C++ で Char の ASCII 値を取得する
-
std::copy
とstd::ostream_iterator
を使用して、char
の ASCII 値を取得する -
printf
フォーマット指定子を使用して、char
の ASCII 値を取得する -
int()
を使用して、char
の ASCII 値を取得する
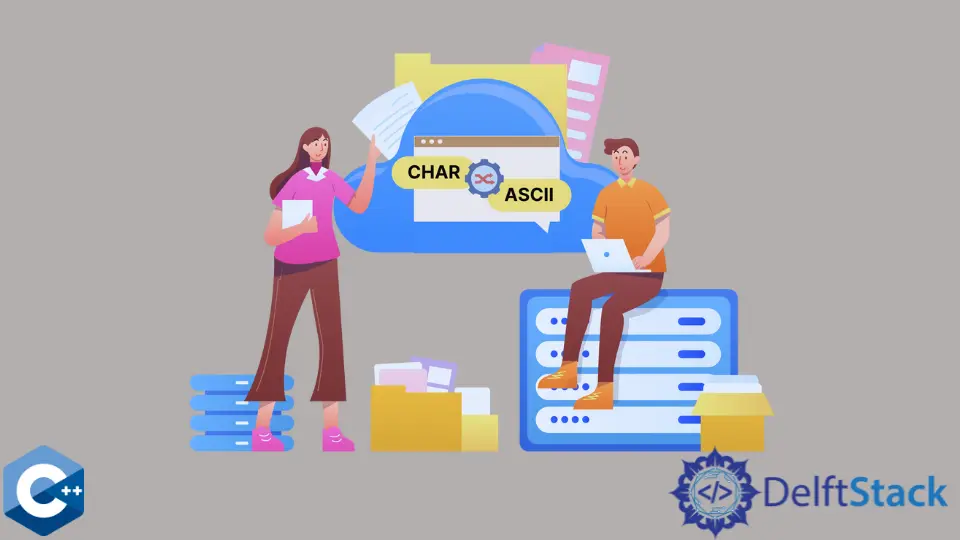
この記事では、C++ で char
の ASCII 値を取得する方法のいくつかの方法について説明します。
std::copy
と std::ostream_iterator
を使用して、char
の ASCII 値を取得する
それ以来、UTF-8 などの新しい標準スキームが登場したにもかかわらず、ASCII 文字エンコードはコンピュータにほぼ遍在しています。元々、ASCII でエンコードされた英字、10 進数、句読記号、およびいくつかの追加の制御コード。これらの記号はすべて、[0 - 127]
の範囲の整数値を使用して表されます。char
タイプは整数として実装されているため、ostream_iterator<int>
および std::copy
アルゴリズムを使用して、その値を処理し、cout
ストリームに出力できます。結果として生じるアルファベットには、隣接する数値があることに注意してください。
#include <iostream>
#include <iterator>
#include <vector>
using std::copy;
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<char> chars{'g', 'h', 'T', 'U', 'q', '%', '+', '!', '1', '2', '3'};
cout << "chars: ";
std::copy(chars.begin(), chars.end(), std::ostream_iterator<int>(cout, "; "));
return EXIT_SUCCESS;
}
出力:
chars: 103; 104; 84; 85; 113; 37; 43; 33; 49; 50; 51;
printf
フォーマット指定子を使用して、char
の ASCII 値を取得する
printf
関数は、対応する ASCII コードで char
値を出力するためのもう 1つの代替手段です。printf
は両方の引数を char
タイプとして受け取り、フォーマット指定子%c
/%d
のみを区別することに注意してください。後者の指定子は通常、それぞれ char
および int
フォーマッターとして文書化されていますが、それらは相互のタイプと互換性があります。
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<char> chars{'g', 'h', 'T', 'U', 'q', '%', '+', '!', '1', '2', '3'};
cout << endl;
for (const auto &number : chars) {
printf("The ASCII value of '%c' is: %d\n", number, number);
}
return EXIT_SUCCESS;
}
出力:
The ASCII value of 'g' is: 103
The ASCII value of 'h' is: 104
The ASCII value of 'T' is: 84
The ASCII value of 'U' is: 85
The ASCII value of 'q' is: 113
The ASCII value of '%' is: 37
The ASCII value of '+' is: 43
The ASCII value of '!' is: 33
The ASCII value of '1' is: 49
The ASCII value of '2' is: 50
The ASCII value of '3' is: 51
int()
を使用して、char
の ASCII 値を取得する
最後に、int(c)
表記を使用して char
値を変換し、他の変数と同様に値を cout
ストリームに直接出力できます。この例は、フォーマット指定子を備えた printf
バージョンよりも C++ スタイルであることに注意してください。対照的に、ASCII 文字値は、char(i)
表記を使用して int
値から出力できます。
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<char> chars{'g', 'h', 'T', 'U', 'q', '%', '+', '!', '1', '2', '3'};
cout << endl;
for (auto &number : chars) {
cout << "The ASCII value of '" << number << "' is: " << int(number) << endl;
}
return EXIT_SUCCESS;
}
出力:
The ASCII value of 'g' is: 103
The ASCII value of 'h' is: 104
The ASCII value of 'T' is: 84
The ASCII value of 'U' is: 85
The ASCII value of 'q' is: 113
The ASCII value of '%' is: 37
The ASCII value of '+' is: 43
The ASCII value of '!' is: 33
The ASCII value of '1' is: 49
The ASCII value of '2' is: 50
The ASCII value of '3' is: 51
チュートリアルを楽しんでいますか? <a href="https://www.youtube.com/@delftstack/?sub_confirmation=1" style="color: #a94442; font-weight: bold; text-decoration: underline;">DelftStackをチャンネル登録</a> して、高品質な動画ガイドをさらに制作するためのサポートをお願いします。 Subscribe
著者: 胡金庫