C++에서 Int를 Char Array로 변환하는 방법
-
std::sprintf 기능을 사용하여
int
를char*
로 변환 - to_string()과 c_str()의 int를 char*로 변환하는 방법의 조합
- 변환에 std::stringstream 클래스 방법 사용
- std::to_chars 기능을 사용하여 “int"를 “char*“로 변환
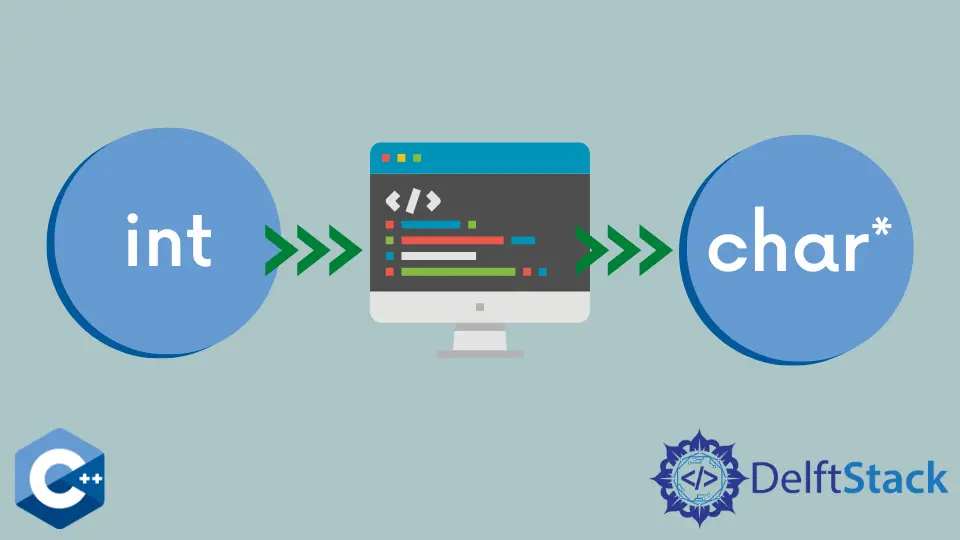
이 글에서는 int를 char 어레이(char*
)로 변환하는 방법에 대해 설명한다.
다음 예에서는 변환 인메모리 버퍼의 출력을 저장한다고 가정하고, 검증을 위해 std::printf
로 결과를 출력한다.
std::sprintf 기능을 사용하여 int
를 char*
로 변환
우선 char 버퍼로 변환할 단일 int 변수를 저장하기 위해 공간을 할당해야 한다. 다음 예제는 정수 데이터에 대한 최대 길이 MAX_DIGITS
를 정의하는 것에 주목하십시오. char 버퍼 길이를 계산하기 위해 sprintf 함수는 목적지에서 자동으로 종료되는 char 문자열을 쓰기 때문에 sizeof(char)를 추가한다. 그러므로 우리는 이 완충재를 위해 충분한 공간을 할당하는 것을 꺼려해야 한다.
#include <iostream>
#define MAX_DIGITS 10
int main() {
int number = 1234;
char num_char[MAX_DIGITS + sizeof(char)];
std::sprintf(num_char, "%d", number);
std::printf("num_char: %s \n", num_char);
return 0;
}
출력:
num_char: 1234
sprintf
는 sprintf(buf, “%s some text to add”, buf 등)의 동작이 정의되지 않고 컴파일러에 따라 결과가 잘못되기 때문에 호출하지 않는 것이 좋다.
to_string()과 c_str()의 int를 char*로 변환하는 방법의 조합
이 버전은 std::string 등급 방식을 사용하여 변환을 수행하므로 앞의 예와 같이 sprintf를 처리하는 것보다 훨씬 안전한 버전이다.
#include <iostream>
int main() {
int number = 1234;
std::string tmp = std::to_string(number);
char const *num_char = tmp.c_str();
printf("num_char: %s \n", num_char);
return 0;
}
변환에 std::stringstream 클래스 방법 사용
std::stringstream 클래스를 사용하여 구현한다. 즉, int
데이터를 저장한 다음 str 방식으로 문자열 객체를 반환하고 최종적으로 c_str을 호출하여 변환하는 임시 문자열 기반 스트림을 만든다.
#include <iostream>
#include <sstream>
int main() {
int number = 1234;
std::stringstream tmp;
tmp << number;
char const *num_char = tmp.str().c_str();
printf("num_char: %s \n", num_char);
;
return 0;
}
std::to_chars 기능을 사용하여 “int"를 “char*“로 변환
이 버전은 C++17에 추가된 순수한 C++ 스타일 함수로서 헤더 <charconv>
에 정의되어 있다. 긍정적인 측면에서는, 이 방법은 특정 시나리오에서 가장 유연한 솔루션이 될 수 있는 범위에 대한 운영을 제공한다.
#include <charconv>
#include <iostream>
#define MAX_DIGITS 10
int main() {
int number = 1234;
char num_char[MAX_DIGITS + sizeof(char)];
std::to_chars(num_char, num_char + MAX_DIGITS, number);
std::printf("num_char: %s \n", num_char);
return 0;
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook관련 문장 - C++ Integer
- C++에서 128비트 정수 사용
- C++에서 64비트 정수 지정
- C++의 큰 정수
- C++의 부호 있는 정수 표현식과 부호 없는 정수 표현식 비교
- C++에서 숫자가 소수인지 확인
- C++에서 숫자의 자릿수 계산