C++ 中计算字符串一个字符的出现次数
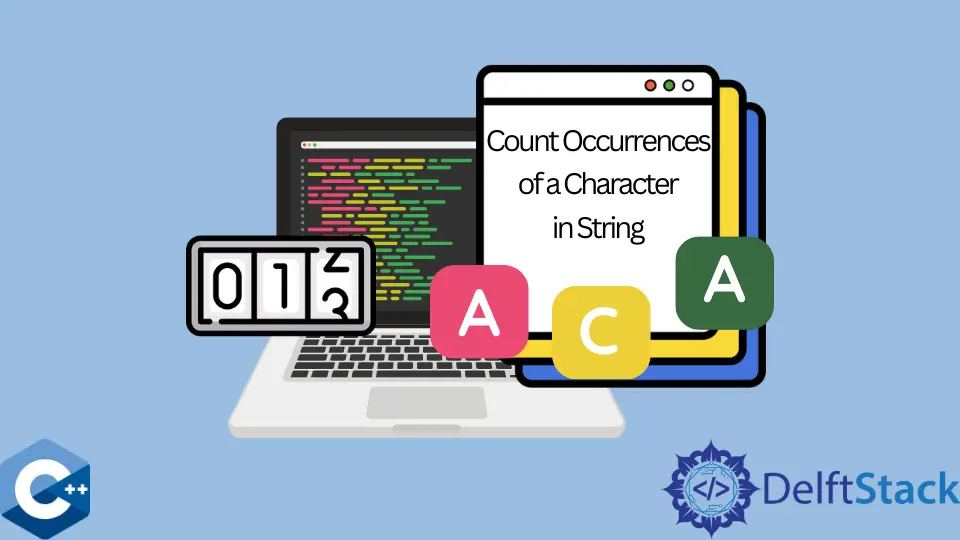
本文将解释如何在 C++ 字符串中计算一个字符的出现次数的几种方法。
使用迭代方法计算字符串中字符的出现次数
C++ 字符串库提供了一个 std::string
类,该类可用于存储和操作类似于 char
的对象序列。它提供了多种搜索和查找给定字符或字符串中子字符串的方法,但是在这种情况下,我们需要检查每个字符,因此实现在增加 count
变量的同时迭代字符串的函数将更加简单。该函数将一个字符串对象作为参考,并使用一个字符作为值来计数出现次数。请注意,此版本无法区分大写字母和小写字母,因此同时包含大小写字母时,该方法无法使用。
#include <iostream>
#include <string>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::string;
size_t countOccurrences(char c, string &str) {
size_t count = 0;
for (char i : str)
if (i == c) count++;
return count;
}
int main() {
char ch1 = 'e';
char ch2 = 'h';
string str1("hello there, how are you doing?");
string str2("Hello there! How are you doing?");
cout << "number of char - '" << ch1
<< "' occurrences = " << countOccurrences(ch1, str1) << endl;
cout << "number of char - '" << ch2
<< "' occurrences = " << countOccurrences(ch2, str2) << endl;
exit(EXIT_SUCCESS);
}
输出:
number of char - 'e' occurrences = 4
number of char - 'h' occurrences = 1
使用 std::tolower
函数来计算字母大小写的字符出现次数
实现上述示例的另一种方法是重写 countOccurrences
函数,以在每次迭代时比较字符的转换值。注意,std::tolower
很难使用,因为它要求唯一的 char
参数可以表示为 unsigned char
。否则,行为是不确定的。因此,我们将强制转换的 unsigned char
值传递给 tolower
函数,然后将其强制转换回 char
以存储到临时变量 tmp
中。另外,我们在每次迭代时都调用 tolower
函数,以将字符串字符转换为比较语句的小写字母。请注意,以下代码示例可以区分大写字母和小写字母,并对它们全部进行计数。
#include <iostream>
#include <string>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::string;
size_t countOccurrences(char c, string &str) {
size_t count = 0;
char tmp = static_cast<char>(tolower(static_cast<unsigned char>(c)));
for (char i : str)
if (tolower(static_cast<unsigned char>(i)) == tmp) count++;
return count;
}
int main() {
char ch1 = 'e';
char ch2 = 'h';
string str1("hello there, how are you doing?");
string str2("Hello there! How are you doing?");
cout << "number of char - '" << ch1
<< "' occurrences = " << countOccurrences(ch1, str1) << endl;
cout << "number of char - '" << ch2
<< "' occurrences = " << countOccurrences(ch2, str2) << endl;
exit(EXIT_SUCCESS);
}
输出:
number of char - 'e' occurrences = 4
number of char - 'h' occurrences = 3
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Jinku Hu