How to Count Occurrences of a Character in String C++
- Use a Loop With to Count Occurrences of a Character in a String
-
Use the
std::tolower
to Count Character Occurrences Regardless of Letter Case -
Use
std::count
to Count Occurrences of a Character in a String - Conclusion
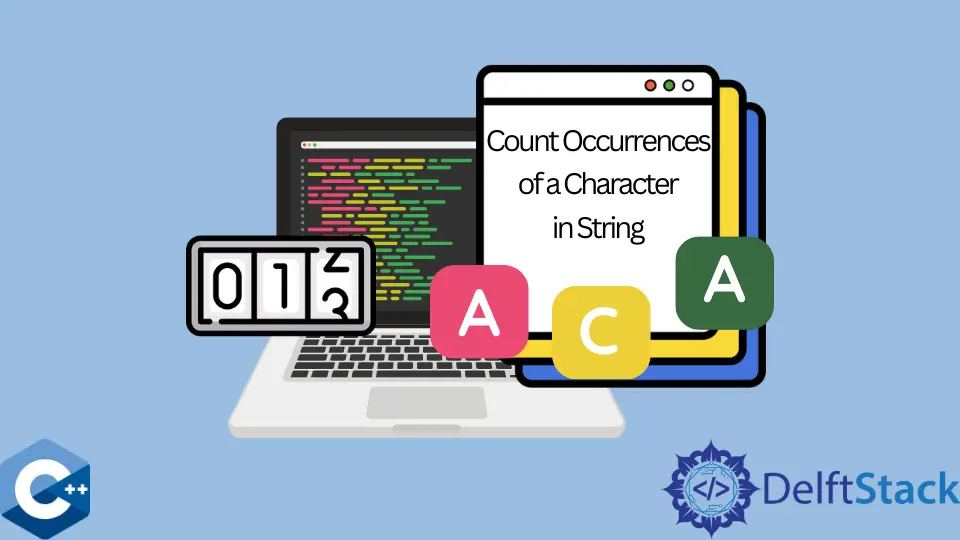
Counting occurrences of a character in a string is a common task in C++ programming, often required in text processing and data analysis. This operation involves determining the frequency with which a specific character appears within a given string.
C++ provides several approaches to achieve this, and each method has its advantages, allowing developers to choose an approach that aligns with their coding style and specific application needs. This article will explain several methods of how to count occurrences of a character in a string C++.
Use a Loop With to Count Occurrences of a Character in a String
In this method, we employ a loop in C++ to iterate through each character in a given string. The goal is to count the occurrences of a specific character.
This method involves defining a loop that traverses the characters of the string, and for each character, it checks whether it matches the specified character for counting. This manual counting technique using a loop provides a basic and flexible way to achieve the task of counting character occurrences in a string.
Code Example:
#include <iostream>
#include <string>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::string;
size_t countOccurrences(char c, string &str) {
size_t count = 0;
for (char i : str)
if (i == c) count++;
return count;
}
int main() {
char ch1 = 'e';
char ch2 = 'h';
string str1("hello there, how are you doing?");
string str2("Hello there! How are you doing?");
cout << "Occurrences of '" << ch1
<< "' in str1 = " << countOccurrences(ch1, str1) << endl;
cout << "Occurrences of '" << ch2
<< "' in str2 = " << countOccurrences(ch2, str2) << endl;
exit(EXIT_SUCCESS);
}
In this code, we define a function called countOccurrences
, which takes a character and a string as parameters and returns the count of occurrences of that character in the given string. Inside this function, we use a loop to iterate through each character in the string, and if the character matches the specified one, we increment the count.
Output:
Occurrences of 'e' in str1 = 4
Occurrences of 'h' in str2 = 1
The output showcases the counts of 'e'
in str1
and 'h'
in str2
. For the provided strings, the output indicates that 'e'
appears 4
times in str1
, while 'h'
appears 1
time in str2
.
Use the std::tolower
to Count Character Occurrences Regardless of Letter Case
Similar to the first method, this approach focuses on counting character occurrences in a string. However, in this case, we specifically leverage the std::tolower
function to achieve a case-insensitive count.
The function is applied to both the target character and each character in the string, ensuring a uniform comparison in terms of letter case. This method is valuable when case sensitivity is not desired and a total count of occurrences, irrespective of letter case, is needed.
The basic syntax of std::tolower
:
int tolower(int c);
The std::tolower
function is part of the <cctype>
header in C++, and it takes an integer argument c
, which represents the character to be converted to lowercase. The function returns the lowercase equivalent of the input character if it is an uppercase letter; otherwise, it returns the input character unchanged.
In the following code, we use the std::tolower
function to achieve a case-insensitive count.
Code Example:
#include <iostream>
#include <string>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::string;
size_t countOccurrences(char c, string &str) {
size_t count = 0;
char tmp = static_cast<char>(tolower(static_cast<unsigned char>(c)));
for (char i : str)
if (tolower(static_cast<unsigned char>(i)) == tmp) count++;
return count;
}
int main() {
char ch1 = 'e';
char ch2 = 'h';
string str1("hello there, how are you doing?");
string str2("Hello there! How are you doing?");
cout << "Occurrences of '" << ch1
<< "' in str1 = " << countOccurrences(ch1, str1) << endl;
cout << "Occurrences of '" << ch2
<< "' in str2 = " << countOccurrences(ch2, str2) << endl;
exit(EXIT_SUCCESS);
}
In this code, we create a program that counts the occurrences of specific characters in two strings while ignoring the case. The countOccurrences
function is designed to achieve case-insensitive character counting.
Within the function, we convert both the target character and each character in the string to lowercase using the std::tolower
function. This ensures that the comparison is performed uniformly, regardless of the original letter case.
Output:
Occurrences of 'e' in str1 = 4
Occurrences of 'h' in str2 = 3
The output showcases the counts of 'e'
in str1
and 'h'
in str2
while ignoring the letter case. For the provided strings, the output indicates that 'e'
appears 4
times in str1
, and 'h'
appears 3
times in str2
.
Use std::count
to Count Occurrences of a Character in a String
In this method, we utilize the std::count
algorithm provided by the C++ Standard Template Library (STL). The std::count
function is designed to efficiently count the occurrences of a specified value within a given range, such as the characters in a string.
This approach is concise and expressive, offering a straightforward solution for counting occurrences without the need for manual iteration. It’s a preferred choice when leveraging standard algorithms for a clean and efficient implementation.
The basic syntax of std::count
:
size_t std::count(ForwardIt first, ForwardIt last, const T& value);
The syntax involves specifying the range using iterators (first
and last
), the data type (T
), and the target value (value
). This algorithm provides an efficient and concise way to count occurrences without requiring manual iteration.
Code Example:
#include <algorithm>
#include <iostream>
#include <string>
size_t countOccurrencesUsingAlgorithms(char c, const std::string& str) {
return std::count(str.begin(), str.end(), c);
}
int main() {
char ch1 = 'e';
char ch2 = 'h';
std::string str1("hello there, how are you doing?");
std::string str2("Hello there! How are you doing?");
std::cout << "Occurrences of '" << ch1
<< "' in str1: " << countOccurrencesUsingAlgorithms(ch1, str1)
<< std::endl;
std::cout << "Occurrences of '" << ch2
<< "' in str2: " << countOccurrencesUsingAlgorithms(ch2, str2)
<< std::endl;
return 0;
}
In this code, we count the occurrences of specific characters in two strings using the std::count
algorithm from the C++ Standard Template Library (STL). The countOccurrencesUsingAlgorithms
function takes a character and a string as parameters and utilizes the std::count
function to efficiently count the occurrences of that character in the given string.
Output:
Occurrences of 'e' in str1: 4
Occurrences of 'h' in str2: 1
The output of the program showcases the counts of 'e'
in str1
and 'h'
in str2
. For the provided strings, the output indicates that 'e'
appears 4
times in str1
, and 'h'
appears 1
time in str2
.
Conclusion
This article explores three methods in C++ for counting occurrences of a character in a string. The first method employs a manual counting loop that iterates through the string, providing a flexible yet basic approach.
The second method extends this by incorporating std::tolower
for a case-insensitive count, useful when case sensitivity is not required. The third method leverages the std::count
algorithm from the C++ STL, offering a concise and efficient solution without manual iteration.
Developers can choose the method that best aligns with their coding style and application needs, considering factors such as case sensitivity and efficiency. The provided code examples and outputs demonstrate the versatility of each approach.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook