How to Get ASCII Value of Char in C++
-
Use
std::copy
andstd::ostream_iterator
to Get the ASCII Value of achar
in C++ -
Use the
printf
Format Specifiers to Get the ASCII Value of achar
in C++ -
Use
int()
to Get the ASCII Value of achar
in C++ -
Use Type Casting to Get the ASCII Value of a
char
in C++ - Conclusion
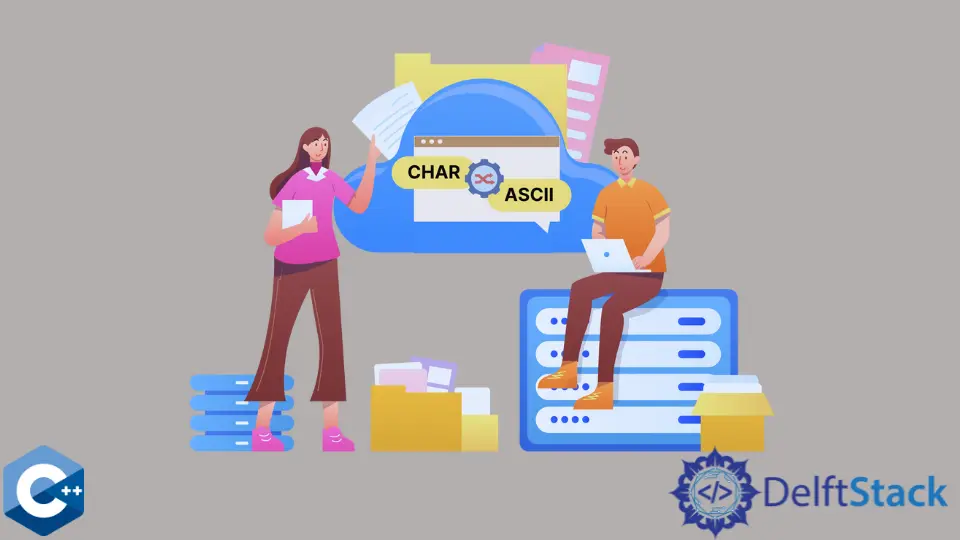
In C++, obtaining the ASCII values of characters is a common task with multiple methods available.
This article will explore various techniques, including type casting, printf
format specifiers, int()
conversion, and the use of std::copy
with std::ostream_iterator
.
Each method provides a unique approach, catering to different preferences and coding scenarios.
Use std::copy
and std::ostream_iterator
to Get the ASCII Value of a char
in C++
In C++, you can easily retrieve the ASCII values of characters using the std::copy
algorithm along with std::ostream_iterator
. This method allows you to output the ASCII values directly to the console.
The std::copy
algorithm is part of the C++ Standard Template Library (STL), and it is used for copying elements from one container to another. In this case, we use it to copy the characters from a vector of characters to the console.
On the other hand, std::ostream_iterator
is an output iterator that writes values to an output stream. By combining these two, we can seamlessly output the ASCII values of characters to the console.
The key syntax involves specifying the range of elements to copy (chars.begin()
to chars.end()
), the destination iterator (std::ostream_iterator<int>(cout, "; ")
), and the source container (chars
).
The int
in std::ostream_iterator<int>
is crucial as it tells the iterator to treat the values as integers, ensuring that ASCII values are correctly displayed.
Here’s an example:
#include <iostream>
#include <iterator>
#include <vector>
int main() {
std::vector<char> chars{'g', 'h', 'T', 'U', 'q', '%',
'+', '!', '1', '2', '3'};
std::cout << "ASCII values: ";
std::copy(chars.begin(), chars.end(),
std::ostream_iterator<int>(std::cout, "; "));
return 0;
}
In this code, we first include necessary header files (<iostream>
, <iterator>
, and <vector>
). We then define the main
function, where we initialize a vector chars
with a sequence of characters.
The std::copy
function takes the range of characters from chars.begin()
to chars.end()
and copies them to the output stream (std::cout
) using std::ostream_iterator<int>
.
The std::ostream_iterator<int>(std::cout, "; ")
specifies that a semicolon and a space should follow each value. The int
indicates that the values should be treated as integers, displaying the ASCII values rather than the characters themselves.
Output:
ASCII values: 103; 104; 84; 85; 113; 37; 43; 33; 49; 50; 51;
In the output, you can observe the ASCII values of the characters from the chars
vector, separated by semicolons and spaces.
Use the printf
Format Specifiers to Get the ASCII Value of a char
in C++
Another approach to retrieve the ASCII values of characters is by utilizing the versatile printf
function with specific format specifiers. This method allows you to print both the character and its corresponding ASCII value clearly and concisely.
In this context, we use %c
to represent the character and %d
to represent its ASCII value. The syntax involves providing these format specifiers within the format string, followed by the actual values to be displayed.
Notably, %c
is used for both character types and %d
for integer types, allowing seamless integration of character and ASCII value representation.
Below is an example:
#include <iostream>
#include <vector>
int main() {
std::vector<char> chars{'g', 'h', 'T', 'U', 'q', '%',
'+', '!', '1', '2', '3'};
std::cout << std::endl;
for (const auto &character : chars) {
printf("The ASCII value of '%c' is: %d\n", character, character);
}
return 0;
}
In this example, after including the necessary header files (<iostream>
and <vector>
), we define the main
function. A vector named chars
is initialized with a sequence of characters.
The program then enters a loop where each character is converted to its ASCII value using int(character)
. The result is then printed to the console along with a descriptive message.
The conversion is performed within the std::cout
statement, where we use int(character)
to obtain the ASCII value of each character.
Output:
The ASCII value of 'g' is: 103
The ASCII value of 'h' is: 104
The ASCII value of 'T' is: 84
The ASCII value of 'U' is: 85
The ASCII value of 'q' is: 113
The ASCII value of '%' is: 37
The ASCII value of '+' is: 43
The ASCII value of '!' is: 33
The ASCII value of '1' is: 49
The ASCII value of '2' is: 50
The ASCII value of '3' is: 51
As evident in the output, this method yields the ASCII values of characters, providing a concise and readable representation.
Use Type Casting to Get the ASCII Value of a char
in C++
Obtaining the ASCII values of characters can also be accomplished through typecasting. Type casting involves explicitly converting one data type to another, and in this case, we cast char
to int
to interpret the character as its corresponding ASCII value.
The syntax involves using the static_cast
operator or the traditional C-style casting (int)
to explicitly cast a char
to an int
. This conversion works seamlessly because the ASCII values of characters align with their integer representations.
The resulting integer value can then be used as needed, such as for printing or further processing.
#include <iostream>
#include <vector>
int main() {
std::vector<char> chars{'g', 'h', 'T', 'U', 'q', '%',
'+', '!', '1', '2', '3'};
std::cout << std::endl;
for (auto &character : chars) {
int asciiValue = static_cast<int>(character);
std::cout << "The ASCII value of '" << character << "' is: " << asciiValue
<< std::endl;
}
return 0;
}