C++에서 문자열을 문자 배열로 변환하는 방법
- std::basic_string::c_str 메서드를 사용하여 문자열을 Char 배열로 변환
- std::vector 컨테이너를 사용하여 문자열을 Char 배열로 변환
- 포인터 조작 작업을 사용하여 문자열을 문자 배열로 변환
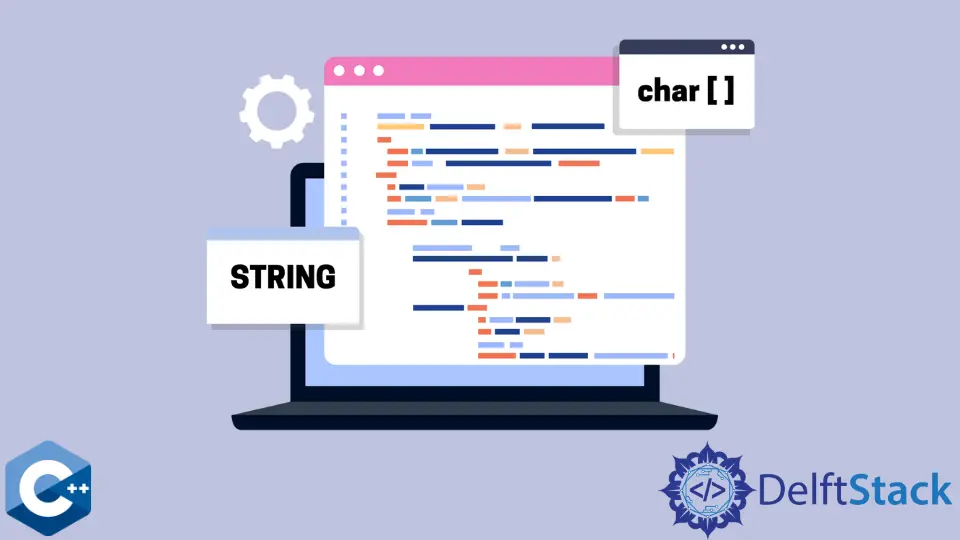
본 기사는 문자열 데이터를 char 배열로 변환하는 여러 가지 방법을 소개하고 있다.
std::basic_string::c_str 메서드를 사용하여 문자열을 Char 배열로 변환
이 버전은 위의 문제를 해결하는 C++ 방법이다. 그것은 문자열이 없는 문자열에 포인터를 돌려주는 문자열 builtin 방식 c_str을 사용한다.
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl using std::string;
int main() {
string tmp_string = "This will be converted to char*";
auto c_string = tmp_string.c_str();
cout << c_string << endl;
return EXIT_SUCCESS;
}
출력:
This will be converted to char*
c_str()
방법으로 반환된 포인터에 저장된 데이터를 수정하려면 먼저 memmove 기능을 사용하여 범위를 다른 위치에 복사한 다음 다음과 같이 문자열을 조작해야 한다.
#include <cstring>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl using std::string;
int main() {
string tmp_string = "This will be converted to char*";
char *c_string_copy = new char[tmp_string.length() + 1];
memmove(c_string_copy, tmp_string.c_str(), tmp_string.length());
/* do operations on c_string_copy here */
cout << c_string_copy;
delete[] c_string_copy;
return EXIT_SUCCESS;
}
다음과 같은 다양한 함수를 사용하여c_string
데이터를 복사 할 수 있습니다. memcpy
, memccpy
, mempcpy
, strcpy
또는 strncpy
, 매뉴얼 페이지를 읽고 가장자리 사례/버그를주의 깊게 고려하십시오.
std::vector 컨테이너를 사용하여 문자열을 Char 배열로 변환
또 다른 방법은 문자열을 벡터 용기에 저장한 다음 강력한 내장 방식을 사용하여 데이터를 안전하게 조작하는 것이다.
#include <iostream>
#include <iterator>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
int main() {
string tmp_string = "This will be converted to char*\n";
vector<char> vec_str(tmp_string.begin(), tmp_string.end());
std::copy(vec_str.begin(), vec_str.end(),
std::ostream_iterator<char>(cout, ""));
return EXIT_SUCCESS;
}
포인터 조작 작업을 사용하여 문자열을 문자 배열로 변환
이 버전에서는 tmp_ptr라는 이름의 char 포인터를 정의하고 첫 번째 문자의 주소를 tmp_string으로 지정한다.
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl using std::string;
int main() {
string tmp_string = "This will be converted to char*\n";
string mod_string = "I've been overwritten";
char *tmp_ptr = &tmp_string[0];
cout << tmp_ptr;
return EXIT_SUCCESS;
}
단, tmp_ptr
에서 데이터를 수정하면 tmp_string
값도 변경되므로 다음과 같은 코드 샘플을 실행하면 알 수 있다.
#include <cstring>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl using std::string;
int main() {
string tmp_string = "This will be converted to char*\n";
string mod_string = "I've been overwritten";
char *tmp_ptr = &tmp_string[0];
memmove(tmp_ptr, mod_string.c_str(), mod_string.length());
cout << tmp_string;
return EXIT_SUCCESS;
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook관련 문장 - C++ String
- C++에서 가장 긴 공통 하위 문자열 찾기
- C++에서 문자열의 첫 글자를 대문자로
- C++에서 문자열의 첫 번째 반복 문자 찾기
- C++에서 문자열과 문자 비교
- C++의 문자열에서 마지막 문자 제거
- C++의 문자열에서 마지막 문자 가져오기