如何在 C++ 中把字符串转换为 Int
Jinku Hu
2023年10月12日
C++
C++ String
C++ Integer
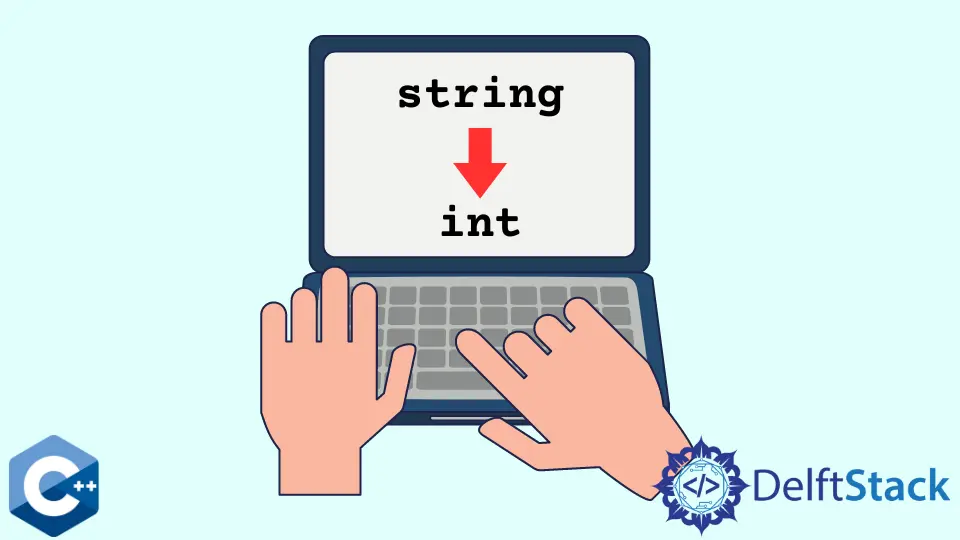
本文介绍了在 C++ 中把字符串转换为 int
的多种方法。
在 C++ 中使用 std::stoi
方法将 String 转换为 Int
stoi
方法是一个内置的 string
容器功能,用于转换为有符号的整数。该方法需要一个类型为 string
的必选参数来操作。我们也可以传递一些可选的参数,如字符串中的起始位置和数基。下面的代码示例演示了多种输入 string
的情况和相应的 stoi
用例。
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl using std::string;
using std::stoi;
int main() {
string s1 = "123";
string s2 = "-123";
string s3 = "123xyz";
string s4 = "-123xyz";
string s5 = "7B";
string s6 = "-7B";
string s7 = "1111011";
string s8 = "-1111011";
int num1, num2, num3, num4, num5, num6, num7, num8;
num1 = stoi(s1);
num2 = stoi(s2);
num3 = stoi(s3);
num4 = stoi(s4);
num5 = stoi(s5, nullptr, 16);
num6 = stoi(s6, nullptr, 16);
num7 = stoi(s7, nullptr, 2);
num8 = stoi(s8, nullptr, 2);
cout << "num1: " << num1 << " | num2: " << num2 << endl;
cout << "num3: " << num3 << " | num4: " << num4 << endl;
cout << "num5: " << num5 << " | num6: " << num6 << endl;
cout << "num7: " << num7 << " | num8: " << num8 << endl;
return EXIT_SUCCESS;
}
输出:
num1: 123 | num2: -123
num3: 123 | num4: -123
num5: 123 | num6: -123
num7: 123 | num8: -123
请注意,stoi
可以处理前导空格字符,+/-
符号,十六进制前缀(0x
或 0X
),甚至多个 0,并正确返回整数。它不能处理数字前的其他字符,如果发现一个,就会抛出异常 std::invalid_argument
。你可以通过下面的代码示例来了解其行为。
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl using std::string;
using std::stoi;
int main() {
string s1 = " 123";
;
string s2 = "xyz123";
;
int num1, num2;
num1 = stoi(s1);
cout << "num1: " << num1 << endl;
try {
num2 = stoi(s2);
cout << "num2: " << num2 << endl;
} catch (std::exception& e) {
cout << e.what() << " caused the error!!!\n";
}
return EXIT_SUCCESS;
}
输出:
num1: 123
stoi caused the error!!!
有多个 stoi
风格的方法可以转换为不同的数字类型,比如:std::stoi, std::stol, std::stoll
, std::stoul, std::stoull
, std::stof, std::stod, std::stold
,这些方法可以根据需要实现特定的功能。
在 C++ 中使用 std::from_chars
方法将字符串转换为 Int
from_chars
方法是 C++17 中对 utilities
库的补充,定义在 <charconv>
头文件中。它可以分析具有指定模式的字符序列。这样做的好处是处理时间更快,并且在解析失败的情况下能更好地处理异常。虽然对输入字符串有一些限制,即:前导空格/其他字符,甚至基数 16 的 0x
/0X
前缀也不能处理。对于有符号的整数,只识别前导减号。
std::from_chars
的语法
from_chars(const char* first, const char* last, TYPE& value, int base = 10)
参数 | 说明 |
---|---|
[first, last) |
要解析的字符地址范围 |
value |
如果解析成功,则为解析结果的变量。 |
base |
int 基数。默认为 10 。 |
#include <charconv>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl using std::string;
using std::from_chars;
using std::stoi;
int main() {
string s1 = "123";
string s2 = "-123";
string s3 = "123xyz";
string s4 = "-123xyz";
string s5 = "7B";
string s6 = "-7B";
string s7 = "1111011";
string s8 = "-1111011";
string s9 = " 123";
string s10 = "x123";
int num1, num2, num3, num4, num5, num6, num7, num8, num9, num10;
from_chars(s1.c_str(), s1.c_str() + s1.length(), num1);
from_chars(s2.c_str(), s2.c_str() + s2.length(), num2);
from_chars(s3.c_str(), s3.c_str() + s3.length(), num3);
from_chars(s4.c_str(), s4.c_str() + s4.length(), num4);
from_chars(s5.c_str(), s5.c_str() + s5.length(), num5, 16);
from_chars(s6.c_str(), s6.c_str() + s6.length(), num6, 16);
from_chars(s7.c_str(), s7.c_str() + s7.length(), num7, 2);
from_chars(s8.c_str(), s8.c_str() + s8.length(), num8, 2);
from_chars(s9.c_str(), s9.c_str() + s9.length(), num9);
from_chars(s10.c_str(), s10.c_str() + s10.length(), num10);
cout << "num1: " << num1 << " | num2: " << num2 << endl;
cout << "num3: " << num3 << " | num4: " << num4 << endl;
cout << "num5: " << num5 << " | num6: " << num6 << endl;
cout << "num7: " << num7 << " | num8: " << num8 << endl;
cout << "num9: " << num9 << " | num10: " << num10 << endl;
return EXIT_SUCCESS;
}
输出:
num1: 123 | num2: -123
num3: 123 | num4: -123
num5: 123 | num6: -123
num7: 123 | num8: -123
num9: -16777216 | num10: -1 // incorrect values varying over runs
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Jinku Hu
相关文章 - C++ String
- C++ 中字符串和字符的比较
- 从 C++ 中的字符串中获取最后一个字符
- 从 C++ 中的字符串中删除最后一个字符
- C++ 中的 std::string::erase 函数
- C++ 中字符串的 sizeof 运算符和 strlen 函数的区别
- 在 C++ 中打印字符串的所有排列