C++에서 Char의 ASCII 값 가져 오기
-
std::copy
및std::ostream_iterator
를 사용하여char
의 ASCII 값을 가져옵니다 -
printf
형식 지정자를 사용하여char
의 ASCII 값 가져 오기 -
int()
를 사용하여char
의 ASCII 값 가져 오기
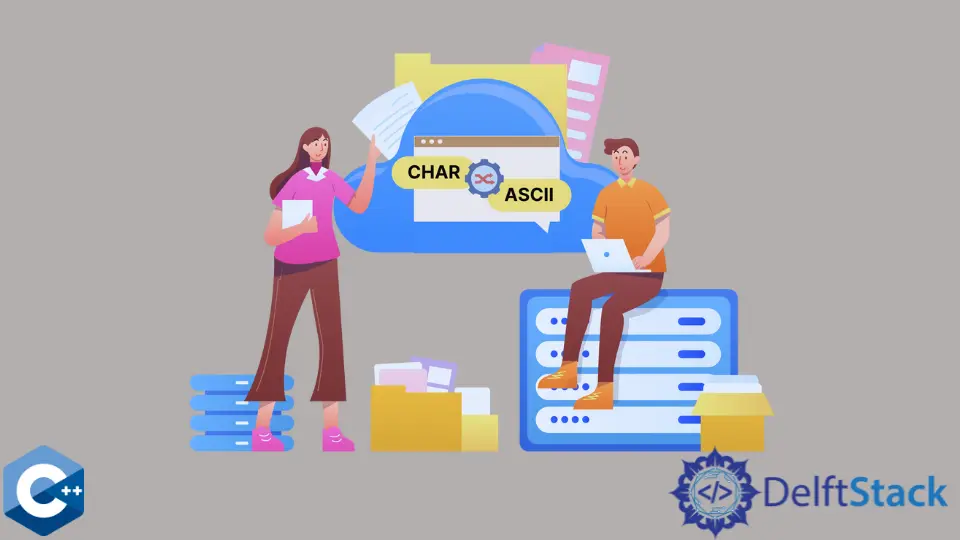
이 기사에서는 C++에서char
의 ASCII 값을 얻는 방법에 대한 몇 가지 방법을 설명합니다.
std::copy
및std::ostream_iterator
를 사용하여char
의 ASCII 값을 가져옵니다
ASCII 문자 인코딩은 UTF-8 및 기타와 같은 새로운 표준 체계가 그 이후로 등장했지만 컴퓨터에서 거의 어디에나 있습니다. 원래 ASCII로 인코딩 된 영어 문자, 십진수, 구두점 기호 및 일부 추가 제어 코드입니다. 이러한 모든 기호는[0 - 127]
범위의 정수 값을 사용하여 표시됩니다. char
유형이 정수로 구현되었으므로 해당 값을 처리하고ostream_iterator<int>
및std::copy
알고리즘을 사용하여cout
스트림에 출력 할 수 있습니다. 결과적으로 나오는 알파벳 문자에는 인접한 숫자 값이 있습니다.
#include <iostream>
#include <iterator>
#include <vector>
using std::copy;
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<char> chars{'g', 'h', 'T', 'U', 'q', '%', '+', '!', '1', '2', '3'};
cout << "chars: ";
std::copy(chars.begin(), chars.end(), std::ostream_iterator<int>(cout, "; "));
return EXIT_SUCCESS;
}
출력:
chars: 103; 104; 84; 85; 113; 37; 43; 33; 49; 50; 51;
printf
형식 지정자를 사용하여char
의 ASCII 값 가져 오기
printf
함수는 해당 ASCII 코드와 함께char
값을 출력하는 또 다른 대안입니다. printf
는 두 인수를char
유형으로 취하고 형식 지정자%c
/%d
만 구별합니다. 후자의 지정자는 일반적으로 각각char
및int
포맷터로 문서화되지만 서로의 유형과 호환됩니다.
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<char> chars{'g', 'h', 'T', 'U', 'q', '%', '+', '!', '1', '2', '3'};
cout << endl;
for (const auto &number : chars) {
printf("The ASCII value of '%c' is: %d\n", number, number);
}
return EXIT_SUCCESS;
}
출력:
The ASCII value of 'g' is: 103
The ASCII value of 'h' is: 104
The ASCII value of 'T' is: 84
The ASCII value of 'U' is: 85
The ASCII value of 'q' is: 113
The ASCII value of '%' is: 37
The ASCII value of '+' is: 43
The ASCII value of '!' is: 33
The ASCII value of '1' is: 49
The ASCII value of '2' is: 50
The ASCII value of '3' is: 51
int()
를 사용하여char
의 ASCII 값 가져 오기
마지막으로int(c)
표기법을 사용하여char
값을 변환하고 값을 다른 변수로cout
스트림에 직접 출력 할 수 있습니다. 이 예제는 형식 지정자가있는printf
버전보다 더 C++ 스타일입니다. 반대로 ASCII 문자 값은char(i)
표기법을 사용하여int
값에서 인쇄 할 수 있습니다.
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<char> chars{'g', 'h', 'T', 'U', 'q', '%', '+', '!', '1', '2', '3'};
cout << endl;
for (auto &number : chars) {
cout << "The ASCII value of '" << number << "' is: " << int(number) << endl;
}
return EXIT_SUCCESS;
}
출력:
The ASCII value of 'g' is: 103
The ASCII value of 'h' is: 104
The ASCII value of 'T' is: 84
The ASCII value of 'U' is: 85
The ASCII value of 'q' is: 113
The ASCII value of '%' is: 37
The ASCII value of '+' is: 43
The ASCII value of '!' is: 33
The ASCII value of '1' is: 49
The ASCII value of '2' is: 50
The ASCII value of '3' is: 51
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook