在 C++ 中獲取字元的 ASCII 值
-
使用
std::copy
和std::ostream_iterator
來獲取char
的 ASCII 值 -
使用
printf
格式說明符來獲取字元的 ASCII 值 -
使用
int()
獲得字元的 ASCII 值
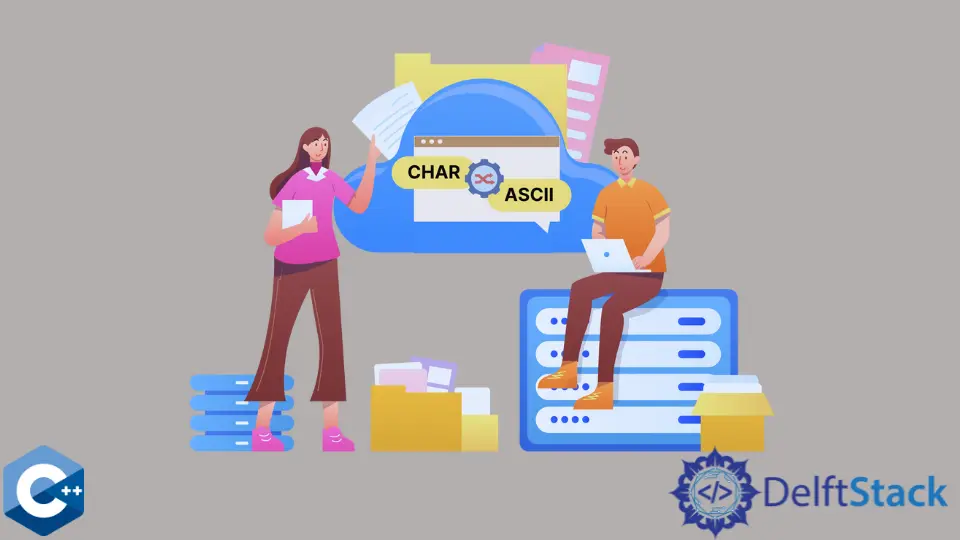
本文將介紹幾種如何在 C++ 中獲取字元的 ASCII 值的方法。
使用 std::copy
和 std::ostream_iterator
來獲取 char
的 ASCII 值
儘管自那時以來出現了較新的標準方案,如 UTF-8 等,ASCII 字元編碼在計算機中幾乎無處不在。最初,ASCII 編碼的英文字母,十進位制數字,標點符號和一些其他控制程式碼。所有這些符號都使用 [0 - 127]
範圍內的某個整數值表示。由於 char
型別被實現為整數,因此我們可以使用 ostream_iterator<int>
和 std::copy
演算法將其值輸出到 cout
流中。請注意,隨後的字母具有相鄰的數字值。
#include <iostream>
#include <iterator>
#include <vector>
using std::copy;
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<char> chars{'g', 'h', 'T', 'U', 'q', '%', '+', '!', '1', '2', '3'};
cout << "chars: ";
std::copy(chars.begin(), chars.end(), std::ostream_iterator<int>(cout, "; "));
return EXIT_SUCCESS;
}
輸出:
chars: 103; 104; 84; 85; 113; 37; 43; 33; 49; 50; 51;
使用 printf
格式說明符來獲取字元的 ASCII 值
printf
函式是輸出帶有相應 ASCII 碼的字元值的另一種選擇。注意,printf
將兩個引數都作為 char
型別,並且僅區分格式說明符%c
/%d
。儘管後面的說明符通常通常分別記錄為 char
和 int
格式化程式,但它們彼此相容。
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<char> chars{'g', 'h', 'T', 'U', 'q', '%', '+', '!', '1', '2', '3'};
cout << endl;
for (const auto &number : chars) {
printf("The ASCII value of '%c' is: %d\n", number, number);
}
return EXIT_SUCCESS;
}
輸出:
The ASCII value of 'g' is: 103
The ASCII value of 'h' is: 104
The ASCII value of 'T' is: 84
The ASCII value of 'U' is: 85
The ASCII value of 'q' is: 113
The ASCII value of '%' is: 37
The ASCII value of '+' is: 43
The ASCII value of '!' is: 33
The ASCII value of '1' is: 49
The ASCII value of '2' is: 50
The ASCII value of '3' is: 51
使用 int()
獲得字元的 ASCII 值
最後,可以使用 int(c)
表示法轉換 char
值,並將這些值作為任何其他變數直接輸出到 cout
流。請注意,此示例比帶有格式說明符的 printf
版本具有更多的 C++ 風格。相反,可以使用 char(i)
標記從 int
值列印 ASCII 字元值。
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<char> chars{'g', 'h', 'T', 'U', 'q', '%', '+', '!', '1', '2', '3'};
cout << endl;
for (auto &number : chars) {
cout << "The ASCII value of '" << number << "' is: " << int(number) << endl;
}
return EXIT_SUCCESS;
}
輸出:
The ASCII value of 'g' is: 103
The ASCII value of 'h' is: 104
The ASCII value of 'T' is: 84
The ASCII value of 'U' is: 85
The ASCII value of 'q' is: 113
The ASCII value of '%' is: 37
The ASCII value of '+' is: 43
The ASCII value of '!' is: 33
The ASCII value of '1' is: 49
The ASCII value of '2' is: 50
The ASCII value of '3' is: 51
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Jinku Hu