在 C++ 中获取字符的 ASCII 值
-
使用
std::copy
和std::ostream_iterator
来获取char
的 ASCII 值 -
使用
printf
格式说明符来获取字符的 ASCII 值 -
使用
int()
获得字符的 ASCII 值
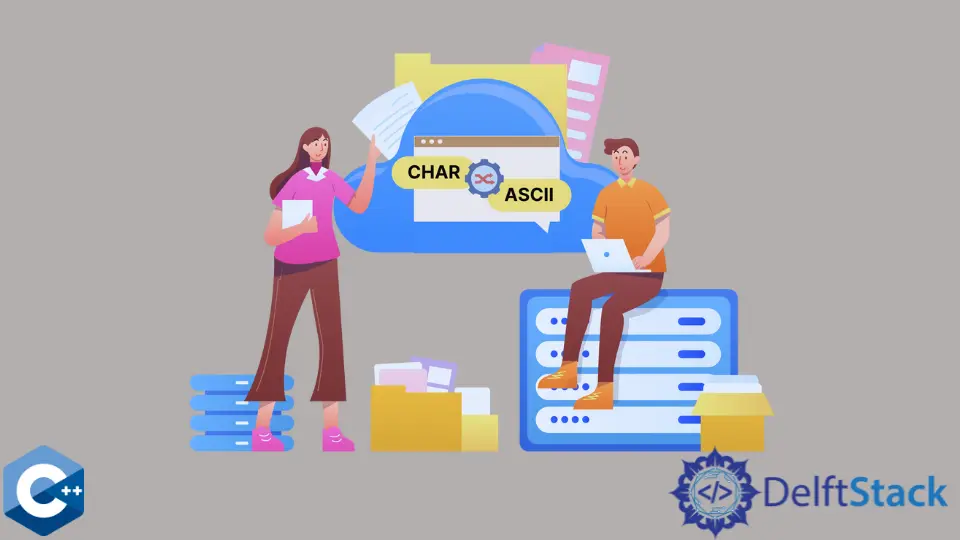
本文将介绍几种如何在 C++ 中获取字符的 ASCII 值的方法。
使用 std::copy
和 std::ostream_iterator
来获取 char
的 ASCII 值
尽管自那时以来出现了较新的标准方案,如 UTF-8 等,ASCII 字符编码在计算机中几乎无处不在。最初,ASCII 编码的英文字母,十进制数字,标点符号和一些其他控制代码。所有这些符号都使用 [0 - 127]
范围内的某个整数值表示。由于 char
类型被实现为整数,因此我们可以使用 ostream_iterator<int>
和 std::copy
算法将其值输出到 cout
流中。请注意,随后的字母具有相邻的数字值。
#include <iostream>
#include <iterator>
#include <vector>
using std::copy;
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<char> chars{'g', 'h', 'T', 'U', 'q', '%', '+', '!', '1', '2', '3'};
cout << "chars: ";
std::copy(chars.begin(), chars.end(), std::ostream_iterator<int>(cout, "; "));
return EXIT_SUCCESS;
}
输出:
chars: 103; 104; 84; 85; 113; 37; 43; 33; 49; 50; 51;
使用 printf
格式说明符来获取字符的 ASCII 值
printf
函数是输出带有相应 ASCII 码的字符值的另一种选择。注意,printf
将两个参数都作为 char
类型,并且仅区分格式说明符%c
/%d
。尽管后面的说明符通常通常分别记录为 char
和 int
格式化程序,但它们彼此兼容。
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<char> chars{'g', 'h', 'T', 'U', 'q', '%', '+', '!', '1', '2', '3'};
cout << endl;
for (const auto &number : chars) {
printf("The ASCII value of '%c' is: %d\n", number, number);
}
return EXIT_SUCCESS;
}
输出:
The ASCII value of 'g' is: 103
The ASCII value of 'h' is: 104
The ASCII value of 'T' is: 84
The ASCII value of 'U' is: 85
The ASCII value of 'q' is: 113
The ASCII value of '%' is: 37
The ASCII value of '+' is: 43
The ASCII value of '!' is: 33
The ASCII value of '1' is: 49
The ASCII value of '2' is: 50
The ASCII value of '3' is: 51
使用 int()
获得字符的 ASCII 值
最后,可以使用 int(c)
表示法转换 char
值,并将这些值作为任何其他变量直接输出到 cout
流。请注意,此示例比带有格式说明符的 printf
版本具有更多的 C++ 风格。相反,可以使用 char(i)
标记从 int
值打印 ASCII 字符值。
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<char> chars{'g', 'h', 'T', 'U', 'q', '%', '+', '!', '1', '2', '3'};
cout << endl;
for (auto &number : chars) {
cout << "The ASCII value of '" << number << "' is: " << int(number) << endl;
}
return EXIT_SUCCESS;
}
输出:
The ASCII value of 'g' is: 103
The ASCII value of 'h' is: 104
The ASCII value of 'T' is: 84
The ASCII value of 'U' is: 85
The ASCII value of 'q' is: 113
The ASCII value of '%' is: 37
The ASCII value of '+' is: 43
The ASCII value of '!' is: 33
The ASCII value of '1' is: 49
The ASCII value of '2' is: 50
The ASCII value of '3' is: 51
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Jinku Hu