How to Convert Char Array to String in C++
- Character Arrays in C++
- Strings in C++
-
Use the
for
Loop and+
Operator to Convert a Character Array to String in C++ -
Use the
while
Loop and+
Operator to Convert a Character Array to String in C++ -
Use the
string
Constructor to Convert a Character Array to String in C++ - Conclusion
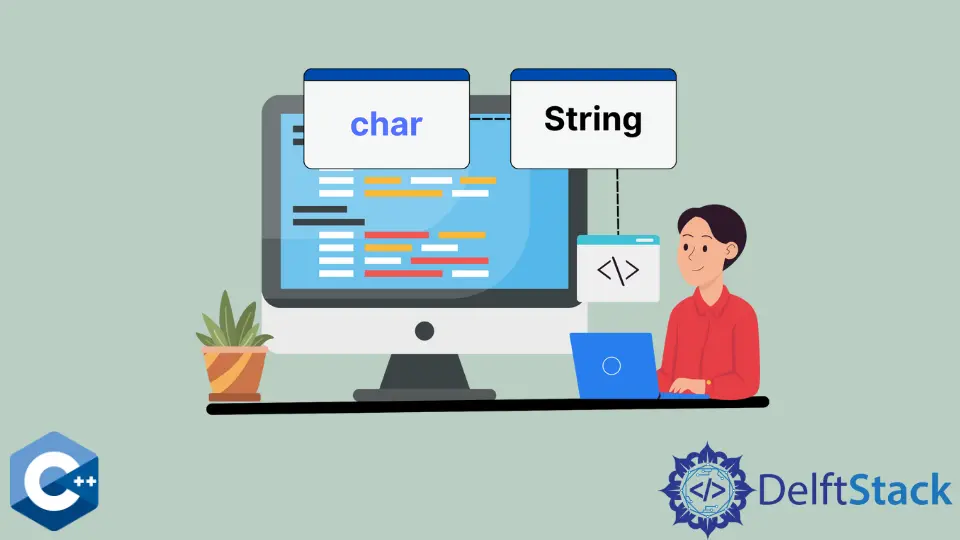
This tutorial discusses the several ways in which we can convert a character array to a string in C++. Let us begin by explaining a little bit about character arrays and strings in C++.
Character Arrays in C++
Arrays are a crucial concept in programming and are used extensively to represent and manipulate data efficiently. Elements in an array are stored in contiguous memory locations, and each element is accessed using an index or a key.
In C++, the char
data type is used to represent individual characters. It is a fundamental data type and is commonly used for storing characters, which can include letters, digits, punctuation, and special symbols.
A character array is a sequence of characters stored in contiguous memory locations. It is a fundamental data type used to represent and manipulate strings.
Strings in C++
In C++, strings are sequences of characters represented by the string
class, which is part of the C++ Standard Library. It provides a convenient and powerful way to work with strings.
It is more versatile and user-friendly than character arrays.
Strings and character arrays are used to represent a sequence of characters in C++. On the front end, they both look identical but they have a lot of differences in the back end.
A character array is terminated by a NULL
character and is fixed in lengths. Such a character does not terminate a string, and the memory and length of a string can be dynamically adjusted as per the programmer’s need.
The string class also provides a rich collection of functions that help to work with string objects.
Use the for
Loop and +
Operator to Convert a Character Array to String in C++
The for
loop allows a programmer to execute a set of statements a repeated number of times based on a condition. It is an entry-controlled loop, meaning that the condition is evaluated before loop execution.
In C++, you can use a for
loop to iterate through elements of an array. We can adapt the loop to work with arrays of different sizes or types.
The key is to adjust the loop’s initialization
, condition
, and update
statements accordingly.
Along with the for
loop, we can use the +
operator for string concatenation. We should note that the behavior of the +
operator can be overloaded for user-defined types, allowing one to define custom addition behavior for objects of a class or structure.
In this method, we will iterate through a character array and append each character to a string individually using the +
operator.
Remember to include the string
header file at the start of the program.
Let us understand this with the syntax.
char arr = ".." string s = "" for (i = 0; i < len_of_arr; ++i) {
s = s + arr[i]
}
We will create a character array and loop over this array using the for
loop, append each character individually to an empty string, and display the final result.
Let us understand this with the example code below.
#include <iostream>
#include <string>
int main() {
int i;
char c_arr[] = "DelftStack";
int len = sizeof(c_arr) / sizeof(char);
std::string str = "";
for (i = 0; i < len; ++i) {
str = str + c_arr[i];
}
std::cout << str;
return 0;
}
In this example, we initialize an integer i
, a character array c_arr
containing the string DelftStack
, and an integer len
to store the length of the character array. The length is calculated using the sizeof
operator, which determines the size of the character array in bytes, divided by the size of a single character.
A std::string
named str
is then initialized as an empty string.
The code enters a for
loop that iterates through each character in the c_arr
array. Inside this loop, each character is appended to the str
string using the +
operator.
Output:
The output above displays the string str
, whose value is DelftStack
after the conversion.
Use the while
Loop and +
Operator to Convert a Character Array to String in C++
We can also use a while
loop similar to the above-discussed method. The while
loop is an exit-controlled loop where the condition is evaluated at the end of the execution.
Let’s use the while
loop in the above example.
#include <iostream>
#include <string>
int main() {
int i = 0;
char c_arr[] = "DelftStack";
int len = sizeof(c_arr) / sizeof(char);
std::string str = "";
while (i < len) {
str = str + c_arr[i];
i = i + 1;
}
std::cout << str;
return 0;
}
In the above example, we use the while
loop instead of the for
loop to carry out the same task as before.
Output:
As we see in the output, the contents of the char array are converted into a string str
, and the contents are displayed.
Notice the slight differences in the code.
First, we initialized the value of the counter variable i
as 0
explicitly before the loop. The increment of this variable is also done explicitly in the loop statements.
The overall program logic is the same as earlier.
Use the string
Constructor to Convert a Character Array to String in C++
In C++, a constructor is a special member function of a class that is automatically called when an object of the class is created.
Constructors are responsible for initializing the object’s data members and setting up the object’s initial state. They have the same name as the class and do not have a return type.
A very simple and direct method is to use the string
constructor and initialize it with the character array. This will directly convert the character array to a string.
Syntax:
char arr = ".." string str = arr
The constructor takes care of the NULL
character at the end of the character array and returns a string object.
Let us see an example.
#include <iostream>
#include <string>
int main() {
char c_arr[] = "DelftStack";
std::string str(c_arr);
std::cout << str;
return 0;
}
The key aspect of this example is the use of the string
constructor that takes a character array c_arr
as an argument. This constructor allows for a direct conversion of the character array to a C++ string in a single line.
Output:
The output displays the final result, which is the value stored in the string str
.
Keep in mind that this conversion creates a copy of the character array, and the resulting std::string
will be null-terminated, just like the original character array.
Conclusion
This tutorial demonstrated different methods to convert a character array to a string in Python. First, we discussed character arrays and how they represent a sequence of characters in C++ similar to the string
objects from the string
class.
We highlighted the differences between character arrays and strings in C++. Two methods were discussed to make this conversion.
In the first method, individual characters are added to a string using the for
loop and the +
operator. We demonstrated how to iterate through an array and use the +
operator for concatenation.
We also demonstrated how the above logic can be implemented using the while
loop, which provides the same logic and output.
In the second method, we use the string
constructor to directly make the conversion. We use constructors in C++ to initialize objects with an initial value.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookRelated Article - C++ Char
- How to Count Occurrences of a Character in String C++
- How to Get ASCII Value of Char in C++
- How to Convert ASCII to Char in C++
- How to Convert String to Char Array in C++
- How to Convert Int to Char Array in C++
Related Article - C++ String
- How to Capitalize First Letter of a String in C++
- How to Find the Longest Common Substring in C++
- How to Find the First Repeating Character in a String in C++
- How to Compare String and Character in C++
- How to Get the Last Character From a String in C++
- How to Remove Last Character From a String in C++