C++ 中計算字串一個字元的出現次數
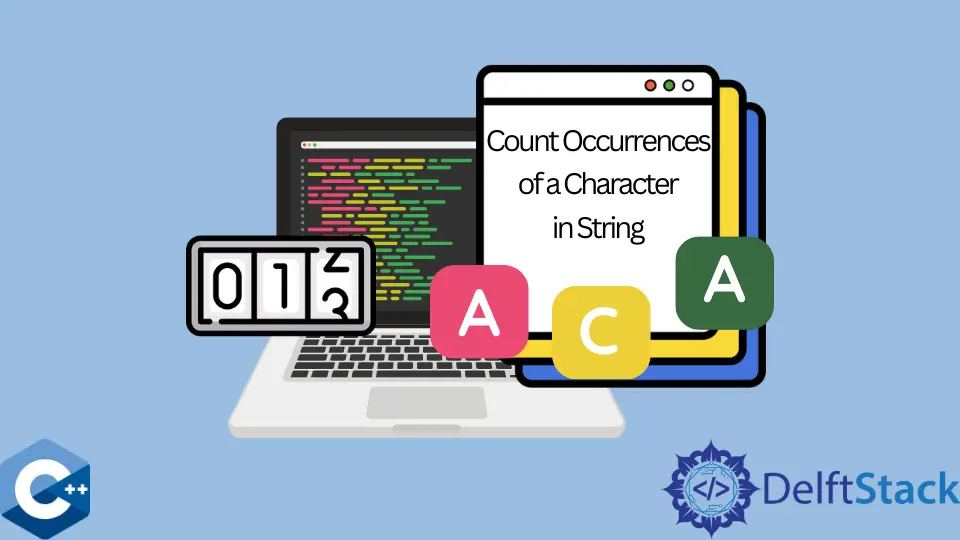
本文將解釋如何在 C++ 字串中計算一個字元的出現次數的幾種方法。
使用迭代方法計算字串中字元的出現次數
C++ 字串庫提供了一個 std::string
類,該類可用於儲存和操作類似於 char
的物件序列。它提供了多種搜尋和查詢給定字元或字串中子字串的方法,但是在這種情況下,我們需要檢查每個字元,因此實現在增加 count
變數的同時迭代字串的函式將更加簡單。該函式將一個字串物件作為參考,並使用一個字元作為值來計數出現次數。請注意,此版本無法區分大寫字母和小寫字母,因此同時包含大小寫字母時,該方法無法使用。
#include <iostream>
#include <string>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::string;
size_t countOccurrences(char c, string &str) {
size_t count = 0;
for (char i : str)
if (i == c) count++;
return count;
}
int main() {
char ch1 = 'e';
char ch2 = 'h';
string str1("hello there, how are you doing?");
string str2("Hello there! How are you doing?");
cout << "number of char - '" << ch1
<< "' occurrences = " << countOccurrences(ch1, str1) << endl;
cout << "number of char - '" << ch2
<< "' occurrences = " << countOccurrences(ch2, str2) << endl;
exit(EXIT_SUCCESS);
}
輸出:
number of char - 'e' occurrences = 4
number of char - 'h' occurrences = 1
使用 std::tolower
函式來計算字母大小寫的字元出現次數
實現上述示例的另一種方法是重寫 countOccurrences
函式,以在每次迭代時比較字元的轉換值。注意,std::tolower
很難使用,因為它要求唯一的 char
引數可以表示為 unsigned char
。否則,行為是不確定的。因此,我們將強制轉換的 unsigned char
值傳遞給 tolower
函式,然後將其強制轉換回 char
以儲存到臨時變數 tmp
中。另外,我們在每次迭代時都呼叫 tolower
函式,以將字串字元轉換為比較語句的小寫字母。請注意,以下程式碼示例可以區分大寫字母和小寫字母,並對它們全部進行計數。
#include <iostream>
#include <string>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::string;
size_t countOccurrences(char c, string &str) {
size_t count = 0;
char tmp = static_cast<char>(tolower(static_cast<unsigned char>(c)));
for (char i : str)
if (tolower(static_cast<unsigned char>(i)) == tmp) count++;
return count;
}
int main() {
char ch1 = 'e';
char ch2 = 'h';
string str1("hello there, how are you doing?");
string str2("Hello there! How are you doing?");
cout << "number of char - '" << ch1
<< "' occurrences = " << countOccurrences(ch1, str1) << endl;
cout << "number of char - '" << ch2
<< "' occurrences = " << countOccurrences(ch2, str2) << endl;
exit(EXIT_SUCCESS);
}
輸出:
number of char - 'e' occurrences = 4
number of char - 'h' occurrences = 3
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Jinku Hu