How to Convert ASCII to Char in C++
- Understanding ASCII and Characters in C++
- Method 1: Using Type Casting
- Method 2: Using Character Arithmetic
- Method 3: Using Functions for Conversion
- Conclusion
- FAQ
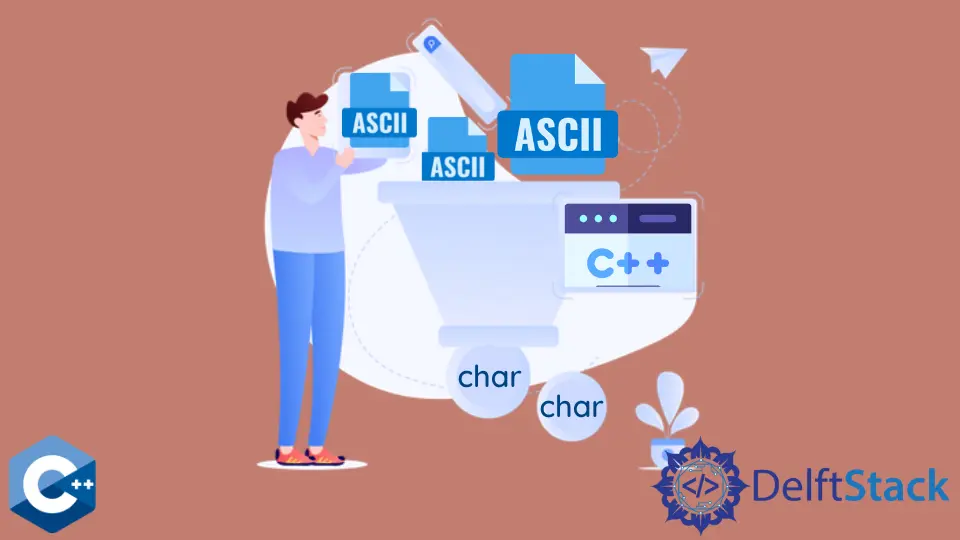
Converting ASCII values to characters in C++ is a fundamental task that every programmer should understand. Whether you’re working on a simple project or diving into more complex applications, knowing how to manipulate ASCII values can enhance your coding skills.
In this article, we will explore different methods to convert ASCII values to characters in C++. We’ll delve into practical examples that demonstrate how to achieve this conversion seamlessly. By the end of this guide, you’ll have a solid grasp of the techniques available and be ready to implement them in your own projects. So, let’s get started!
Understanding ASCII and Characters in C++
Before we dive into the conversion methods, it’s essential to understand what ASCII is. ASCII, which stands for American Standard Code for Information Interchange, is a character encoding standard that uses numeric codes to represent characters. For instance, the ASCII value for the character ‘A’ is 65, while ‘a’ is 97. In C++, you can easily convert these numeric values back into their corresponding characters using simple arithmetic and type casting.
Method 1: Using Type Casting
One of the simplest methods to convert an ASCII value to a character in C++ is through type casting. This method involves converting an integer ASCII value directly to a char type. Here’s how you can do it:
#include <iostream>
using namespace std;
int main() {
int asciiValue = 65; // ASCII value for 'A'
char character = static_cast<char>(asciiValue);
cout << "The character for ASCII value " << asciiValue << " is: " << character << endl;
return 0;
}
Output:
The character for ASCII value 65 is: A
In this example, we first define an integer variable asciiValue
and assign it the ASCII value of ‘A’, which is 65. Using static_cast<char>
, we convert the integer to a character type. Finally, we print the result. This method is straightforward and effective for converting any valid ASCII value.
Method 2: Using Character Arithmetic
Another interesting approach to convert ASCII values to characters in C++ is through character arithmetic. This method leverages the fact that characters in C++ are essentially represented by their ASCII values. Here’s how you can implement this:
#include <iostream>
using namespace std;
int main() {
int asciiValue = 97; // ASCII value for 'a'
char character = asciiValue; // Implicit conversion
cout << "The character for ASCII value " << asciiValue << " is: " << character << endl;
return 0;
}
Output:
The character for ASCII value 97 is: a
In this code snippet, we directly assign the ASCII value of ‘a’ (97) to a char variable named character
. The C++ compiler automatically performs the conversion due to implicit type conversion. This method is clean and efficient, allowing you to convert ASCII values without needing explicit casting.
Method 3: Using Functions for Conversion
If you want to encapsulate the conversion logic in a reusable manner, creating a function is an excellent idea. This method allows you to convert ASCII values to characters easily throughout your code. Here’s an example:
#include <iostream>
using namespace std;
char asciiToChar(int asciiValue) {
return static_cast<char>(asciiValue);
}
int main() {
int asciiValue = 90; // ASCII value for 'Z'
char character = asciiToChar(asciiValue);
cout << "The character for ASCII value " << asciiValue << " is: " << character << endl;
return 0;
}
Output:
The character for ASCII value 90 is: Z
In this example, we define a function asciiToChar
that takes an integer as input and returns its corresponding character. This makes the code modular and reusable across different parts of your application. The function uses type casting to perform the conversion, ensuring that you can easily convert any ASCII value.
Conclusion
Converting ASCII values to characters in C++ is a straightforward process that can be accomplished using various methods, including type casting, character arithmetic, and function encapsulation. Each method has its advantages, and the choice largely depends on your coding style and the specific requirements of your project. With the examples provided, you should feel confident in implementing these conversions in your own C++ programs. As you continue to explore C++, mastering these fundamental concepts will undoubtedly enhance your programming skills.
FAQ
-
What is ASCII?
ASCII stands for American Standard Code for Information Interchange, a character encoding standard that uses numeric codes to represent characters. -
Can I convert any integer to a char in C++?
You can convert any integer to a char, but it must correspond to a valid ASCII value (0-127 for standard ASCII). -
What happens if I convert an invalid ASCII value?
Converting an invalid ASCII value may lead to undefined behavior or unexpected characters being displayed. -
Is there a built-in function in C++ for ASCII conversion?
No, there is no built-in function specifically for ASCII conversion, but you can easily create your own using type casting. -
Can I convert characters back to ASCII values in C++?
Yes, you can convert characters back to their ASCII values by simply assigning a char to an int variable.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook