Converti Int in Char Array in C++
-
Usa la funzione
std::sprintf
per convertireint
inchar*
-
Combina i metodi
to_string()
ec_str()
per convertireint
inchar*
-
Usa i metodi di classe
std::stringstream
per la conversione -
Usa la funzione
std::to_chars
per convertireint
inchar*
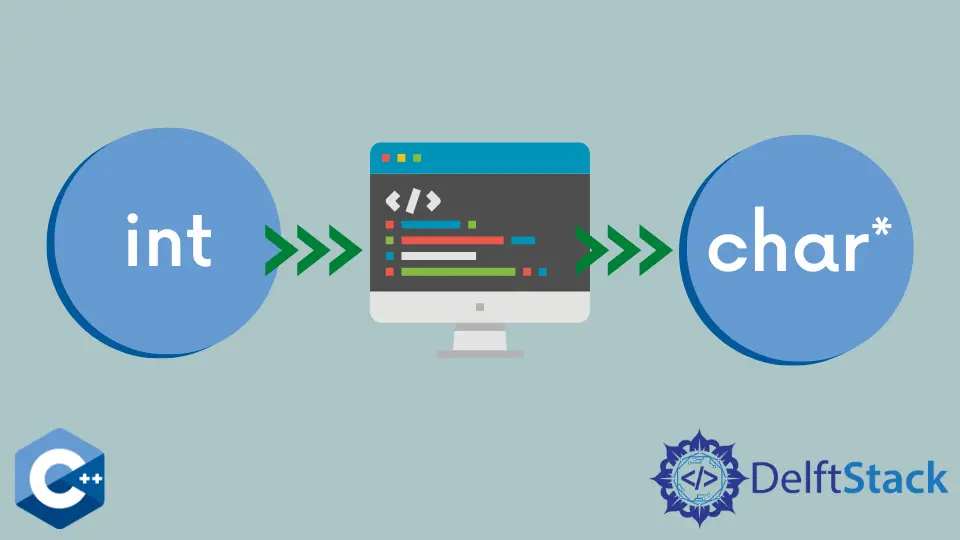
Questo articolo spiegherà come convertire int
in un char array
(char*
) usando metodi diversi.
Nei seguenti esempi, supponiamo di memorizzare l’output del buffer di memoria di conversione e, per scopi di verifica, restituiremo il risultato con std::printf
.
Usa la funzione std::sprintf
per convertire int
in char*
Per prima cosa, dobbiamo allocare spazio per memorizzare una singola variabile int
che convertiremo in un buffer char
. Notare che il seguente esempio definisce la lunghezza massima MAX_DIGITS
per i dati interi. Per calcolare la lunghezza del buffer di caratteri, aggiungiamo sizeof(char)
perché la funzione sprintf
scrive automaticamente la stringa di caratteri che termina il byte \0
nella destinazione. Quindi dovremmo occuparci di allocare abbastanza spazio per questo buffer.
#include <iostream>
#define MAX_DIGITS 10
int main() {
int number = 1234;
char num_char[MAX_DIGITS + sizeof(char)];
std::sprintf(num_char, "%d", number);
std::printf("num_char: %s \n", num_char);
return 0;
}
Produzione:
num_char : 1234
Nota che chiamare sprintf
con buffer sorgente e destinazione sovrapposti (es. sprintf(buf, "%s some text to add", buf)
) non è raccomandato in quanto ha un comportamento indefinito e produrrà risultati errati a seconda del compilatore.
Combina i metodi to_string()
e c_str()
per convertire int
in char*
Questa versione utilizza i metodi della classe std::string
per eseguire la conversione, rendendola una versione molto più sicura rispetto a sprintf
come mostrato nell’esempio precedente.
#include <iostream>
int main() {
int number = 1234;
std::string tmp = std::to_string(number);
char const *num_char = tmp.c_str();
printf("num_char: %s \n", num_char);
return 0;
}
Usa i metodi di classe std::stringstream
per la conversione
Questo metodo è implementato usando la classe std::stringstream
. Vale a dire, creiamo un flusso temporaneo basato su stringhe in cui memorizzare i dati int
, quindi restituire l’oggetto stringa con il metodo str
e infine chiamare c_str
per eseguire la conversione.
#include <iostream>
#include <sstream>
int main() {
int number = 1234;
std::stringstream tmp;
tmp << number;
char const *num_char = tmp.str().c_str();
printf("num_char: %s \n", num_char);
;
return 0;
}
Usa la funzione std::to_chars
per convertire int
in char*
Questa versione è una funzione in puro stile C++ aggiunta in C++ 17 e definita nell’intestazione <charconv>
. Tra i lati positivi, questo metodo offre operazioni su intervalli, che potrebbero essere la soluzione più flessibile in scenari specifici.
#include <charconv>
#include <iostream>
#define MAX_DIGITS 10
int main() {
int number = 1234;
char num_char[MAX_DIGITS + sizeof(char)];
std::to_chars(num_char, num_char + MAX_DIGITS, number);
std::printf("num_char: %s \n", num_char);
return 0;
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookArticolo correlato - C++ Integer
- Contare il numero di cifre in un numero in C++
- Controlla se l'input è intero in C++
- Controlla se un numero è primo in C++
- Espressioni macro INT_MAX e INT_MIN in C++
- Analizzare un int da una stringa in C++
- Converti Int in caratteri ASCII in C++