How to Convert Int to Char Array in C++
-
Method 1: Using
sprintf
-
Method 2: Using
itoa
-
Method 3: Using
std::to_string
andc_str
-
Use
std::stringstream
Class Methods -
Use
std::to_chars
Function - Conclusion
- FAQ
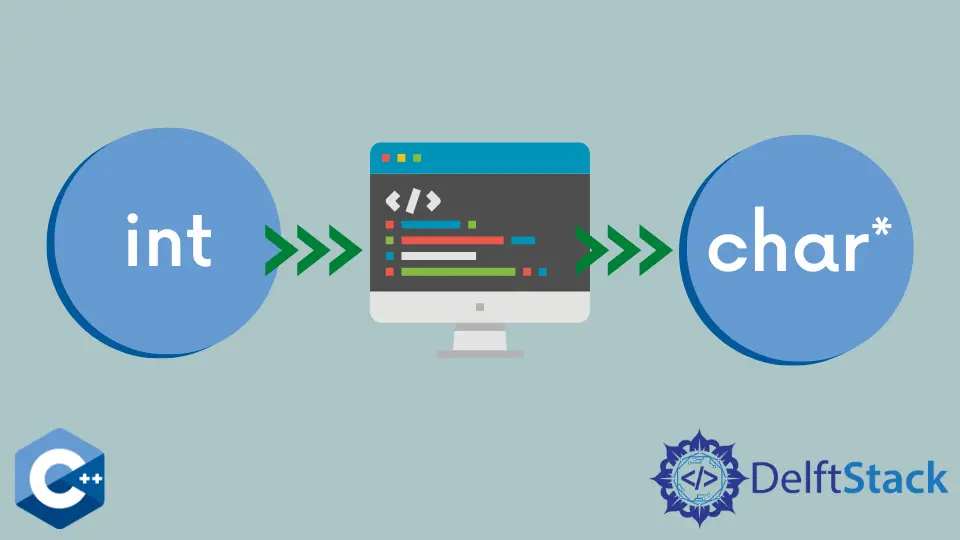
Converting an integer to a character array in C++ is a common task that many programmers encounter. Whether you’re working on a simple project or a complex application, understanding how to perform this conversion can enhance your coding skills and improve the efficiency of your programs.
In this article, we will delve into several methods to convert the integer data type to a character string. Each method will be explained in detail, complete with code examples and output demonstrations. By the end of this article, you’ll be well-equipped to handle integer-to-character conversions in your C++ projects. Let’s get started!
Method 1: Using sprintf
One of the most straightforward ways to convert an integer to a character array in C++ is by using the sprintf
function. This function formats and stores a series of characters and values in a string. It can be particularly useful for creating formatted strings from various data types.
Here’s how you can use sprintf
to convert an integer to a character array:
#include <iostream>
#include <cstdio>
int main() {
int num = 12345;
char buffer[20];
sprintf(buffer, "%d", num);
std::cout << "Character Array: " << buffer << std::endl;
return 0;
}
Output:
Character Array: 12345
In this example, we declare an integer num
with the value 12345
. We also create a character array called buffer
that will hold the resulting string. The sprintf
function is then used to format the integer into the character array. The format specifier %d
indicates that we are converting an integer. Finally, we print the character array to the console. This method is efficient and widely used, making it a great choice for converting integers to character arrays.
Method 2: Using itoa
Another popular method for converting an integer to a character array in C++ is using the itoa
function. This function converts an integer to a string representation in a specified base. It’s particularly useful when you want to convert numbers into different numeral systems, such as binary, octal, decimal, or hexadecimal.
Here’s how to use itoa
:
#include <iostream>
#include <cstdlib>
int main() {
int num = 67890;
char buffer[20];
itoa(num, buffer, 10);
std::cout << "Character Array: " << buffer << std::endl;
return 0;
}
Output:
Character Array: 67890
In this code, we define an integer num
with the value 67890
. We also create a character array called buffer
to store the result. The itoa
function is used here, where the first argument is the integer to convert, the second is the character array to store the result, and the third is the base (in this case, base 10 for decimal). After the conversion, we print the character array to the console. This method is straightforward and can be particularly effective when dealing with different numeral systems.
Method 3: Using std::to_string
and c_str
If you prefer a more modern C++ approach, you can use the std::to_string
function, which is part of the C++11 standard. This function converts various data types, including integers, to strings. You can then convert the resulting string to a character array using the c_str()
method.
Here’s how to do it:
#include <iostream>
#include <string>
int main() {
int num = 54321;
std::string str = std::to_string(num);
const char* charArray = str.c_str();
std::cout << "Character Array: " << charArray << std::endl;
return 0;
}
Output:
Character Array: 54321
In this example, we first convert the integer num
to a string using std::to_string
. The resulting string is stored in the variable str
. To obtain a character array, we call the c_str()
method on str
, which returns a pointer to a null-terminated character array representing the string. Finally, we print the character array to the console. This method is clean and leverages modern C++ features, making it a great choice for those familiar with C++11 and beyond.
Use std::stringstream
Class Methods
This method is implemented using[the std::stringstream
class. Namely, we create a temporary string-based stream where store int
data, then return string object by the str
method and finally call c_str
to do the conversion.
#include <iostream>
#include <sstream>
int main() {
int number = 1234;
std::stringstream tmp;
tmp << number;
char const *num_char = tmp.str().c_str();
printf("num_char: %s \n", num_char);
;
return 0;
}
The above code demonstrates how to convert an integer to a C-style string (const char*) using C++ streams. It uses the std::stringstream
class from the <sstream>
header. First, an integer number
is defined. Then, a stringstream
object tmp
is created, and the number is inserted into it using the stream insertion operator <<
. The str()
method is called on tmp
to get the string representation, and c_str()
is used to convert this to a C-style string. Finally, the result is printed using printf
. This method is useful when you need to convert numbers to strings in a type-safe manner, especially when working with legacy C code or APIs that require C-style strings.
Use std::to_chars
Function
This version is a pure C++ style function added in C++17 and defined in header <charconv>
. On the plus side, this method offers operations on ranges, which might be the most flexible solution in specific scenarios.
#include <charconv>
#include <iostream>
#define MAX_DIGITS 10
int main() {
int number = 1234;
char num_char[MAX_DIGITS + sizeof(char)];
std::to_chars(num_char, num_char + MAX_DIGITS, number);
std::printf("num_char: %s \n", num_char);
return 0;
}
This code showcases a modern C++ approach to convert an integer to a C-style string using the <charconv>
header, introduced in C++17. It defines a constant MAX_DIGITS
to allocate sufficient space for the string representation. The std::to_chars
function is used to perform the conversion, which writes the string representation of number
directly into the num_char
array. This method is highly efficient and doesn’t require creating temporary string objects. It’s particularly useful in performance-critical code or when working with fixed-size buffers. The result is then printed using std::printf
. This approach combines C++’s type safety with C-style string handling, offering a balance of efficiency and modern language features.
Conclusion
In this article, we explored three effective methods for converting an integer to a character array in C++. From the traditional sprintf
and itoa
functions to the more modern std::to_string
, each method has its own advantages and use cases. Understanding these methods will not only enhance your C++ programming skills but also enable you to handle string manipulations with ease. Remember to choose the method that best fits your project requirements and coding style. Happy coding!
FAQ
-
What is the difference between
sprintf
anditoa
?
sprintf
is a general-purpose formatting function, whileitoa
is specifically designed for converting integers to strings in different bases. -
Can I use
std::to_string
in C++ versions prior to C++11?
No,std::to_string
is only available starting from C++11. For earlier versions, you can usesprintf
oritoa
. -
Is there a performance difference between these methods?
Generally,itoa
andsprintf
may perform faster thanstd::to_string
, but the difference is usually negligible for most applications. -
Can I convert negative integers using these methods?
Yes, all the methods discussed can handle negative integers properly. -
Are there any limitations to the size of the integer I can convert?
The size limit depends on the data type you are using. Forint
, it typically ranges from -2,147,483,648 to 2,147,483,647.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookRelated Article - C++ Integer
- Use of 128-Bit Integer in C++
- Big Integer in C++
- How to Specify 64-Bit Integer in C++
- Comparison Between Signed and Unsigned Integer Expressions in C++
- INT_MAX and INT_MIN Macro Expressions in C++
- How to Check if a Number Is Prime in C++