement this in C++.
#include <iostream>
#include <cmath>
bool isPrime(int n) {
if (n <= 1) return false;
for (int i = 2; i <= sqrt(n); i++) {
if (n % i == 0) return false;
}
return true;
}
int main() {
int number;
std::cout << "Enter a number: ";
std::cin >> number;
if (isPrime(number)) {
std::cout << number << " is a prime number." << std::endl;
} else {
std::cout << number << " is not a prime number." << std::endl;
}
return 0;
}
Output:
Enter a number: 17
17 is a prime number.
In this code, we first check if the number is less than or equal to 1, in which case it cannot be prime. We then loop through all integers from 2 to the square root of the number. If we find any integer that divides the number evenly, we return false, indicating it’s not prime. Otherwise, we return true, confirming it is a prime number. This method is straightforward and effective for small numbers.
Method 2: Sieve of Eratosthenes
For checking multiple numbers or generating a list of prime numbers, the Sieve of Eratosthenes is a more efficient algorithm. It works by iteratively marking the multiples of each prime number starting from 2. Here’s how you can implement it in C++.
#include <iostream>
#include <vector>
void sieveOfEratosthenes(int n) {
std::vector<bool> prime(n + 1, true);
prime[0] = prime[1] = false; // 0 and 1 are not prime numbers
for (int p = 2; p * p <= n; p++) {
if (prime[p]) {
for (int i = p * p; i <= n; i += p) {
prime[i] = false;
}
}
}
std::cout << "Prime numbers up to " << n << " are: ";
for (int p = 2; p <= n; p++) {
if (prime[p]) {
std::cout << p << " ";
}
}
std::cout << std::endl;
}
int main() {
int limit;
std::cout << "Enter the limit: ";
std::cin >> limit;
sieveOfEratosthenes(limit);
return 0;
}
Output:
Enter the limit: 30
Prime numbers up to 30 are: 2 3 5 7 11 13 17 19 23 29
In this implementation, we create a boolean vector initialized to true, representing that all numbers are prime initially. We then start from the first prime number (2) and mark all its multiples as non-prime. We continue this process for all numbers up to the square root of the limit. Finally, we print all numbers that remain marked as prime. This method is efficient and works well for generating a list of primes up to a specified limit.
Method 3: Optimized Trial Division
While trial division is simple, it can be optimized further by eliminating even numbers and only checking odd divisors. This method significantly reduces the number of checks needed for larger numbers.
#include <iostream>
bool isPrimeOptimized(int n) {
if (n <= 1) return false;
if (n <= 3) return true;
if (n % 2 == 0 || n % 3 == 0) return false;
for (int i = 5; i * i <= n; i += 6) {
if (n % i == 0 || n % (i + 2) == 0) return false;
}
return true;
}
int main() {
int number;
std::cout << "Enter a number: ";
std::cin >> number;
if (isPrimeOptimized(number)) {
std::cout << number << " is a prime number." << std::endl;
} else {
std::cout << number << " is not a prime number." << std::endl;
}
return 0;
}
Output:
Enter a number: 29
29 is a prime number.
In this optimized version, we first check for small numbers and even divisibility by 2 and 3. After that, we only check odd numbers starting from 5, incrementing by 6 each time. This allows us to skip even numbers and multiples of 3, significantly reducing the number of iterations needed. This method is efficient for larger numbers and retains the simplicity of the trial division approach.
Conclusion
In this article, we explored different methods to check if a number is prime in C++. From the straightforward trial division to the efficient Sieve of Eratosthenes and optimized trial division, each method offers unique advantages depending on the context. Understanding these algorithms not only enhances your programming skills but also deepens your appreciation for the beauty of mathematics. Whether you’re working on a small project or a complex algorithm, knowing how to determine prime numbers is a valuable skill in your coding toolkit.
FAQ
-
What is a prime number?
A prime number is a natural number greater than 1 that cannot be formed by multiplying two smaller natural numbers. -
Why is the Sieve of Eratosthenes efficient?
The Sieve of Eratosthenes is efficient because it systematically eliminates multiples of prime numbers, allowing for the quick identification of primes up to a specified limit. -
Can negative numbers be prime?
No, prime numbers are defined only for natural numbers greater than 1, so negative numbers cannot be prime. -
How do I check if a large number is prime?
For large numbers, optimized algorithms like the Miller-Rabin primality test or using libraries designed for big integers can be more effective. -
What is the time complexity of trial division?
The time complexity of trial division is O(sqrt(n)), where n is the number being checked for primality.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook